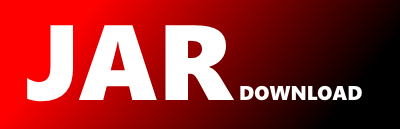
io.lettuce.core.sentinel.RedisSentinelReactiveCommandsImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of lettuce-core Show documentation
Show all versions of lettuce-core Show documentation
Advanced and thread-safe Java Redis client for synchronous, asynchronous, and
reactive usage. Supports Cluster, Sentinel, Pipelining, Auto-Reconnect, Codecs
and much more.
The newest version!
/*
* Copyright 2011-Present, Redis Ltd. and Contributors
* All rights reserved.
*
* Licensed under the MIT License.
*
* This file contains contributions from third-party contributors
* licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package io.lettuce.core.sentinel;
import java.net.SocketAddress;
import java.util.Map;
import io.lettuce.core.AbstractRedisReactiveCommands;
import io.lettuce.core.ClientListArgs;
import io.lettuce.core.KillArgs;
import io.lettuce.core.api.StatefulConnection;
import io.lettuce.core.codec.RedisCodec;
import io.lettuce.core.internal.LettuceAssert;
import io.lettuce.core.json.JsonParser;
import io.lettuce.core.output.CommandOutput;
import io.lettuce.core.protocol.Command;
import io.lettuce.core.protocol.CommandArgs;
import io.lettuce.core.protocol.ProtocolKeyword;
import io.lettuce.core.sentinel.api.StatefulRedisSentinelConnection;
import io.lettuce.core.sentinel.api.reactive.RedisSentinelReactiveCommands;
import reactor.core.publisher.Flux;
import reactor.core.publisher.Mono;
/**
* A reactive and thread-safe API for a Redis Sentinel connection.
*
* @param Key type.
* @param Value type.
* @author Mark Paluch
* @since 3.0
*/
public class RedisSentinelReactiveCommandsImpl extends AbstractRedisReactiveCommands
implements RedisSentinelReactiveCommands {
private final SentinelCommandBuilder commandBuilder;
public RedisSentinelReactiveCommandsImpl(StatefulConnection connection, RedisCodec codec,
Mono parser) {
super(connection, codec, parser);
commandBuilder = new SentinelCommandBuilder(codec);
}
@Override
public Mono getMasterAddrByName(K key) {
return createMono(() -> commandBuilder.getMasterAddrByKey(key));
}
@Override
public Flux
© 2015 - 2025 Weber Informatics LLC | Privacy Policy