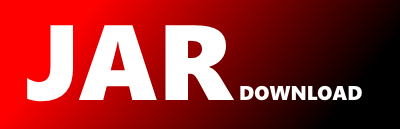
io.logz.sawmill.Doc Maven / Gradle / Ivy
The newest version!
package io.logz.sawmill;
import org.apache.commons.collections4.MapUtils;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import static com.google.common.base.Preconditions.checkState;
public class Doc {
private final Map source;
public Doc(Map source) {
checkState(MapUtils.isNotEmpty(source), "source cannot be empty");
this.source = source;
}
public Map getSource() { return source; }
public boolean hasField(String path) {
Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy