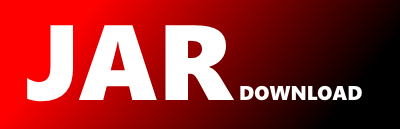
io.liftwizard.reladomo.simseq.ObjectSequenceData Maven / Gradle / Ivy
The newest version!
package io.liftwizard.reladomo.simseq;
import java.util.*;
import java.sql.Timestamp;
import java.sql.Date;
import java.math.BigDecimal;
import java.sql.Timestamp;
import java.util.*;
import java.io.*;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import com.gs.fw.common.mithra.*;
import com.gs.fw.common.mithra.attribute.*;
import com.gs.fw.common.mithra.util.*;
import com.gs.fw.common.mithra.notification.*;
import com.gs.fw.common.mithra.notification.listener.*;
import com.gs.fw.common.mithra.list.cursor.Cursor;
import com.gs.fw.common.mithra.bulkloader.*;
import com.gs.fw.common.mithra.finder.PrintablePreparedStatement;
import com.gs.fw.common.mithra.finder.RelatedFinder;
import com.gs.fw.common.mithra.cache.offheap.MithraOffHeapDataObject;
import com.gs.fw.common.mithra.cache.offheap.OffHeapDataStorage;
/**
* This file was automatically generated using Mithra 18.0.0. Please do not modify it.
* Add custom logic to its subclass instead.
*/
public class ObjectSequenceData
implements MithraDataObject
{
private byte isNullBits0 = 0;
private long nextId;
private String sequenceName;
public boolean isSequenceNameNull()
{
return this.getSequenceName() == null;
}
public boolean isNextIdNull()
{
return (isNullBits0 & 1) != 0 ;
}
public void zSerializeFullData(ObjectOutput out) throws IOException
{
zWriteNullBits(out);
out.writeLong(this.nextId);
out.writeObject(this.sequenceName);
}
private void zWriteNullBits(ObjectOutput out) throws IOException
{
out.writeByte(this.isNullBits0);
}
public long getNextId()
{
return this.nextId;
}
public void setNextId(long value)
{
this.nextId = value;
isNullBits0 = (byte)((int)isNullBits0 & ~( 1));
}
public String getSequenceName()
{
return this.sequenceName;
}
public int zGetSequenceNameAsInt()
{
return StringPool.getInstance().getOffHeapAddressWithoutAdding(sequenceName);
}
public void setSequenceName(String value)
{
this.sequenceName = StringPool.getInstance().getOrAddToCache(value, ObjectSequenceFinder.isFullCache());
}
public void setSequenceNameNull()
{
this.setSequenceName(null);
}
public void setNextIdNull()
{
isNullBits0 = (byte)((int)isNullBits0 | 1);
}
public byte zGetIsNullBits0()
{
return this.isNullBits0;
}
public void zSetIsNullBits0(byte newValue)
{
this.isNullBits0 = newValue;
}
protected void copyInto(ObjectSequenceData copy, boolean withRelationships)
{
copy.isNullBits0 = this.isNullBits0;
copy.nextId = this.nextId;
copy.sequenceName = this.sequenceName;
if (withRelationships)
{
}
}
public void zDeserializeFullData(ObjectInput in) throws IOException, ClassNotFoundException
{
this.isNullBits0 = in.readByte();
this.nextId = in.readLong();
this.sequenceName = StringPool.getInstance().getOrAddToCache((String)in.readObject(), ObjectSequenceFinder.isFullCache());
}
public boolean hasSamePrimaryKeyIgnoringAsOfAttributes(MithraDataObject other)
{
if (this == other) return true;
final ObjectSequenceData otherData = (ObjectSequenceData) other;
if (!isSequenceNameNull() ? !getSequenceName().equals(otherData.getSequenceName()) : !otherData.isSequenceNameNull())
{
return false;
}
return true;
}
public void zSerializePrimaryKey(ObjectOutput out) throws IOException
{
out.writeObject(this.sequenceName);
}
public void zDeserializePrimaryKey(ObjectInput in) throws IOException, ClassNotFoundException
{
this.sequenceName = StringPool.getInstance().getOrAddToCache((String)in.readObject(), ObjectSequenceFinder.isFullCache());
}
public void clearRelationships()
{
clearAllDirectRefs();
}
public void clearAllDirectRefs()
{
}
public void zSerializeRelationships(ObjectOutputStream out) throws IOException
{
}
public void zDeserializeRelationships(ObjectInputStream in) throws IOException, ClassNotFoundException
{
}
public MithraOffHeapDataObject zCopyOffHeap()
{
throw new RuntimeException("off heap no supported");
}
public void copyNonPkAttributes(MithraDataObject newData)
{
final ObjectSequenceData objectSequenceData = (ObjectSequenceData) newData;
this.setNextId(objectSequenceData.getNextId());
if (objectSequenceData.isNextIdNull()) this.setNextIdNull();
}
public byte zGetDataVersion()
{
return (byte)0;
}
public void zSetDataVersion(byte version)
{
}
// only used by dated objects
public void zIncrementDataVersion()
{
}
public boolean zNonPrimaryKeyAttributesChanged(MithraDataObject newData)
{
return this.zNonPrimaryKeyAttributesChanged(newData, 0.0);
}
public boolean zNonPrimaryKeyAttributesChanged(MithraDataObject newData, double toleranceForFloatingPointFields)
{
final ObjectSequenceData other = (ObjectSequenceData) newData;
if ( isNextIdNull() != other.isNextIdNull() || getNextId() != other.getNextId())
{
return true;
}
return false;
}
public MithraDataObject copy()
{
ObjectSequenceData copy = new ObjectSequenceData();
this.copyInto(copy, true);
return copy;
}
public MithraDataObject copy(boolean withRelationships)
{
ObjectSequenceData copy = new ObjectSequenceData();
this.copyInto(copy, withRelationships);
return copy;
}
public String zGetPrintablePrimaryKey()
{
String result = "";
result += "sequenceName: "+"'"+getSequenceName()+"'"+ " / ";
return result;
}
public boolean zAsOfAttributesFromEquals(MithraDataObject other)
{
return true;
}
public boolean zAsOfAttributesChanged(MithraDataObject other)
{
return false;
}
public void zWriteDataClassName(ObjectOutput out) throws IOException
{
}
public String zReadDataClassName(ObjectInput in) throws IOException, ClassNotFoundException
{
return "io.liftwizard.reladomo.simseq.ObjectSequenceData";
}
public boolean changed(MithraDataObject newData)
{
if(zNonPrimaryKeyAttributesChanged(newData))
{
return true;
}
else
{
return zAsOfAttributesChanged(newData);
}
}
public boolean zHasSameNullPrimaryKeyAttributes(MithraDataObject newData)
{
return true;
}
public MithraObjectPortal zGetMithraObjectPortal(int hierarchyDepth)
{
return ObjectSequenceFinder.getMithraObjectPortal();
}
public MithraObjectPortal zGetMithraObjectPortal()
{
return ObjectSequenceFinder.getMithraObjectPortal();
}
public Number zGetIdentityValue()
{
return null;
}
public boolean zHasIdentity()
{
return false;
}
public void zSetIdentity(Number identityValue)
{
}
public String zGetSerializationClassName()
{
return "io.liftwizard.reladomo.simseq.ObjectSequenceData";
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy