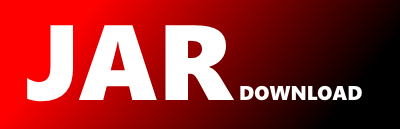
io.lettuce.core.protocol.RedisCommand Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of lettuce-core Show documentation
Show all versions of lettuce-core Show documentation
Advanced and thread-safe Java Redis client for synchronous, asynchronous, and
reactive usage. Supports Cluster, Sentinel, Pipelining, Auto-Reconnect, Codecs
and much more.
The newest version!
package io.lettuce.core.protocol;
import io.lettuce.core.output.CommandOutput;
import io.netty.buffer.ByteBuf;
/**
* A redis command that holds an output, arguments and a state, whether it is completed or not.
*
* Commands can be wrapped. Outer commands have to notify inner commands but inner commands do not communicate with outer
* commands.
*
* @author Mark Paluch
* @param Key type.
* @param Value type.
* @param Output type.
* @since 3.0
*/
public interface RedisCommand {
/**
* The command output. Can be null.
*
* @return the command output.
*/
CommandOutput getOutput();
/**
* Complete a command.
*/
void complete();
/**
* Complete this command by attaching the given {@link Throwable exception}.
*
* @param throwable the exception
* @return {@code true} if this invocation caused this CompletableFuture to transition to a completed state, else
* {@code false}
*/
boolean completeExceptionally(Throwable throwable);
/**
* Attempts to cancel execution of this command. This attempt will fail if the task has already completed, has already been
* cancelled, or could not be cancelled for some other reason.
*/
void cancel();
/**
* @return the current command args.
*/
CommandArgs getArgs();
/**
*
* @return the Redis command type like {@code SADD}, {@code HMSET}, {@code QUIT}.
*/
ProtocolKeyword getType();
/**
* Encode the command.
*
* @param buf byte buffer to operate on.
*/
void encode(ByteBuf buf);
/**
* Returns {@code true} if this task was cancelled before it completed normally.
*
* @return {@code true} if the command was cancelled before it completed normally.
*/
boolean isCancelled();
/**
* Returns {@code true} if this task completed.
*
* Completion may be due to normal termination, an exception, or cancellation. In all of these cases, this method will
* return {@code true}.
*
* @return {@code true} if this task completed.
*/
boolean isDone();
/**
* Set a new output. Only possible as long as the command is not completed/cancelled.
*
* @param output the new command output
* @throws IllegalStateException if the command is cancelled/completed
*/
void setOutput(CommandOutput output);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy