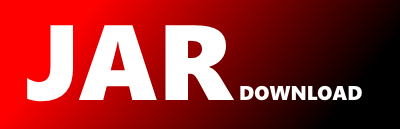
io.micronaut.data.mongodb.operations.DefaultMongoPreparedQuery Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of micronaut-data-mongodb Show documentation
Show all versions of micronaut-data-mongodb Show documentation
Data Repository Support for Micronaut
/*
* Copyright 2017-2022 original authors
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package io.micronaut.data.mongodb.operations;
import com.mongodb.client.model.Sorts;
import io.micronaut.core.annotation.Internal;
import io.micronaut.data.model.Pageable;
import io.micronaut.data.model.Pageable.Mode;
import io.micronaut.data.model.Sort;
import io.micronaut.data.model.runtime.PreparedQuery;
import io.micronaut.data.model.runtime.RuntimePersistentEntity;
import io.micronaut.data.mongodb.operations.options.MongoFindOptions;
import io.micronaut.data.runtime.operations.internal.query.DefaultBindableParametersPreparedQuery;
import io.micronaut.data.runtime.query.internal.DefaultPreparedQuery;
import io.micronaut.data.runtime.query.internal.DelegatePreparedQuery;
import io.micronaut.data.runtime.query.internal.DelegateStoredQuery;
import org.bson.BsonDocument;
import org.bson.BsonInt32;
import org.bson.conversions.Bson;
import java.util.ArrayList;
import java.util.List;
import java.util.stream.Collectors;
/**
* Default implementation of {@link MongoPreparedQuery}.
*
* @param The entity type
* @param The result type
* @author Denis Stepanov
* @since 3.3.
*/
@Internal
final class DefaultMongoPreparedQuery extends DefaultBindableParametersPreparedQuery implements DelegatePreparedQuery, MongoPreparedQuery {
private final DefaultPreparedQuery defaultPreparedQuery;
private final MongoStoredQuery mongoStoredQuery;
public DefaultMongoPreparedQuery(PreparedQuery preparedQuery) {
super(preparedQuery);
this.defaultPreparedQuery = (DefaultPreparedQuery) preparedQuery;
this.mongoStoredQuery = (MongoStoredQuery) ((DelegateStoredQuery
© 2015 - 2025 Weber Informatics LLC | Privacy Policy