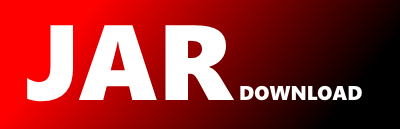
io.magentys.cucumber.java8.CherryOnTop Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cherry-cucumber Show documentation
Show all versions of cherry-cucumber Show documentation
Integrate Cucumber and Cherry :-)
The newest version!
package io.magentys.cucumber.java8;
import cucumber.api.java8.GlueBase;
import cucumber.runtime.java.JavaBackend;
import cucumber.runtime.java8.ConstantPoolTypeIntrospector;
import io.magentys.FunctionalAgent;
import io.magentys.functional.Functions;
public interface CherryOnTop extends GlueBase {
FunctionalAgent getFunctionalAgent();
default void CherryStep(final String regexp, final Functions.FunctionalMission body) {
CherryStepBody.Body0 a0Body = () -> body.apply(getFunctionalAgent());
JavaBackend.INSTANCE.get().addStepDefinition(regexp, 0, a0Body, CherryTypeInspector.INSTANCE);
}
default void CherryStep(final String regexp, final long timeout, final Functions.FunctionalMission body) {
CherryStepBody.Body0 a0Body = () -> body.apply(getFunctionalAgent());
JavaBackend.INSTANCE.get().addStepDefinition(regexp, timeout, a0Body, CherryTypeInspector.INSTANCE);
}
default void CherryStep(final String regexp, final Functions.FunctionalMission1 body) {
CherryStepBody.Body1 aBody = new CherryStepBody.Body1() {
@Override
public void accept(String p1) {
body.apply(p1, getFunctionalAgent());
}
};
JavaBackend.INSTANCE.get().addStepDefinition(regexp, 0, aBody, ConstantPoolTypeIntrospector.INSTANCE);
}
default void CherryStep(final String regexp, final long timeout, final Functions.FunctionalMission1 body) {
CherryStepBody.Body1 aBody = new CherryStepBody.Body1() {
@Override
public void accept(String p1) {
body.apply(p1, getFunctionalAgent());
}
};
JavaBackend.INSTANCE.get().addStepDefinition(regexp, timeout, aBody, ConstantPoolTypeIntrospector.INSTANCE);
}
default void CherryStep(final String regexp, final Functions.FunctionalMission2 body) {
CherryStepBody.Body2 aBody = new CherryStepBody.Body2() {
@Override
public void accept(String p1, String p2) {
body.apply(p1, p2, getFunctionalAgent());
}
};
JavaBackend.INSTANCE.get().addStepDefinition(regexp, 0, aBody, ConstantPoolTypeIntrospector.INSTANCE);
}
default void CherryStep(final String regexp, final long timeout, final Functions.FunctionalMission2 body) {
CherryStepBody.Body2 aBody = new CherryStepBody.Body2() {
@Override
public void accept(String p1, String p2) {
body.apply(p1, p2, getFunctionalAgent());
}
};
JavaBackend.INSTANCE.get().addStepDefinition(regexp, timeout, aBody, ConstantPoolTypeIntrospector.INSTANCE);
}
default void CherryStep(final String regexp, final Functions.FunctionalMission3 body) {
CherryStepBody.Body3 aBody = new CherryStepBody.Body3() {
@Override
public void accept(String p1, String p2, String p3) {
body.apply(p1, p2, p3, getFunctionalAgent());
}
};
JavaBackend.INSTANCE.get().addStepDefinition(regexp, 0, aBody, ConstantPoolTypeIntrospector.INSTANCE);
}
default void CherryStep(final String regexp, final long timeout, final Functions.FunctionalMission3 body) {
CherryStepBody.Body3 aBody = new CherryStepBody.Body3() {
@Override
public void accept(String p1, String p2, String p3) {
body.apply(p1, p2, p3, getFunctionalAgent());
}
};
JavaBackend.INSTANCE.get().addStepDefinition(regexp, timeout, aBody, ConstantPoolTypeIntrospector.INSTANCE);
}
default void CherryStep(final String regexp, final Functions.FunctionalMission4 body) {
CherryStepBody.Body4 aBody = new CherryStepBody.Body4() {
@Override
public void accept(String p1, String p2, String p3, String p4) {
body.apply(p1, p2, p3, p4, getFunctionalAgent());
}
};
JavaBackend.INSTANCE.get().addStepDefinition(regexp, 0, aBody, ConstantPoolTypeIntrospector.INSTANCE);
}
default void CherryStep(final String regexp, final long timeout, final Functions.FunctionalMission4 body) {
CherryStepBody.Body4 aBody = new CherryStepBody.Body4() {
@Override
public void accept(String p1, String p2, String p3, String p4) {
body.apply(p1, p2, p3, p4, getFunctionalAgent());
}
};
JavaBackend.INSTANCE.get().addStepDefinition(regexp, timeout, aBody, ConstantPoolTypeIntrospector.INSTANCE);
}
default void CherryStep(final String regexp, final Functions.FunctionalMission5 body) {
CherryStepBody.Body5 aBody = new CherryStepBody.Body5() {
@Override
