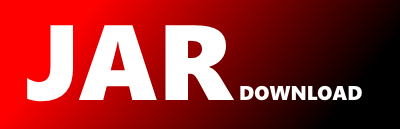
io.reactivex.mantis.remote.observable.DynamicConnection Maven / Gradle / Ivy
/*
* Copyright 2019 Netflix, Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package io.reactivex.mantis.remote.observable;
import io.mantisrx.common.network.Endpoint;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import rx.Observable;
import rx.Observable.OnSubscribe;
import rx.Observer;
import rx.Subscriber;
import rx.functions.Action1;
import rx.functions.Func1;
import rx.observables.GroupedObservable;
import rx.subjects.PublishSubject;
public class DynamicConnection {
private static final Logger logger = LoggerFactory.getLogger(DynamicConnection.class);
private Observable changeEndpointObservable;
private PublishSubject> subject = PublishSubject.create();
private Func1> toObservableFunc;
DynamicConnection(Func1> toObservableFunc, Observable changeEndpointObservable) {
this.changeEndpointObservable = changeEndpointObservable;
this.toObservableFunc = toObservableFunc;
}
public static DynamicConnection> create(
final ConnectToGroupedObservable.Builder config, Observable endpoints) {
Func1>> toObservableFunc = new
Func1>>() {
@Override
public Observable> call(Endpoint endpoint) {
// copy config, change host, port and id
ConnectToGroupedObservable.Builder configCopy = new ConnectToGroupedObservable.Builder(config);
configCopy
.host(endpoint.getHost())
.port(endpoint.getPort())
.slotId(endpoint.getSlotId());
return RemoteObservable.connect(configCopy.build()).getObservable();
}
};
return new DynamicConnection>(toObservableFunc, endpoints);
}
public static DynamicConnection create(
final ConnectToObservable.Builder config, Observable endpoints) {
Func1> toObservableFunc = new
Func1>() {
@Override
public Observable call(Endpoint endpoint) {
// copy config, change host, port and id
ConnectToObservable.Builder configCopy = new ConnectToObservable.Builder(config);
configCopy
.host(endpoint.getHost())
.port(endpoint.getPort())
.slotId(endpoint.getSlotId());
return RemoteObservable.connect(configCopy.build()).getObservable();
}
};
return new DynamicConnection(toObservableFunc, endpoints);
}
public void close() {
subject.onCompleted();
}
public Observable observable() {
return Observable.create(new OnSubscribe() {
@Override
public void call(final Subscriber super T> subscriber) {
subscriber.add(subject.flatMap(new Func1, Observable>() {
@Override
public Observable call(Observable t1) {
return t1;
}
}).subscribe(new Observer() {
@Override
public void onCompleted() {
subscriber.onCompleted();
}
@Override
public void onError(Throwable e) {
subscriber.onError(e);
}
@Override
public void onNext(T t) {
subscriber.onNext(t);
}
}));
subscriber.add(changeEndpointObservable.subscribe(new Action1() {
@Override
public void call(Endpoint endpoint) {
logger.debug("New endpoint: " + endpoint);
subject.onNext(toObservableFunc.call(endpoint));
}
}));
}
});
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy