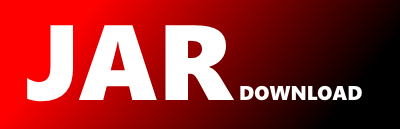
io.lettuce.core.resource.EventLoopGroupProvider Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of lettuce-core Show documentation
Show all versions of lettuce-core Show documentation
Advanced and thread-safe Java Redis client for synchronous, asynchronous, and
reactive usage. Supports Cluster, Sentinel, Pipelining, Auto-Reconnect, Codecs
and much more.
The newest version!
package io.lettuce.core.resource;
import java.util.concurrent.TimeUnit;
import io.netty.channel.EventLoopGroup;
import io.netty.util.concurrent.EventExecutorGroup;
import io.netty.util.concurrent.Future;
/**
* Provider for {@link EventLoopGroup EventLoopGroups and EventExecutorGroups}. A event loop group is a heavy-weight instance
* holding and providing {@link Thread} instances. Multiple instances can be created but are expensive. Keeping too many
* instances open can exhaust the number of open files.
*
* Usually, the default settings are sufficient. However, customizing might be useful for some special cases where multiple
* {@link io.lettuce.core.RedisClient} or {@link io.lettuce.core.cluster.RedisClusterClient} instances are needed that share one
* or more event loop groups.
*
*
* The {@link EventLoopGroupProvider} allows to allocate and release instances implementing {@link EventExecutorGroup}. The
* {@link EventExecutorGroup} instances must not be terminated or shutdown by the user. Resources are managed by the particular
* {@link EventLoopGroupProvider}.
*
* You can implement your own {@link EventLoopGroupProvider} to share existing {@link EventLoopGroup EventLoopGroup's} with
* lettuce.
*
* @author Mark Paluch
* @since 3.4
*/
public interface EventLoopGroupProvider {
/**
* Retrieve a {@link EventLoopGroup} for the {@link EventLoopGroup channel} {@code type}. Do not terminate or shutdown the
* instance. Call the {@link #release(EventExecutorGroup, long, long, TimeUnit)} to release an individual instance or
* {@link #shutdown(long, long, TimeUnit)} method to free the all resources.
*
* @param type class of the {@link EventLoopGroup}, must not be {@code null}
* @param type of the {@link EventLoopGroup}
* @return the {@link EventLoopGroup}.
*/
T allocate(Class type);
/**
* Returns the pool size (number of threads) for IO threads. The indicated size does not reflect the number for all IO
* threads, it is the number of threads that are used to create a particular thread pool.
*
* @return the pool size (number of threads) for all IO tasks.
*/
int threadPoolSize();
/**
* Release a {@code eventLoopGroup} instance. The method will shutdown/terminate the {@link EventExecutorGroup} if it is no
* longer needed.
*
* @param eventLoopGroup the eventLoopGroup instance, must not be {@code null}
* @param quietPeriod the quiet period
* @param timeout the timeout
* @param unit time unit for the quiet period/the timeout
* @return a close future to synchronize the called for shutting down.
*/
Future release(EventExecutorGroup eventLoopGroup, long quietPeriod, long timeout, TimeUnit unit);
/**
* Shutdown the provider and release all instances.
*
* @param quietPeriod the quiet period
* @param timeout the timeout
* @param timeUnit the unit of {@code quietPeriod} and {@code timeout}
* @return a close future to synchronize the called for shutting down.
*/
Future shutdown(long quietPeriod, long timeout, TimeUnit timeUnit);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy