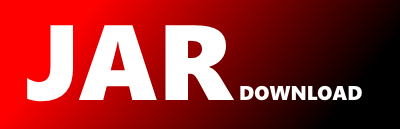
io.micronaut.data.operations.async.AsyncRepositoryOperations Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of micronaut-data-model Show documentation
Show all versions of micronaut-data-model Show documentation
Data Repository Support for Micronaut
/*
* Copyright 2017-2020 original authors
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package io.micronaut.data.operations.async;
import io.micronaut.core.annotation.NonNull;
import io.micronaut.core.annotation.NonBlocking;
import io.micronaut.core.async.annotation.SingleResult;
import io.micronaut.data.model.Page;
import io.micronaut.data.model.runtime.*;
import java.util.concurrent.CompletionStage;
import java.util.concurrent.Executor;
/**
* Asynchronous operations for reading data from a backing implementations.
*
* @author graemerocher
* @since 1.0.0
*/
@NonBlocking
public interface AsyncRepositoryOperations {
/**
* @return The executor used by this async operations
*/
@NonNull Executor getExecutor();
/**
* Find one by ID.
*
* @param type The type
* @param id The id
* @param The generic type
* @return A completion stage that emits the result
*/
@NonNull
CompletionStage findOne(@NonNull Class type, @NonNull Object id);
/**
* Check with an record exists for the given query.
* @param preparedQuery The query
* @param The declaring type
* @return True if it exists
*/
CompletionStage exists(@NonNull PreparedQuery preparedQuery);
/**
* Find one by Query.
*
* @param preparedQuery The prepared query
* @param The generic resultType
* @param The result type
* @return A completion stage that emits the result
*/
@NonNull CompletionStage findOne(@NonNull PreparedQuery preparedQuery);
/**
* Find one by ID.
*
* @param type The type
* @param id The id
* @param The generic type
* @return A completion stage that emits the result or null if there is no result
*/
@NonNull
CompletionStage findOptional(@NonNull Class type, @NonNull Object id);
/**
* Find one by Query.
*
* @param preparedQuery The prepared query
* @param The generic resultType
* @param The result type
* @return A completion stage that emits the result or null if there is no result
*/
@NonNull CompletionStage findOptional(@NonNull PreparedQuery preparedQuery);
/**
* Finds all results for the given query.
* @param pagedQuery The paged query
* @param The generic type
* @return A completion stage that emits the results
*/
@NonNull CompletionStage> findAll(PagedQuery pagedQuery);
/**
* Counts all results for the given query.
* @param pagedQuery The paged query
* @param The generic type
* @return A completion stage that emits the count as a long
*/
@NonNull CompletionStage count(PagedQuery pagedQuery);
/**
* Finds all results for the given query.
* @param preparedQuery The prepared query
* @param The entity type
* @param The result type
* @return A completion stage that emits an iterable with all results
*/
@NonNull CompletionStage> findAll(@NonNull PreparedQuery preparedQuery);
/**
* Persist the entity returning a possibly new entity.
* @param operation The entity operation
* @param The generic type
* @return A completion stage that emits the entity
*/
@NonNull CompletionStage persist(@NonNull InsertOperation operation);
/**
* Updates the entity returning a possibly new entity.
* @param operation The entity operation
* @param The generic type
* @return A completion stage that emits the entity
*/
@NonNull CompletionStage update(@NonNull UpdateOperation operation);
/**
* Updates the entities for the given operation.
*
* @param operation The operation
* @param The generic type
* @return The updated entities
*/
default @NonNull CompletionStage> updateAll(@NonNull UpdateBatchOperation operation) {
throw new UnsupportedOperationException("The updateAll is required to be implemented.");
}
/**
* Deletes the entity.
* @param operation The batch operation
* @param The generic type
* @return A publisher that emits the number of entities deleted
*/
@SingleResult
@NonNull
CompletionStage delete(@NonNull DeleteOperation operation);
/**
* Persist all the given entities.
* @param operation The batch operation
* @param The generic type
* @return The entities, possibly mutated
*/
@NonNull CompletionStage> persistAll(@NonNull InsertBatchOperation operation);
/**
* Executes an update for the given query and parameter values. If it is possible to
* return the number of objects updated, then do so.
* @param preparedQuery The prepared query
* @return A completion that emits a boolean true if successful
*/
@NonNull
CompletionStage executeUpdate(
@NonNull PreparedQuery, Number> preparedQuery
);
/**
* Executes a delete batch for the given query and parameter values. If it is possible to
* return the number of objects updated, then do so.
* @param preparedQuery The prepared query
* @return A completion that emits a boolean true if successful
*/
@NonNull
default CompletionStage executeDelete(
@NonNull PreparedQuery, Number> preparedQuery
) {
return executeUpdate(preparedQuery);
}
/**
* Deletes all the entities of the given type.
* @param operation The batch operation
* @param The generic type
* @return A completion that emits a boolean true if successful
*/
@NonNull CompletionStage deleteAll(@NonNull DeleteBatchOperation operation);
/**
* Find a page for the given entity and pageable.
* @param pagedQuery The paged query
* @param The entity generic type
* @return The page type
*/
@NonNull CompletionStage> findPage(@NonNull PagedQuery pagedQuery);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy