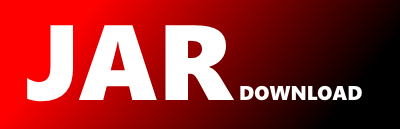
io.microconfig.core.environments.EnvironmentImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of microconfig-core Show documentation
Show all versions of microconfig-core Show documentation
Powerful tool for microservice configuration management
package io.microconfig.core.environments;
import io.microconfig.core.properties.PropertiesFactory;
import lombok.Getter;
import lombok.RequiredArgsConstructor;
import java.io.File;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import java.util.function.Predicate;
import java.util.function.Supplier;
import static io.microconfig.utils.Logger.info;
import static io.microconfig.utils.StreamUtils.*;
import static java.util.Objects.requireNonNull;
import static java.util.function.Function.identity;
import static java.util.stream.Collectors.toList;
import static java.util.stream.Collectors.toMap;
@RequiredArgsConstructor
public class EnvironmentImpl implements Environment {
@Getter
private final File source;
@Getter
private final String name;
@Getter
private final int portOffset;
@Getter
private final List groups;
private final ComponentFactory componentFactory;
private final PropertiesFactory propertiesFactory;
@Override
public List findGroupsWithIp(String ip) {
return filter(groups, g -> g.getIp().filter(ip::equals).isPresent());
}
@Override
public ComponentGroup getGroupWithName(String groupName) {
return findGroup(group -> group.getName().equals(groupName),
() -> "groupName=" + groupName);
}
@Override
public Optional findGroupWithComponent(String componentName) {
return groups.stream()
.filter(g -> g.findComponentWithName(componentName).isPresent())
.findFirst();
}
@Override
public Components getAllComponents() {
List components = groups.stream()
.map(ComponentGroup::getComponents)
.map(Components::asList)
.flatMap(List::stream)
.collect(toList());
return new ComponentsImpl(components, propertiesFactory);
}
@Override
public Component getComponentWithName(String componentName) {
return findFirstResult(groups, g -> g.findComponentWithName(componentName))
.orElseThrow(() -> new IllegalArgumentException(notFoundComponentMessage(componentName)));
}
@Override
public Components findComponentsFrom(List groups, List componentNames) {
List componentsFromGroups = componentsFrom(groups);
List result = filterBy(componentNames, componentsFromGroups);
info("Filtered " + result.size() + " component(s) in [" + name + "] env.");
return new ComponentsImpl(result, propertiesFactory);
}
@Override
public Component getOrCreateComponentWithName(String componentName) {
return findFirstResult(groups, g -> g.findComponentWithName(componentName))
.orElseGet(() -> componentFactory.createComponent(componentName, componentName, name));
}
private List componentsFrom(List groups) {
if (groups.isEmpty()) return getAllComponents().asList();
return groups.stream()
.map(this::getGroupWithName)
.map(ComponentGroup::getComponents)
.map(Components::asList)
.flatMap(List::stream)
.collect(toList());
}
private List filterBy(List components, List componentFromGroups) {
if (components.isEmpty()) return componentFromGroups;
Map componentByName = componentFromGroups.stream()
.collect(toMap(Component::getName, identity()));
return forEach(components, c -> requireNonNull(componentByName.get(c), () -> notFoundComponentMessage(c)));
}
private ComponentGroup findGroup(Predicate groupPredicate,
Supplier description) {
return groups.stream()
.filter(groupPredicate)
.findFirst()
.orElseThrow(() -> new IllegalArgumentException("Can't find group by filter: '" + description.get() + "' in env '" + name + "'"));
}
private String notFoundComponentMessage(String component) {
return "Component '" + component + "' is not configured for env '" + name + "'";
}
@Override
public String toString() {
return name + ": " + groups;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy