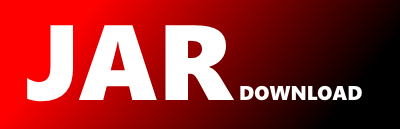
io.micronaut.data.model.jpa.criteria.impl.AbstractPersistentEntityCriteriaDelete Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of micronaut-data-model Show documentation
Show all versions of micronaut-data-model Show documentation
Data Repository Support for Micronaut
/*
* Copyright 2017-2021 original authors
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package io.micronaut.data.model.jpa.criteria.impl;
import io.micronaut.core.annotation.Internal;
import io.micronaut.core.annotation.NonNull;
import io.micronaut.data.annotation.Join;
import io.micronaut.data.model.PersistentEntity;
import io.micronaut.data.model.jpa.criteria.IExpression;
import io.micronaut.data.model.jpa.criteria.PersistentEntityCriteriaDelete;
import io.micronaut.data.model.jpa.criteria.PersistentEntityRoot;
import io.micronaut.data.model.jpa.criteria.impl.predicate.ConjunctionPredicate;
import io.micronaut.data.model.jpa.criteria.impl.query.QueryModelPredicateVisitor;
import io.micronaut.data.model.jpa.criteria.impl.util.Joiner;
import io.micronaut.data.model.query.QueryModel;
import io.micronaut.data.model.query.builder.QueryBuilder;
import io.micronaut.data.model.query.builder.QueryResult;
import jakarta.persistence.criteria.Expression;
import jakarta.persistence.criteria.Predicate;
import jakarta.persistence.criteria.Subquery;
import jakarta.persistence.metamodel.EntityType;
import java.util.Arrays;
import java.util.Collections;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.stream.Collectors;
/**
* The abstract implementation of {@link PersistentEntityCriteriaDelete}.
*
* @param The entity type
* @author Denis Stepanov
* @since 3.2
*/
@Internal
public abstract class AbstractPersistentEntityCriteriaDelete implements PersistentEntityCriteriaDelete,
QueryResultPersistentEntityCriteriaQuery {
protected Predicate predicate;
protected PersistentEntityRoot entityRoot;
@Override
public QueryModel getQueryModel() {
if (entityRoot == null) {
throw new IllegalStateException("The root entity must be specified!");
}
QueryModel qm = QueryModel.from(entityRoot.getPersistentEntity());
Joiner joiner = new Joiner();
if (predicate instanceof PredicateVisitable) {
PredicateVisitable predicate = (PredicateVisitable) this.predicate;
predicate.accept(createPredicateVisitor(qm));
predicate.accept(joiner);
}
for (Map.Entry e : joiner.getJoins().entrySet()) {
qm.join(e.getKey(), Optional.ofNullable(e.getValue().getType()).orElse(Join.Type.DEFAULT), e.getValue().getAlias());
}
return qm;
}
/**
* Creates query model predicate visitor.
* @param queryModel The query model
* @return the visitor
*/
@NonNull
protected QueryModelPredicateVisitor createPredicateVisitor(QueryModel queryModel) {
return new QueryModelPredicateVisitor(queryModel);
}
@Override
public QueryResult buildQuery(QueryBuilder queryBuilder) {
return queryBuilder.buildDelete(getQueryModel());
}
@Override
public abstract PersistentEntityRoot from(Class entityClass);
@Override
public abstract PersistentEntityRoot from(PersistentEntity persistentEntity);
@Override
public PersistentEntityRoot from(EntityType entity) {
throw CriteriaUtils.notSupportedOperation();
}
@Override
public PersistentEntityCriteriaDelete where(Expression restriction) {
predicate = new ConjunctionPredicate(Collections.singleton((IExpression) restriction));
return this;
}
@Override
public PersistentEntityCriteriaDelete where(Predicate... restrictions) {
Objects.requireNonNull(restrictions);
if (restrictions.length > 0) {
predicate = restrictions.length == 1 ? restrictions[0] : new ConjunctionPredicate(
Arrays.stream(restrictions).sequential().map(x -> (IExpression) x).collect(Collectors.toList())
);
} else {
predicate = null;
}
return this;
}
@Override
public PersistentEntityRoot getRoot() {
return entityRoot;
}
@Override
public Predicate getRestriction() {
return predicate;
}
@Override
public Subquery subquery(Class type) {
throw new IllegalStateException("Unsupported!");
}
public final boolean hasVersionRestriction() {
if (entityRoot.getPersistentEntity().getVersion() == null) {
return false;
}
return CriteriaUtils.hasVersionPredicate(predicate);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy