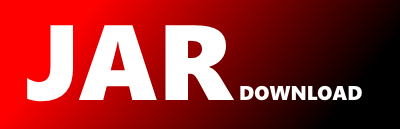
io.reactivex.mantis.remote.observable.ServeNestedObservable Maven / Gradle / Ivy
/*
* Copyright 2019 Netflix, Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package io.reactivex.mantis.remote.observable;
import io.mantisrx.common.codec.Encoder;
import io.reactivex.mantis.remote.observable.filter.ServerSideFilters;
import io.reactivex.mantis.remote.observable.slotting.RoundRobin;
import io.reactivex.mantis.remote.observable.slotting.SlottingStrategy;
import java.util.Map;
import rx.Observable;
import rx.Observer;
import rx.Subscription;
import rx.functions.Action0;
import rx.functions.Func1;
public class ServeNestedObservable extends ServeConfig {
private Encoder encoder;
public ServeNestedObservable(Builder builder) {
super(builder.name, builder.slottingStrategy, builder.filterFunction,
builder.maxWriteAttempts);
this.encoder = builder.encoder;
applySlottingSideEffectToObservable(builder.observable);
}
private void applySlottingSideEffectToObservable(Observable> o) {
final Observable withSideEffects =
Observable.merge(o)
.doOnEach(new Observer() {
@Override
public void onCompleted() {
slottingStrategy.completeAllConnections();
}
@Override
public void onError(Throwable e) {
slottingStrategy.errorAllConnections(e);
}
@Override
public void onNext(T value) {
slottingStrategy.writeOnSlot(null, value);
}
});
final MutableReference subscriptionRef = new MutableReference<>();
slottingStrategy.registerDoAfterFirstConnectionAdded(new Action0() {
@Override
public void call() {
subscriptionRef.setValue(withSideEffects.subscribe());
}
});
slottingStrategy.registerDoAfterLastConnectionRemoved(new Action0() {
@Override
public void call() {
subscriptionRef.getValue().unsubscribe();
}
});
}
public Encoder getEncoder() {
return encoder;
}
public static class Builder {
private String name;
private Observable> observable;
private SlottingStrategy slottingStrategy = new RoundRobin<>();
private Encoder encoder;
private Func1
© 2015 - 2024 Weber Informatics LLC | Privacy Policy