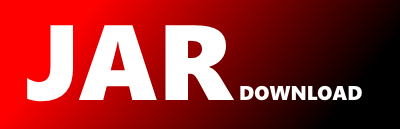
io.lightlink.config.ConfigManager Maven / Gradle / Ivy
package io.lightlink.config;
/*
* #%L
* lightlink-core
* %%
* Copyright (C) 2015 Vitaliy Shevchuk
* %%
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Lesser Public License for more details.
*
* You should have received a copy of the GNU General Lesser Public
* License along with this program. If not, see
* .
* #L%
*/
import io.lightlink.translator.ScriptTranslator;
import io.lightlink.utils.Utils;
import javax.script.ScriptEngine;
import javax.script.ScriptEngineManager;
import javax.servlet.ServletContext;
import java.io.IOException;
import java.io.InputStream;
import java.net.URL;
import java.util.ArrayList;
import java.util.List;
public class ConfigManager {
public static final String DEFAULT_ROOT_PACKAGE = "lightlink";
private ServletContext servletContext;
private String rootPackage;
private ScriptTranslator translator = new ScriptTranslator();
public ConfigManager() {
this(DEFAULT_ROOT_PACKAGE);
}
public ConfigManager(String rootPackage) {
this.rootPackage = rootPackage.replace('.', '/');
}
public ConfigManager(String rootPackage, ServletContext servletContext) {
this(rootPackage);
this.servletContext = servletContext;
}
public ConfigManager(ServletContext servletContext) {
this();
this.servletContext = servletContext;
}
public static ScriptEngine getScriptEngine() {
ScriptEngineManager manager = new ScriptEngineManager();
ScriptEngine engine = manager.getEngineByName("nashorn");
if (engine==null){
throw new IllegalStateException("Nashorn engine is not found. Please make sure you use Java 8 or higher OR " +
"to run on Java7 you need to add Nashorn backport jar to your webapp");
}
return engine;
}
public String getRootPackage() {
return rootPackage;
}
public static boolean isInDebugMode() {
return "true".equalsIgnoreCase(System.getProperty("lightlink.debug"));
}
public List