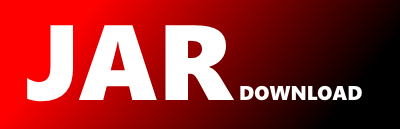
io.reactivex.mantis.remote.observable.ConnectToGroupedObservable Maven / Gradle / Ivy
/*
* Copyright 2019 Netflix, Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package io.reactivex.mantis.remote.observable;
import io.mantisrx.common.codec.Decoder;
import java.util.HashMap;
import java.util.Map;
import rx.functions.Action0;
import rx.functions.Action3;
import rx.subjects.PublishSubject;
public class ConnectToGroupedObservable extends ConnectToConfig {
private Decoder keyDecoder;
private Decoder valueDecoder;
private Action3 deocdingErrorHandler;
ConnectToGroupedObservable(Builder builder) {
super(builder.host, builder.port,
builder.name,
builder.subscribeParameters,
builder.subscribeAttempts,
builder.suppressDecodingErrors,
builder.connectionDisconnectCallback,
builder.closeTrigger);
this.keyDecoder = builder.keyDecoder;
this.valueDecoder = builder.valueDecoder;
this.deocdingErrorHandler = builder.deocdingErrorHandler;
}
public Decoder getKeyDecoder() {
return keyDecoder;
}
public Decoder getValueDecoder() {
return valueDecoder;
}
public Action3 getDeocdingErrorHandler() {
return deocdingErrorHandler;
}
public static class Builder {
private String host;
private int port;
private String name;
private Decoder keyDecoder;
private Decoder valueDecoder;
private Map subscribeParameters = new HashMap<>();
private int subscribeAttempts = 3;
private Action3 deocdingErrorHandler = new Action3() {
@Override
public void call(K key, V value, Throwable t2) {
t2.printStackTrace();
}
};
private boolean suppressDecodingErrors = false;
private Action0 connectionDisconnectCallback = new Action0() {
@Override
public void call() {}
};
private PublishSubject closeTrigger = PublishSubject.create();
public Builder() {}
public Builder(Builder config) {
this.host = config.host;
this.port = config.port;
this.name = config.name;
this.keyDecoder = config.keyDecoder;
this.valueDecoder = config.valueDecoder;
this.subscribeParameters.putAll(config.subscribeParameters);
this.subscribeAttempts = config.subscribeAttempts;
this.deocdingErrorHandler = config.deocdingErrorHandler;
this.suppressDecodingErrors = config.suppressDecodingErrors;
}
public Builder host(String host) {
this.host = host;
return this;
}
public Builder port(int port) {
this.port = port;
return this;
}
public Builder closeTrigger(PublishSubject closeTrigger) {
this.closeTrigger = closeTrigger;
return this;
}
public Builder connectionDisconnectCallback(Action0 connectionDisconnectCallback) {
this.connectionDisconnectCallback = connectionDisconnectCallback;
return this;
}
public Builder deocdingErrorHandler(Action3 handler, boolean suppressDecodingErrors) {
this.deocdingErrorHandler = handler;
this.suppressDecodingErrors = suppressDecodingErrors;
return this;
}
public Builder name(String name) {
this.name = name;
this.subscribeParameters.put("groupId", name);//used with modern server for routing
return this;
}
public Builder slotId(String slotId) {
this.subscribeParameters.put("slotId", slotId);
return this;
}
public Builder keyDecoder(Decoder keyDecoder) {
this.keyDecoder = keyDecoder;
return this;
}
public Builder valueDecoder(Decoder valueDecoder) {
this.valueDecoder = valueDecoder;
return this;
}
public Builder subscribeParameters(Map subscribeParameters) {
this.subscribeParameters.putAll(subscribeParameters);
return this;
}
public Builder subscribeAttempts(int subscribeAttempts) {
this.subscribeAttempts = subscribeAttempts;
return this;
}
public ConnectToGroupedObservable build() {
return new ConnectToGroupedObservable(this);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy