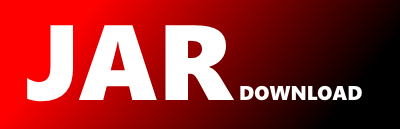
io.logicdrop.openapi.models.PropertyDefinition Maven / Gradle / Ivy
/*
* Sparks OpenAPI
* Generated documentation for the Logicdrop Sparks API and OpenAPI clients. Logicdrop Sparks lets users build rules, analyze data, and automate documents. Use it to make decisions faster, generate documents better, and learn from your data. ### Documentation - [User Documentation](https://docs.logicdrop.com) ### Modules - [Sparks Compute](https://docs.logicdrop.com/rules/introduction) - [Sparks Decision Tables](https://docs.logicdrop.com/rules/authoring-decision-tables) - [Sparks Documents](https://docs.logicdrop.com/documents/introduction) ### Clients - [OpenAPI Clients](https://docs.logicdrop.com/development/sample-clients) ### Security - [Authorizing API Requests](https://docs.logicdrop.com/development/authorization)
*
* The version of the OpenAPI document: v_VERSION_, build# _BUILD_
* Contact: [email protected]
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package io.logicdrop.openapi.models;
import java.util.Objects;
import java.util.Arrays;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonValue;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
/**
* PropertyDefinition
*/
@JsonPropertyOrder({
PropertyDefinition.JSON_PROPERTY_NAME,
PropertyDefinition.JSON_PROPERTY_TYPE,
PropertyDefinition.JSON_PROPERTY_VALUE,
PropertyDefinition.JSON_PROPERTY_KEY
})
public class PropertyDefinition {
public static final String JSON_PROPERTY_NAME = "name";
private String name;
/**
* Data type
*/
public enum TypeEnum {
STRING("String"),
INTEGER("Integer"),
DOUBLE("Double"),
FLOAT("Float"),
SET("Set"),
LIST("List"),
MAP("Map");
private String value;
TypeEnum(String value) {
this.value = value;
}
@JsonValue
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
@JsonCreator
public static TypeEnum fromValue(String value) {
for (TypeEnum b : TypeEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
throw new IllegalArgumentException("Unexpected value '" + value + "'");
}
}
public static final String JSON_PROPERTY_TYPE = "type";
private TypeEnum type;
public static final String JSON_PROPERTY_VALUE = "value";
private String value;
public static final String JSON_PROPERTY_KEY = "key";
private Boolean key;
public PropertyDefinition name(String name) {
this.name = name;
return this;
}
/**
* Name of the property
* @return name
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Name of the property")
@JsonProperty(JSON_PROPERTY_NAME)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public PropertyDefinition type(TypeEnum type) {
this.type = type;
return this;
}
/**
* Data type
* @return type
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Data type")
@JsonProperty(JSON_PROPERTY_TYPE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public TypeEnum getType() {
return type;
}
public void setType(TypeEnum type) {
this.type = type;
}
public PropertyDefinition value(String value) {
this.value = value;
return this;
}
/**
* Default value
* @return value
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Default value")
@JsonProperty(JSON_PROPERTY_VALUE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getValue() {
return value;
}
public void setValue(String value) {
this.value = value;
}
public PropertyDefinition key(Boolean key) {
this.key = key;
return this;
}
/**
* Is the property a key?
* @return key
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Is the property a key?")
@JsonProperty(JSON_PROPERTY_KEY)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Boolean getKey() {
return key;
}
public void setKey(Boolean key) {
this.key = key;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
PropertyDefinition propertyDefinition = (PropertyDefinition) o;
return Objects.equals(this.name, propertyDefinition.name) &&
Objects.equals(this.type, propertyDefinition.type) &&
Objects.equals(this.value, propertyDefinition.value) &&
Objects.equals(this.key, propertyDefinition.key);
}
@Override
public int hashCode() {
return Objects.hash(name, type, value, key);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class PropertyDefinition {\n");
sb.append(" name: ").append(toIndentedString(name)).append("\n");
sb.append(" type: ").append(toIndentedString(type)).append("\n");
sb.append(" value: ").append(toIndentedString(value)).append("\n");
sb.append(" key: ").append(toIndentedString(key)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy