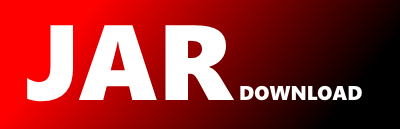
db.mapper.IssueMapper.xml Maven / Gradle / Ivy
<?xml version="1.0" encoding="UTF-8"?> <!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd"> <mapper namespace="io.lsn.spring.issue.domain.IssueDao"> <select id="getOneHeader" parameterType="map" resultType="String"> select row_to_json(t) from (select * from issue where id = #{id}) as t </select> <insert id="create" parameterType="map" useGeneratedKeys="true" keyProperty="issue.id,issue.uid" keyColumn="id,uid"> insert into issue (user_profile, author_id, number, subject, description, issue_date, planned_date, status_code, priority_code, reference_id, reference_type, type_code, external_user_id) values (#{user.username}, #{issue.author.id}, #{issue.number}, #{issue.subject}, #{issue.description}, #{issue.issueDate}, #{issue.plannedDate}, #{issue.statusCode}, #{issue.priorityCode}, #{issue.referenceId}, #{issue.referenceType}, #{issue.typeCode}, #{issue.externalUserId}); </insert> <insert id="addComment" parameterType="map" useGeneratedKeys="true" keyProperty="comment.id,comment.uid" keyColumn="id,uid"> insert into issue_comment (user_profile, issue_id, author_id, comment_date, body) values (#{user.username}, #{comment.issueId}, #{comment.author.id}, #{comment.commentDate}, #{comment.body}); </insert> <update id="removeComment" parameterType="map"> update issue_comment set deleted = true, user_profile = #{user.username} where id = #{comment.id}; </update> <insert id="addIssueFile" parameterType="map"> insert into issue_file (issue_id, file_hash) select #{issue.id}, #{hash} where not exists(select id from issue_file where issue_id = #{issue.id} and file_hash = #{hash} and comment_id is null and not deleted ); </insert> <update id="removeIssueFile" parameterType="map"> update issue_file set deleted = true, user_profile = #{user.username} where issue_id = #{issue.id} and file_hash = #{hash} and not deleted and comment_id is null; </update> <insert id="addIssueWatcher" parameterType="map"> insert into issue_watcher (user_profile, issue_id, user_id) select #{user.username}, #{issue.id}, #{userId} where not exists(select id from issue_watcher where issue_id = #{issue.id} and not deleted and user_id = #{userId}); </insert> <insert id="addCommentFile" parameterType="map"> insert into issue_file (user_profile, issue_id, comment_id, file_hash) select #{user.username}, #{comment.issueId}, #{comment.id}, #{hash} where not exists( select id from issue_file where issue_id = #{comment.issueId} and comment_id = #{comment.id} and file_hash = #{hash} and not deleted ); </insert> <insert id="addAssignee" parameterType="map"> insert into issue_assignee (user_profile, issue_id, user_id) select #{user.username}, #{issue.id}, #{assigneeId} where not exists(select 1 from issue_assignee where issue_id = #{issue.id} and not deleted and user_id = #{assigneeId}); </insert> <update id="removeAssignee" parameterType="map"> update issue_assignee set deleted = true where issue_id = #{issue.id} and user_id = #{assigneeId}; </update> <update id="removeCommentFile" parameterType="map"> update issue_file set deleted = true, user_profile = #{user.username} where issue_id = #{comment.issueId} and comment_id = #{comment.id} and not deleted; </update> <update id="removeIssueWatcher"> update issue_watcher set deleted = true where not deleted and issue_id = #{issue.id} and user_id = #{userId}; </update> <update id="updateIssue" parameterType="map"> update issue set user_profile = #{user.username}, status_code = #{issue.statusCode}, priority_code = #{issue.priorityCode}, number = #{issue.number}, subject = #{issue.subject}, planned_date = #{issue.plannedDate}, reference_id = #{issue.referenceId}, reference_type = #{issue.referenceType} where id = #{issue.id}; </update> <update id="updateStatus"> update issue set status_code = #{statusCode}, status_date = now() :: timestamp, user_profile = #{user.username} where id = #{issue.id} and status_code != #{statusCode}; </update> <update id="updateComment"> update issue_comment set body = #{comment.body} where id = #{comment.id}; </update> <update id="closeAllByReference" parameterType="map"> update issue set status_code = #{statusCode}, status_date = now() :: timestamp, user_profile = #{user.username} where reference_id is not null and reference_id = #{referenceId} and reference_type is not null and reference_type = #{referenceType} and status_code != #{statusCode}; </update> <update id="updateAttributeList"> delete from issue_attributes where issue_id = #{issue.id}; <foreach collection="issue.attributeList" item="a"> insert into issue_attributes (user_profile, issue_id, code, type, string_value, long_value, decimal_value, date_value, date_time_value) values (#{user.id}, #{issue.id}, #{a.code}, #{a.type.name}, #{a.stringValue}, #{a.longValue}, #{a.decimalValue}, #{a.dateValue}, #{a.dateTimeValue}); </foreach> </update> </mapper>
© 2015 - 2025 Weber Informatics LLC | Privacy Policy