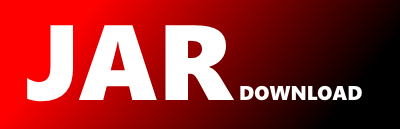
io.lettuce.core.output.DefaultTransactionResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of lettuce-core Show documentation
Show all versions of lettuce-core Show documentation
Advanced and thread-safe Java Redis client for synchronous, asynchronous, and
reactive usage. Supports Cluster, Sentinel, Pipelining, Auto-Reconnect, Codecs
and much more.
The newest version!
package io.lettuce.core.output;
import java.util.Iterator;
import java.util.List;
import java.util.stream.Stream;
import io.lettuce.core.TransactionResult;
import io.lettuce.core.internal.LettuceAssert;
/**
* Result of a {@code MULTI} transaction.
*
* @author Mark Paluch
* @since 5.0
*/
class DefaultTransactionResult implements Iterable
© 2015 - 2025 Weber Informatics LLC | Privacy Policy