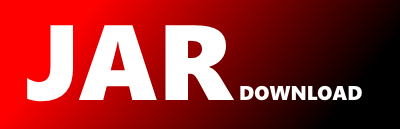
io.reactivex.mantis.remote.observable.FixedConnectionSet Maven / Gradle / Ivy
/*
* Copyright 2019 Netflix, Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package io.reactivex.mantis.remote.observable;
import io.mantisrx.common.network.Endpoint;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import rx.Observable;
import rx.Observable.OnSubscribe;
import rx.Observer;
import rx.Subscriber;
import rx.Subscription;
import rx.functions.Action1;
import rx.functions.Func1;
import rx.observables.GroupedObservable;
import rx.subscriptions.BooleanSubscription;
public class FixedConnectionSet {
private static final Logger logger = LoggerFactory.getLogger(FixedConnectionSet.class);
private EndpointInjector endpointInjector;
private Func1> toObservableFunc;
private MergedObservable mergedObservable;
public FixedConnectionSet(int expectedTerminalCount, EndpointInjector endpointInjector,
Func1> toObservableFunc) {
this.endpointInjector = endpointInjector;
this.toObservableFunc = toObservableFunc;
this.mergedObservable = MergedObservable.create(expectedTerminalCount);
}
public static FixedConnectionSet> create(int expectedTerminalCount,
final ConnectToGroupedObservable.Builder config, EndpointInjector endpointService) {
Func1>> toObservableFunc = new
Func1>>() {
@Override
public Observable> call(Endpoint endpoint) {
config.host(endpoint.getHost())
.port(endpoint.getPort());
return RemoteObservable.connect(config.build()).getObservable();
}
};
return new FixedConnectionSet>(expectedTerminalCount, endpointService, toObservableFunc);
}
public static FixedConnectionSet create(int expectedTerminalCount,
final ConnectToObservable.Builder config, EndpointInjector endpointService) {
Func1> toObservableFunc = new
Func1>() {
@Override
public Observable call(Endpoint endpoint) {
config.host(endpoint.getHost())
.port(endpoint.getPort());
return RemoteObservable.connect(config.build()).getObservable();
}
};
return new FixedConnectionSet(expectedTerminalCount, endpointService, toObservableFunc);
}
public Observable> getObservables() {
return Observable.create(new OnSubscribe>() {
@Override
public void call(final Subscriber super Observable> subscriber) {
final BooleanSubscription subscription = new BooleanSubscription();
// clean up state if unsubscribe
subscriber.add(new Subscription() {
@Override
public void unsubscribe() {
// NOTE, this assumes one
// unsubscribe should
// clear all state. Which
// is ok if the O>
// is published.refCounted()
mergedObservable.clear();
subscription.unsubscribe();
}
@Override
public boolean isUnsubscribed() {
return subscription.isUnsubscribed();
}
});
subscriber.add(mergedObservable.get().subscribe(new Observer>() {
@Override
public void onCompleted() {
subscriber.onCompleted();
}
@Override
public void onError(Throwable e) {
subscriber.onError(e);
}
@Override
public void onNext(Observable t) {
subscriber.onNext(t);
}
}));
subscriber.add(endpointInjector.deltas().subscribe(new Action1() {
@Override
public void call(EndpointChange ec) {
String id = Endpoint.uniqueHost(ec.getEndpoint().getHost(), ec.getEndpoint().getPort(), ec.getEndpoint().getSlotId());
if (EndpointChange.Type.add == ec.getType()) {
logger.info("Adding new connection to host: " + ec.getEndpoint().getHost() + " at port: " + ec.getEndpoint().getPort() +
" with id: " + id);
mergedObservable.mergeIn(id, toObservableFunc.call(ec.getEndpoint()), ec.getEndpoint().getErrorCallback(),
ec.getEndpoint().getCompletedCallback());
} else if (EndpointChange.Type.complete == ec.getType()) {
logger.info("Forcing connection to complete host: " + ec.getEndpoint().getHost() + " at port: " +
ec.getEndpoint().getPort() + " with id: " + id);
mergedObservable.forceComplete(id);
}
}
}));
}
});
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy