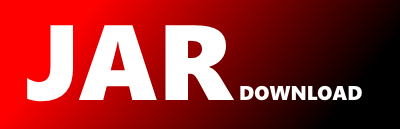
io.linguarobot.aws.cdk.maven.StackDefinition Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws-cdk-maven-plugin Show documentation
Show all versions of aws-cdk-maven-plugin Show documentation
The AWS CDK Maven plugin produces and deploys CloudFormation templates based on the cloud infrastructure defined
by means of CDK. The goal of the project is to improve the experience of Java developers while working with
CDK by eliminating the need for installing Node.js and interacting with the CDK application by means of CDK
Toolkit.
package io.linguarobot.aws.cdk.maven;
import com.google.common.collect.ImmutableList;
import com.google.common.collect.ImmutableMap;
import io.linguarobot.aws.cdk.AssetMetadata;
import javax.annotation.Nonnull;
import javax.annotation.Nullable;
import java.nio.file.Path;
import java.util.List;
import java.util.Map;
import java.util.Objects;
public class StackDefinition {
@Nonnull
private final String stackName;
@Nonnull
private final Path templateFile;
@Nonnull
private final String environment;
@Nullable
private final Integer requiredToolkitStackVersion;
@Nonnull
private final Map parameters;
@Nonnull
private final Map parameterValues;
@Nonnull
private final List assets;
@Nonnull
private final Map> resources;
private StackDefinition(@Nonnull String stackName,
@Nonnull Path templateFile,
@Nonnull String environment,
@Nullable Integer requiredToolkitStackVersion,
@Nullable Map parameters,
@Nullable Map parameterValues, List assets,
@Nullable Map> resources) {
this.stackName = Objects.requireNonNull(stackName, "Stack name can't be null");
this.templateFile = Objects.requireNonNull(templateFile, "Template file can't be null");
this.environment = Objects.requireNonNull(environment, "Environment can't be null");
this.requiredToolkitStackVersion = requiredToolkitStackVersion;
this.parameters = parameters != null ? ImmutableMap.copyOf(parameters) : ImmutableMap.of();
this.parameterValues = parameterValues != null ? ImmutableMap.copyOf(parameterValues) : ImmutableMap.of();
this.assets = assets != null ? ImmutableList.copyOf(assets) : ImmutableList.of();
this.resources = resources != null ? ImmutableMap.copyOf(resources) : ImmutableMap.of();
}
@Nonnull
public String getStackName() {
return stackName;
}
@Nonnull
public Path getTemplateFile() {
return templateFile;
}
@Nonnull
public String getEnvironment() {
return environment;
}
@Nullable
public Integer getRequiredToolkitStackVersion() {
return requiredToolkitStackVersion;
}
@Nonnull
public Map getParameters() {
return parameters;
}
@Nonnull
public Map getParameterValues() {
return parameterValues;
}
@Nonnull
public List getAssets() {
return assets;
}
@Nonnull
public Map> getResources() {
return resources;
}
@Override
public String toString() {
return "StackDefinition{" +
"stackName='" + stackName + '\'' +
", templateFile=" + templateFile +
", environment='" + environment + '\'' +
", requiredToolkitStackVersion=" + requiredToolkitStackVersion +
", parameters=" + parameters +
", parameterValues=" + parameterValues +
", assets=" + assets +
", resources=" + resources +
'}';
}
public static Builder builder() {
return new Builder();
}
public static final class Builder {
private String stackName;
private Path templateFile;
private String environment;
private Integer requiredToolkitStackVersion;
private Map parameters;
private Map parameterValues;
private List assets;
private Map> resources;
private Builder() {
}
public Builder withStackName(@Nonnull String stackName) {
this.stackName = stackName;
return this;
}
public Builder withTemplateFile(@Nonnull Path templateFile) {
this.templateFile = templateFile;
return this;
}
public Builder withEnvironment(@Nonnull String environment) {
this.environment = environment;
return this;
}
public Builder withRequiredToolkitStackVersion(@Nullable Integer requiredToolkitStackVersion) {
this.requiredToolkitStackVersion = requiredToolkitStackVersion;
return this;
}
public Builder withParameters(@Nullable Map parameters) {
this.parameters = parameters;
return this;
}
public Builder withParameterValues(@Nullable Map parameterValues) {
this.parameterValues = parameterValues;
return this;
}
public Builder withAssets(@Nullable List assets) {
this.assets = assets;
return this;
}
public Builder withResources(@Nullable Map> resources) {
this.resources = resources;
return this;
}
public StackDefinition build() {
return new StackDefinition(stackName, templateFile, environment, requiredToolkitStackVersion, parameters, parameterValues, assets, resources);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy