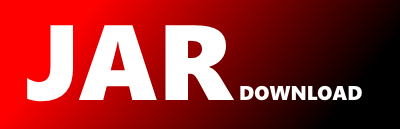
io.micronaut.data.model.jpa.criteria.impl.AbstractPersistentEntityCriteriaUpdate Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of micronaut-data-model Show documentation
Show all versions of micronaut-data-model Show documentation
Data Repository Support for Micronaut
The newest version!
/*
* Copyright 2017-2021 original authors
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package io.micronaut.data.model.jpa.criteria.impl;
import io.micronaut.core.annotation.AnnotationMetadata;
import io.micronaut.core.annotation.Internal;
import io.micronaut.core.annotation.NonNull;
import io.micronaut.data.model.PersistentEntity;
import io.micronaut.data.model.jpa.criteria.ExpressionType;
import io.micronaut.data.model.jpa.criteria.IExpression;
import io.micronaut.data.model.jpa.criteria.PersistentEntityCriteriaUpdate;
import io.micronaut.data.model.jpa.criteria.PersistentEntityRoot;
import io.micronaut.data.model.jpa.criteria.PersistentEntitySubquery;
import io.micronaut.data.model.jpa.criteria.impl.AbstractPersistentEntityQuery.BaseQueryDefinitionImpl;
import io.micronaut.data.model.jpa.criteria.impl.predicate.ConjunctionPredicate;
import io.micronaut.data.model.jpa.criteria.impl.selection.CompoundSelection;
import io.micronaut.data.model.query.builder.QueryBuilder2;
import io.micronaut.data.model.query.builder.QueryResult;
import jakarta.persistence.criteria.Expression;
import jakarta.persistence.criteria.ParameterExpression;
import jakarta.persistence.criteria.Path;
import jakarta.persistence.criteria.Predicate;
import jakarta.persistence.criteria.Selection;
import jakarta.persistence.metamodel.EntityType;
import jakarta.persistence.metamodel.SingularAttribute;
import java.util.Arrays;
import java.util.Collections;
import java.util.LinkedHashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Set;
import static io.micronaut.data.model.jpa.criteria.impl.CriteriaUtils.notSupportedOperation;
import static io.micronaut.data.model.jpa.criteria.impl.CriteriaUtils.requireParameter;
import static io.micronaut.data.model.jpa.criteria.impl.CriteriaUtils.requireProperty;
/**
* The abstract implementation of {@link PersistentEntityCriteriaUpdate}.
*
* @param The entity type
* @author Denis Stepanov
* @since 3.2
*/
@Internal
public abstract class AbstractPersistentEntityCriteriaUpdate implements PersistentEntityCriteriaUpdate,
QueryResultPersistentEntityCriteriaQuery {
protected Predicate predicate;
protected PersistentEntityRoot entityRoot;
protected Map updateValues = new LinkedHashMap<>();
protected Selection> returning;
@Override
public QueryResult buildQuery(AnnotationMetadata annotationMetadata, QueryBuilder2 queryBuilder) {
return queryBuilder.buildUpdate(
annotationMetadata,
new UpdateQueryDefinitionImpl(entityRoot.getPersistentEntity(), predicate, returning, updateValues)
);
}
@Override
public abstract PersistentEntityRoot from(Class entityClass);
@Override
public abstract PersistentEntityRoot from(PersistentEntity persistentEntity);
@Override
public PersistentEntityRoot from(EntityType entity) {
if (entityRoot != null) {
throw new IllegalStateException("The root entity is already specified!");
}
return null;
}
@Override
public PersistentEntityRoot getRoot() {
return entityRoot;
}
@Override
public PersistentEntityCriteriaUpdate set(SingularAttribute super T, Y> attribute, X value) {
throw notSupportedOperation();
}
@Override
public PersistentEntityCriteriaUpdate set(SingularAttribute super T, Y> attribute, Expression extends Y> value) {
throw notSupportedOperation();
}
@Override
public PersistentEntityCriteriaUpdate set(Path attribute, X value) {
setValue(requireProperty(attribute).getPathAsString(), value);
return this;
}
@Override
public PersistentEntityCriteriaUpdate set(Path attribute, Expression extends Y> value) {
setValue(requireProperty(attribute).getPathAsString(), requireParameter(value));
return this;
}
@Override
public PersistentEntityCriteriaUpdate set(String attributeName, Object value) {
setValue(attributeName, value);
return this;
}
/**
* Set update value.
*
* @param attributeName The attribute name
* @param value The value
*/
protected void setValue(String attributeName, Object value) {
updateValues.put(attributeName, value);
}
@Override
public PersistentEntityCriteriaUpdate where(Expression restriction) {
if (restriction instanceof ConjunctionPredicate conjunctionPredicate) {
predicate = conjunctionPredicate;
} else {
predicate = new ConjunctionPredicate(Collections.singleton((IExpression) restriction));
}
return this;
}
@Override
public PersistentEntityCriteriaUpdate where(Predicate... restrictions) {
Objects.requireNonNull(restrictions);
if (restrictions.length > 0) {
predicate = restrictions.length == 1 ? restrictions[0] : new ConjunctionPredicate(
Arrays.stream(restrictions).sequential().map(x -> (IExpression) x).toList()
);
} else {
predicate = null;
}
return this;
}
@Override
public final Predicate getRestriction() {
return predicate;
}
@Override
public PersistentEntitySubquery subquery(ExpressionType type) {
throw notSupportedOperation();
}
public final boolean hasVersionRestriction() {
if (entityRoot.getPersistentEntity().getVersion() == null) {
return false;
}
return CriteriaUtils.hasVersionPredicate(predicate);
}
public final Map getUpdateValues() {
return updateValues;
}
@Override
public Set> getParameters() {
return CriteriaUtils.extractPredicateParameters(predicate);
}
@Override
public PersistentEntityCriteriaUpdate returning(Selection extends T> selection) {
Objects.requireNonNull(selection);
this.returning = selection;
return this;
}
@Override
public PersistentEntityCriteriaUpdate returningMulti(List> selectionList) {
Objects.requireNonNull(selectionList);
if (!selectionList.isEmpty()) {
this.returning = new CompoundSelection<>(selectionList);
} else {
this.returning = null;
}
return this;
}
@Override
public PersistentEntityCriteriaUpdate returningMulti(@NonNull Selection>... selections) {
Objects.requireNonNull(selections);
if (selections.length != 0) {
this.returning = new CompoundSelection<>(List.of(selections));
} else {
this.returning = null;
}
return this;
}
private static final class UpdateQueryDefinitionImpl extends BaseQueryDefinitionImpl implements QueryBuilder2.UpdateQueryDefinition {
private final Map propertiesToUpdate;
private final Selection> returningSelection;
public UpdateQueryDefinitionImpl(PersistentEntity persistentEntity,
Predicate predicate,
Selection> returningSelection,
Map propertiesToUpdate) {
super(persistentEntity, predicate, Map.of());
this.propertiesToUpdate = propertiesToUpdate;
this.returningSelection = returningSelection;
}
@Override
public Map propertiesToUpdate() {
return propertiesToUpdate;
}
@Override
public Selection> returningSelection() {
return returningSelection;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy