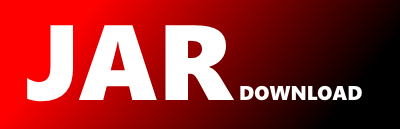
io.lettuce.core.output.PushOutput Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of lettuce-core Show documentation
Show all versions of lettuce-core Show documentation
Advanced and thread-safe Java Redis client for synchronous, asynchronous, and
reactive usage. Supports Cluster, Sentinel, Pipelining, Auto-Reconnect, Codecs
and much more.
The newest version!
package io.lettuce.core.output;
import java.nio.ByteBuffer;
import java.util.*;
import java.util.function.Function;
import io.lettuce.core.api.push.PushMessage;
import io.lettuce.core.codec.RedisCodec;
import io.lettuce.core.codec.StringCodec;
import io.lettuce.core.internal.LettuceAssert;
/**
* Output for push notifications. The response output is always {@code List<Object>} as push notifications may contain
* arbitrary values. The first response element which denotes the push message type is available through {@link #type()}.
*
* @author Mark Paluch
* @since 6.0
*/
public class PushOutput extends NestedMultiOutput implements PushMessage {
private String type;
public PushOutput(RedisCodec codec) {
super(codec);
}
@Override
public void set(ByteBuffer bytes) {
if (type == null) {
bytes.mark();
type = StringCodec.ASCII.decodeKey(bytes);
bytes.reset();
}
super.set(bytes);
}
@Override
public void setSingle(ByteBuffer bytes) {
if (type == null) {
set(bytes);
}
super.setSingle(bytes);
}
public String type() {
return type;
}
@Override
public String getType() {
return type();
}
@Override
public List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy