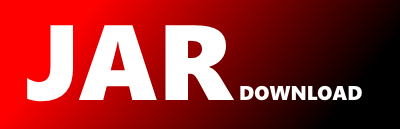
io.linguarobot.aws.cdk.maven.ImageBuild Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws-cdk-maven-plugin Show documentation
Show all versions of aws-cdk-maven-plugin Show documentation
The AWS CDK Maven plugin produces and deploys CloudFormation templates based on the cloud infrastructure defined
by means of CDK. The goal of the project is to improve the experience of Java developers while working with
CDK by eliminating the need for installing Node.js and interacting with the CDK application by means of CDK
Toolkit.
package io.linguarobot.aws.cdk.maven;
import com.google.common.collect.ImmutableMap;
import org.jetbrains.annotations.NotNull;
import javax.annotation.Nonnull;
import javax.annotation.Nullable;
import java.nio.file.Path;
import java.util.Map;
import java.util.Objects;
/**
* Represents Docker image build parameters.
*/
public class ImageBuild {
@Nonnull
private final Path contextDirectory;
@Nonnull
private final Path dockerfile;
@Nonnull
private final String imageTag;
@Nullable
private final String target;
@Nonnull
Map arguments;
private ImageBuild(@NotNull Path contextDirectory,
@NotNull Path dockerfile,
@NotNull String imageTag,
@Nullable String target,
@Nullable Map arguments) {
this.contextDirectory = Objects.requireNonNull(contextDirectory, "Docker context directory path can't be null");
this.dockerfile = Objects.requireNonNull(dockerfile, "Docker Dockerfile path can't be null");
this.imageTag = Objects.requireNonNull(imageTag, "Image tag can't be null");
this.target = target;
this.arguments = arguments != null ? ImmutableMap.copyOf(arguments) : ImmutableMap.of();
}
@Nonnull
public Path getContextDirectory() {
return contextDirectory;
}
@Nonnull
public Path getDockerfile() {
return dockerfile;
}
@Nonnull
public String getImageTag() {
return imageTag;
}
@Nullable
public String getTarget() {
return target;
}
@Nonnull
public Map getArguments() {
return arguments;
}
@Override
public String toString() {
return "ImageBuild{" +
"contextDirectory=" + contextDirectory +
", dockerfile=" + dockerfile +
", imageTag='" + imageTag + '\'' +
", target='" + target + '\'' +
", arguments=" + arguments +
'}';
}
public static Builder builder() {
return new Builder();
}
public static final class Builder {
Map arguments;
private Path contextDirectory;
private Path dockerfile;
private String imageTag;
private String target;
private Builder() {
}
public Builder withContextDirectory(@Nonnull Path contextDirectory) {
this.contextDirectory = contextDirectory;
return this;
}
public Builder withDockerfile(@Nonnull Path dockerfile) {
this.dockerfile = dockerfile;
return this;
}
public Builder withImageTag(@Nonnull String imageTag) {
this.imageTag = imageTag;
return this;
}
public Builder withTarget(@Nullable String target) {
this.target = target;
return this;
}
public Builder withArguments(@Nullable Map arguments) {
this.arguments = arguments;
return this;
}
public ImageBuild build() {
return new ImageBuild(contextDirectory, dockerfile, imageTag, target, arguments);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy