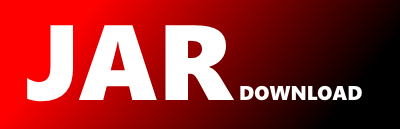
commonMain.maryk.core.values.Values.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of core-jvm Show documentation
Show all versions of core-jvm Show documentation
Maryk is a Kotlin Multiplatform library which helps you to store, query and send data in a structured way over multiple platforms. The data store stores any value with a version, so it is possible to request only the changed data or live listen for updates.
The newest version!
package maryk.core.values
import maryk.core.models.IsValuesDataModel
import maryk.core.models.TypedValuesDataModel
import maryk.core.models.validate
import maryk.core.properties.graph.IsPropRefGraph
import maryk.core.query.RequestContext
import maryk.core.query.changes.IsChange
typealias ValuesImpl = Values
/**
* Contains a [map] with all values related to a DataObject of [dataModel]
*/
data class Values internal constructor(
override val dataModel: DM,
override val values: IsValueItems,
override val context: RequestContext? = null
) : AbstractValues() {
/** make a copy of Values and add new pairs from [pairCreator] */
fun copy(pairCreator: DM.() -> List) =
Values(
dataModel,
values.copyAdding(pairCreator(dataModel)),
context
)
fun copy(values: IsValueItems) =
Values(dataModel, values.copyAdding(values), context)
fun filterWithSelect(select: IsPropRefGraph<*>?): Values {
if (select == null) {
return this
}
return Values(
dataModel = dataModel,
values = this.values.copySelecting(select),
context = context,
)
}
/** Change the Values with given [change] */
fun change(vararg change: IsChange) = this.change(listOf(*change))
fun change(changes: List): Values =
if (changes.isEmpty()) {
this
} else {
val valueItemsToChange = MutableValueItems(mutableListOf())
for (change in changes) {
change.changeValues { ref, valueChanger ->
valueItemsToChange.copyFromOriginalAndChange(this.values, ref.index, valueChanger)
}
}
Values(dataModel, values.copyAdding(valueItemsToChange), context)
}
// ignore context
override fun equals(other: Any?) = when {
this === other -> true
other !is Values<*> -> false
dataModel != other.dataModel -> false
values != other.values -> false
else -> true
}
// ignore context
override fun hashCode(): Int {
var result = dataModel.Meta.hashCode()
result = 31 * result + values.hashCode()
return result
}
override fun toString(): String {
val modelName = dataModel.Meta.name
return "Values<$modelName>${values.toString(dataModel)}"
}
/**
* Validates the contents of values
*/
fun validate() {
this.dataModel.validate(this)
}
}
/** Output values to a json string */
fun , DM: TypedValuesDataModel> V.toJson(
pretty: Boolean = false
): String =
this.dataModel.Serializer.writeJson(this, pretty = pretty)
© 2015 - 2025 Weber Informatics LLC | Privacy Policy