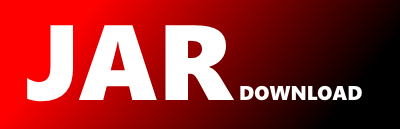
io.linguarobot.aws.cdk.maven.Stacks Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws-cdk-maven-plugin Show documentation
Show all versions of aws-cdk-maven-plugin Show documentation
The AWS CDK Maven plugin produces and deploys CloudFormation templates based on the cloud infrastructure defined
by means of CDK. The goal of the project is to improve the experience of Java developers while working with
CDK by eliminating the need for installing Node.js and interacting with the CDK application by means of CDK
Toolkit.
package io.linguarobot.aws.cdk.maven;
import software.amazon.awssdk.services.cloudformation.CloudFormationClient;
import software.amazon.awssdk.services.cloudformation.model.Stack;
import software.amazon.awssdk.services.cloudformation.model.*;
import javax.annotation.Nullable;
import java.time.Duration;
import java.util.*;
import java.util.concurrent.*;
import java.util.function.Consumer;
import java.util.function.Supplier;
import java.util.stream.Collectors;
import java.util.stream.Stream;
public class Stacks {
private static final ScheduledExecutorService SCHEDULER = new ScheduledThreadPoolExecutor(0);
private static final Capability[] CAPABILITIES =
new Capability[]{Capability.CAPABILITY_IAM, Capability.CAPABILITY_NAMED_IAM, Capability.CAPABILITY_AUTO_EXPAND};
public static Optional findStack(CloudFormationClient client, String stackName) {
Objects.requireNonNull(client, "CloudFormation client can't be null");
Objects.requireNonNull(stackName, "stack name can't be null");
try {
return Optional.of(getStack(client, stackName));
} catch (CloudFormationException e) {
// Assuming that the exception is thrown only if the stack doesn't exist
return Optional.empty();
}
}
public static Stack createStack(CloudFormationClient client, String stackName, TemplateRef template) {
return createStack(client, stackName, template, Collections.emptyMap());
}
public static Stack createStack(CloudFormationClient client,
String stackName,
TemplateRef template,
Map parameters) {
Objects.requireNonNull(client, "CloudFormation client can't be null");
Objects.requireNonNull(stackName, "stack name can't be null");
Objects.requireNonNull(template, "template reference can't be null");
CreateStackRequest request = CreateStackRequest.builder()
.stackName(stackName)
.templateBody(template.getBody())
.templateURL(template.getUrl())
.parameters(parameters != null ? buildParameters(parameters) : Collections.emptyList())
.capabilities(CAPABILITIES)
.build();
CreateStackResponse response = client.createStack(request);
return getStack(client, response.stackId());
}
public static Stack updateStack(CloudFormationClient client,
String stackName,
TemplateRef template,
Map parameters) {
Objects.requireNonNull(client, "CloudFormation client can't be null");
Objects.requireNonNull(stackName, "stack name can't be null");
Objects.requireNonNull(template, "template reference can't be null");
UpdateStackRequest request = UpdateStackRequest.builder()
.stackName(stackName)
.templateBody(template.getBody())
.templateURL(template.getUrl())
.parameters(parameters != null ? buildParameters(parameters) : Collections.emptyList())
.capabilities(CAPABILITIES)
.build();
UpdateStackResponse response = client.updateStack(request);
return getStack(client, response.stackId());
}
private static List buildParameters(Map parameters) {
return parameters.entrySet().stream()
.map(parameter -> Parameter.builder()
.parameterKey(parameter.getKey())
.parameterValue(parameter.getValue().get())
.usePreviousValue(!parameter.getValue().isUpdated())
.build())
.collect(Collectors.toList());
}
public static Stack deleteStack(CloudFormationClient client, String stackName) {
Objects.requireNonNull(client, "CloudFormation client can't be null");
Objects.requireNonNull(stackName, "stack name can't be null");
Stack stack = getStack(client, stackName);
DeleteStackRequest request = DeleteStackRequest.builder()
.stackName(stack.stackId())
.build();
client.deleteStack(request);
return getStack(client, stackName);
}
public static Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy