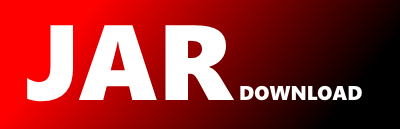
io.lettuce.core.sentinel.StatefulRedisSentinelConnectionImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of lettuce-core Show documentation
Show all versions of lettuce-core Show documentation
Advanced and thread-safe Java Redis client for synchronous, asynchronous, and
reactive usage. Supports Cluster, Sentinel, Pipelining, Auto-Reconnect, Codecs
and much more.
The newest version!
/*
* Copyright 2011-Present, Redis Ltd. and Contributors
* All rights reserved.
*
* Licensed under the MIT License.
*
* This file contains contributions from third-party contributors
* licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package io.lettuce.core.sentinel;
import java.time.Duration;
import java.util.Collection;
import io.lettuce.core.ConnectionState;
import io.lettuce.core.RedisChannelHandler;
import io.lettuce.core.RedisChannelWriter;
import io.lettuce.core.codec.RedisCodec;
import io.lettuce.core.codec.StringCodec;
import io.lettuce.core.json.JsonParser;
import io.lettuce.core.output.StatusOutput;
import io.lettuce.core.protocol.*;
import io.lettuce.core.sentinel.api.StatefulRedisSentinelConnection;
import io.lettuce.core.sentinel.api.async.RedisSentinelAsyncCommands;
import io.lettuce.core.sentinel.api.reactive.RedisSentinelReactiveCommands;
import io.lettuce.core.sentinel.api.sync.RedisSentinelCommands;
import reactor.core.publisher.Mono;
import static io.lettuce.core.ClientOptions.DEFAULT_JSON_PARSER;
/**
* @author Mark Paluch
*/
public class StatefulRedisSentinelConnectionImpl extends RedisChannelHandler
implements StatefulRedisSentinelConnection {
protected final RedisCodec codec;
protected final RedisSentinelCommands sync;
protected final RedisSentinelAsyncCommands async;
protected final RedisSentinelReactiveCommands reactive;
private final SentinelConnectionState connectionState = new SentinelConnectionState();
/**
* Initialize a new Sentinel connection
*
* @param writer the writer used to write commands
* @param codec Codec used to encode/decode keys and values.
* @param timeout Maximum time to wait for a response.
*/
public StatefulRedisSentinelConnectionImpl(RedisChannelWriter writer, RedisCodec codec, Duration timeout) {
this(writer, codec, timeout, DEFAULT_JSON_PARSER);
}
/**
* Initialize a new Sentinel connection
*
* @param writer the writer used to write commands
* @param codec Codec used to encode/decode keys and values.
* @param timeout Maximum time to wait for a response.
* @param parser the parser used to parse JSON responses
*/
public StatefulRedisSentinelConnectionImpl(RedisChannelWriter writer, RedisCodec codec, Duration timeout,
Mono parser) {
super(writer, timeout);
this.codec = codec;
this.async = new RedisSentinelAsyncCommandsImpl<>(this, codec);
this.sync = syncHandler(async, RedisSentinelCommands.class);
this.reactive = new RedisSentinelReactiveCommandsImpl<>(this, codec, parser);
}
@Override
public RedisCommand dispatch(RedisCommand command) {
return super.dispatch(command);
}
@Override
public Collection> dispatch(Collection extends RedisCommand> commands) {
return super.dispatch(commands);
}
@Override
public RedisSentinelCommands sync() {
return sync;
}
@Override
public RedisSentinelAsyncCommands async() {
return async;
}
@Override
public RedisSentinelReactiveCommands reactive() {
return reactive;
}
/**
* @param clientName
* @deprecated since 6.0, use {@link RedisSentinelAsyncCommands#clientSetname(Object)}.
*/
@Deprecated
public void setClientName(String clientName) {
CommandArgs args = new CommandArgs<>(StringCodec.UTF8).add(CommandKeyword.SETNAME).addValue(clientName);
AsyncCommand async = new AsyncCommand<>(
new Command<>(CommandType.CLIENT, new StatusOutput<>(StringCodec.UTF8), args));
connectionState.setClientName(clientName);
dispatch((RedisCommand) async);
}
public ConnectionState getConnectionState() {
return connectionState;
}
static class SentinelConnectionState extends ConnectionState {
@Override
protected void setClientName(String clientName) {
super.setClientName(clientName);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy