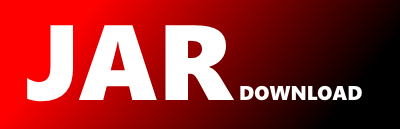
io.mats3.util.eagercache.MatsEagerCacheHtmlGui Maven / Gradle / Ivy
Show all versions of mats-util Show documentation
package io.mats3.util.eagercache;
import static io.mats3.util.eagercache.MatsEagerCacheServer._formatHtmlBytes;
import static io.mats3.util.eagercache.MatsEagerCacheServer._formatHtmlTimestamp;
import static io.mats3.util.eagercache.MatsEagerCacheServer._formatMillis;
import java.io.IOException;
import java.util.Map;
import io.mats3.util.eagercache.MatsEagerCacheClient.CacheClientInformation;
import io.mats3.util.eagercache.MatsEagerCacheServer.CacheServerInformation;
/**
* Embeddable HTML GUI for the {@link MatsEagerCacheClient} and {@link MatsEagerCacheServer}.
*/
public interface MatsEagerCacheHtmlGui {
static MatsEagerCacheHtmlGui create(CacheClientInformation info) {
return new MatsEagerCacheClientHtmlGui(info);
}
static MatsEagerCacheHtmlGui create(CacheServerInformation info) {
return new MatsEagerCacheServerHtmlGui(info);
}
/**
* Note: The output from this method is static, it can be written directly to the HTML page in a script-tag, or
* included as a separate file (with hard caching). It shall only be included once even if there are several GUIs on
* the same page.
*/
void outputStyleSheet(Appendable out) throws IOException;
/**
* Note: The output from this method is static, it can be written directly to the HTML page in a style-tag, or
* included as a separate file (with hard caching). It shall only be included once even if there are several GUIs on
* the same page.
*/
void outputJavaScript(Appendable out) throws IOException;
/**
* The embeddable HTML GUI - map this to GET, content type is "text/html; charset=utf-8"
. This might
* via the browser call back to {@link #json(Appendable, Map, String)} - which you also must mount at (typically)
* the same URL (PUT, POST and DELETEs go there, GETs go here).
*/
void html(Appendable out, Map requestParameters) throws IOException;
/**
* The HTML GUI will invoke JSON-over-HTTP to the same URL it is located at - map this to PUT, POST and DELETE,
* returned content type shall be "application/json; charset=utf-8"
.
*
* NOTICE: If you have several GUIs on the same path, you must route them to the correct instance. This is done by
* the URL parameters 'dataname' and 'nodename' which will always be supplied by the GUI when doing operations.
*
* NOTICE: If you need to change the JSON Path, i.e. the path which this GUI employs to do "active" operations, you
* can do so by setting the JS global variable "matsec_json_path" when outputting the HTML, overriding the default
* which is to use the current URL path (i.e. the same as the GUI is served on). They may be on the same path since
* the HTML is served using GET, while the JSON uses PUT, POST and DELETE with header "Content-Type:
* application/json".
*/
void json(Appendable out, Map requestParameters, String requestBody) throws IOException;
/**
* Implementation of {@link MatsEagerCacheHtmlGui} for {@link MatsEagerCacheClient} - use the
* {@link MatsEagerCacheHtmlGui#create(CacheClientInformation)} factory method to get an instance.
*/
class MatsEagerCacheClientHtmlGui implements MatsEagerCacheHtmlGui {
private final CacheClientInformation _info;
private MatsEagerCacheClientHtmlGui(CacheClientInformation info) {
_info = info;
}
@Override
public void outputStyleSheet(Appendable out) throws IOException {
out.append("/* No CSS for MatsEagerCacheClient */");
}
@Override
public void outputJavaScript(Appendable out) throws IOException {
out.append("/* No JavaScript for MatsEagerCacheClient */");
}
@Override
public void html(Appendable out, Map requestParameters) throws IOException {
out.append("MatsEagerCacheClient '").append(_info.getDataName()).append("' @ '")
.append(_info.getNodename()).append("'
\n");
out.append("DataName: ").append("").append(_info.getDataName()).append("
\n");
out.append("Nodename: ").append("").append(_info.getNodename()).append("
\n");
out.append("LifeCycle: ").append("").append(_info.getCacheClientLifeCycle().toString()).append(
"
\n");
out.append("CacheStartedTimestamp: ")
.append(_formatHtmlTimestamp(_info.getCacheStartedTimestamp())).append("
\n");
out.append("BroadcastTopic: ").append("").append(_info.getBroadcastTopic()).append("
\n");
out.append("InitialPopulationRequestSentTimestamp: ")
.append(_formatHtmlTimestamp(_info.getInitialPopulationRequestSentTimestamp())).append("
\n");
boolean initial = _info.isInitialPopulationDone();
long millisBetween = _info.getInitialPopulationTimestamp() -
_info.getInitialPopulationRequestSentTimestamp();
out.append("InitialPopulationDone: ").append(initial
? "Done @ " + _formatHtmlTimestamp(_info.getInitialPopulationTimestamp())
+ " - " + _formatMillis(millisBetween) + " after request"
: "Waiting")
.append("
\n");
out.append("
\n");
out.append("LastAnypdateReceivedTimestamp: ")
.append(_formatHtmlTimestamp(_info.getAnyUpdateReceivedTimestamp())).append("
\n");
out.append("LastFullUpdateReceivedTimestamp: ")
.append(_formatHtmlTimestamp(_info.getLastFullUpdateReceivedTimestamp())).append("
\n");
out.append("LastPartialUpdateReceivedTimestamp: ")
.append(_formatHtmlTimestamp(_info.getLastPartialUpdateReceivedTimestamp())).append("
\n");
out.append("
\n");
if (initial) {
out.append("LastUpdateType: ").append("")
.append(_info.isLastUpdateFull() ? "Full" : "Partial").append("
\n");
out.append("LastUpdateMode: ").append("")
.append(_info.isLastUpdateLarge() ? "LARGE" : "Small").append("
\n");
out.append("LastUpdateDurationMillis: ")
.append(_formatMillis(_info.getLastUpdateDurationMillis())).append("
\n");
String meta = _info.getLastUpdateMetadata();
out.append("LastUpdateMetadata: ").append(meta != null ? "" + meta + "" : "none")
.append("
\n");
out.append("LastUpdateCompressedSize: ")
.append(_formatHtmlBytes(_info.getLastUpdateCompressedSize())).append("
\n");
out.append("LastUpdateUncompressedSize: ")
.append(_formatHtmlBytes(_info.getLastUpdateUncompressedSize())).append("
\n");
out.append("LastUpdateCount: ").append("")
.append(Integer.toString(_info.getLastUpdateCount())).append("
\n");
out.append("
\n");
}
else {
out.append("Initial population not done yet.
\n");
}
out.append("NumberOfFullUpdatesReceived: ").append("")
.append(Integer.toString(_info.getNumberOfFullUpdatesReceived())).append("
\n");
out.append("NumberOfPartialUpdatesReceived: ").append("")
.append(Integer.toString(_info.getNumberOfPartialUpdatesReceived())).append("
\n");
out.append("NumberOfAccesses: ").append("")
.append(Long.toString(_info.getNumberOfAccesses())).append("
\n");
}
@Override
public void json(Appendable out, Map requestParameters, String requestBody)
throws IOException {
out.append("/* No JSON for MatsEagerCacheClient */");
}
}
/**
* Implementation of {@link MatsEagerCacheHtmlGui} for {@link MatsEagerCacheServer} - use the
* {@link MatsEagerCacheHtmlGui#create(CacheServerInformation)} factory method to get an instance.
*/
class MatsEagerCacheServerHtmlGui implements MatsEagerCacheHtmlGui {
private final CacheServerInformation _info;
private MatsEagerCacheServerHtmlGui(CacheServerInformation info) {
_info = info;
}
@Override
public void outputStyleSheet(Appendable out) throws IOException {
out.append("/* No CSS for MatsEagerCacheServer */");
}
@Override
public void outputJavaScript(Appendable out) throws IOException {
out.append("/* No JavaScript for MatsEagerCacheServer */");
}
@Override
public void html(Appendable out, Map requestParameters) throws IOException {
out.append("MatsEagerCacheServer '" + _info.getDataName() + "' @ '" + _info.getNodename() + "'
");
out.append("DataName: ").append("").append(_info.getDataName()).append("
\n");
out.append("Nodename: ").append("").append(_info.getNodename()).append("
\n");
out.append("LifeCycle: ").append("").append(_info.getCacheServerLifeCycle().toString()).append(
"
\n");
out.append("CacheStartedTimestamp: ")
.append(_formatHtmlTimestamp(_info.getCacheStartedTimestamp())).append("
\n");
out.append("CacheRequestQueue: ").append("").append(_info.getCacheRequestQueue()).append("
\n");
out.append("BroadcastTopic: ").append("").append(_info.getBroadcastTopic()).append("
\n");
out.append("PeriodicFullUpdateIntervalMinutes: ").append("")
.append(Double.toString(_info.getPeriodicFullUpdateIntervalMinutes())).append("
\n");
out.append("
\n");
out.append("LastFullUpdateRequestReceivedTimestamp: ")
.append(_formatHtmlTimestamp(_info.getLastFullUpdateRequestReceivedTimestamp())).append("
\n");
out.append("LastFullUpdateProductionStartedTimestamp: ")
.append(_formatHtmlTimestamp(_info.getLastFullUpdateProductionStartedTimestamp()))
.append(" - took ").append(_formatMillis(_info.getLastFullUpdateProduceTotalMillis()))
.append("
\n");
out.append("LastFullUpdateSentTimestamp: ")
.append(_formatHtmlTimestamp(_info.getLastFullUpdateSentTimestamp())).append("
\n");
out.append("LastFullUpdateReceivedTimestamp: ")
.append(_formatHtmlTimestamp(_info.getLastFullUpdateReceivedTimestamp())).append("
\n");
out.append("LastPartialUpdateReceivedTimestamp: ")
.append(_formatHtmlTimestamp(_info.getLastPartialUpdateReceivedTimestamp())).append("
\n");
out.append("LastAnyUpdateReceivedTimestamp: ")
.append(_formatHtmlTimestamp(_info.getLastAnyUpdateReceivedTimestamp())).append("
\n");
out.append("
\n");
long lastUpdateSent = _info.getLastUpdateSentTimestamp();
if (lastUpdateSent > 0) {
out.append("LastUpdateSentTimestamp: ").append("")
.append(_formatHtmlTimestamp(lastUpdateSent)).append("
\n");
out.append("LastUpdateType: ").append("")
.append(_info.isLastUpdateFull() ? "Full" : "Partial").append("
\n");
out.append("LastUpdateProductionTotalMillis: ")
.append(_formatMillis(_info.getLastUpdateProduceTotalMillis()))
.append(" - source: ")
.append(_formatMillis(_info.getLastUpdateSourceMillis()))
.append(", serialize: ")
.append(_formatMillis(_info.getLastUpdateSerializeMillis()))
.append(", compress: ")
.append(_formatMillis(_info.getLastUpdateCompressMillis()))
.append("
\n");
String meta = _info.getLastUpdateMetadata();
out.append("LastUpdateMetadata: ").append(meta != null ? "" + meta + "" : "none")
.append("
\n");
out.append("LastUpdateCount: ").append("")
.append(Integer.toString(_info.getLastUpdateCount())).append("
\n");
out.append("LastUpdateUncompressedSize: ")
.append(_formatHtmlBytes(_info.getLastUpdateUncompressedSize())).append("
\n");
out.append("LastUpdateCompressedSize: ")
.append(_formatHtmlBytes(_info.getLastUpdateCompressedSize())).append("
\n");
out.append("
\n");
}
else {
out.append("No update sent yet.
\n");
}
out.append("FullUpdates: ").append(Integer.toString(_info.getNumberOfFullUpdatesSent()));
out.append(" sent, out of ").append(Integer.toString(_info.getNumberOfFullUpdatesReceived()))
.append(" received.
\n");
out.append("PartialUpdates: ").append(Integer.toString(_info.getNumberOfPartialUpdatesSent()));
out.append(" sent, out of ").append(Integer.toString(_info.getNumberOfPartialUpdatesReceived()))
.append(" received.
\n");
}
@Override
public void json(Appendable out, Map requestParameters, String requestBody)
throws IOException {
out.append("/* No JSON for MatsEagerCacheServer */");
}
}
}