public void accept(String p1, String p2, String p3, String p4, String p5) {
body.apply(p1, p2, p3, p4, p5, getFunctionalAgent());
}
};
JavaBackend.INSTANCE.get().addStepDefinition(regexp, 0, aBody, ConstantPoolTypeIntrospector.INSTANCE);
}
default void CherryStep(final String regexp, final long timeout, final Functions.FunctionalMission5 body) {
CherryStepBody.Body5 aBody = new CherryStepBody.Body5() {
@Override
public void accept(String p1, String p2, String p3, String p4, String p5) {
body.apply(p1, p2, p3, p4, p5, getFunctionalAgent());
}
};
JavaBackend.INSTANCE.get().addStepDefinition(regexp, timeout, aBody, ConstantPoolTypeIntrospector.INSTANCE);
}
default void CherryStep(final String regexp, final Functions.FunctionalMission6 body) {
CherryStepBody.Body6 aBody = new CherryStepBody.Body6() {
@Override
public void accept(String p1, String p2, String p3, String p4, String p5, String p6) {
body.apply(p1, p2, p3, p4, p5, p6, getFunctionalAgent());
}
};
JavaBackend.INSTANCE.get().addStepDefinition(regexp, 0, aBody, ConstantPoolTypeIntrospector.INSTANCE);
}
default void CherryStep(final String regexp, final long timeout, final Functions.FunctionalMission6 body) {
CherryStepBody.Body6 aBody = new CherryStepBody.Body6() {
@Override
public void accept(String p1, String p2, String p3, String p4, String p5, String p6) {
body.apply(p1, p2, p3, p4, p5, p6, getFunctionalAgent());
}
};
JavaBackend.INSTANCE.get().addStepDefinition(regexp, timeout, aBody, ConstantPoolTypeIntrospector.INSTANCE);
}
default void CherryStep(final String regexp, final Functions.FunctionalMission7 body) {
CherryStepBody.Body7 aBody = new CherryStepBody.Body7() {
@Override
public void accept(String p1, String p2, String p3, String p4, String p5, String p6, String p7) {
body.apply(p1, p2, p3, p4, p5, p6, p7, getFunctionalAgent());
}
};
JavaBackend.INSTANCE.get().addStepDefinition(regexp, 0, aBody, ConstantPoolTypeIntrospector.INSTANCE);
}
default void CherryStep(final String regexp, final long timeout, final Functions.FunctionalMission7 body) {
CherryStepBody.Body7 aBody = new CherryStepBody.Body7() {
@Override
public void accept(String p1, String p2, String p3, String p4, String p5, String p6, String p7) {
body.apply(p1, p2, p3, p4, p5, p6, p7, getFunctionalAgent());
}
};
JavaBackend.INSTANCE.get().addStepDefinition(regexp, timeout, aBody, ConstantPoolTypeIntrospector.INSTANCE);
}
default void CherryStep(final String regexp, final Functions.FunctionalMission8 body) {
CherryStepBody.Body8 aBody = new CherryStepBody.Body8() {
@Override
public void accept(String p1, String p2, String p3, String p4, String p5, String p6, String p7, String p8) {
body.apply(p1, p2, p3, p4, p5, p6, p7, p8, getFunctionalAgent());
}
};
JavaBackend.INSTANCE.get().addStepDefinition(regexp, 0, aBody, ConstantPoolTypeIntrospector.INSTANCE);
}
default void CherryStep(final String regexp, final long timeout, final Functions.FunctionalMission8 body) {
CherryStepBody.Body8 aBody = new CherryStepBody.Body8() {
@Override
public void accept(String p1, String p2, String p3, String p4, String p5, String p6, String p7, String p8) {
body.apply(p1, p2, p3, p4, p5, p6, p7, p8, getFunctionalAgent());
}
};
JavaBackend.INSTANCE.get().addStepDefinition(regexp, timeout, aBody, ConstantPoolTypeIntrospector.INSTANCE);
}
default void CherryStep(final String regexp, final Functions.FunctionalMission9 body) {
CherryStepBody.Body9 aBody = new CherryStepBody.Body9() {
@Override
public void accept(String p1, String p2, String p3, String p4, String p5, String p6, String p7, String p8, String p9) {
body.apply(p1, p2, p3, p4, p5, p6, p7, p8, p9, getFunctionalAgent());
}
};
JavaBackend.INSTANCE.get().addStepDefinition(regexp, 0, aBody, ConstantPoolTypeIntrospector.INSTANCE);
}
default void CherryStep(final String regexp, final long timeout, final Functions.FunctionalMission9 body) {
CherryStepBody.Body9 aBody = new CherryStepBody.Body9() {
@Override
public void accept(String p1, String p2, String p3, String p4, String p5, String p6, String p7, String p8, String p9) {
body.apply(p1, p2, p3, p4, p5, p6, p7, p8, p9, getFunctionalAgent());
}
};
JavaBackend.INSTANCE.get().addStepDefinition(regexp, timeout, aBody, ConstantPoolTypeIntrospector.INSTANCE);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy