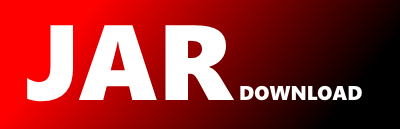
io.micronaut.data.mongodb.serde.DataDecoderContext Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of micronaut-data-mongodb Show documentation
Show all versions of micronaut-data-mongodb Show documentation
Data Repository Support for Micronaut
/*
* Copyright 2017-2022 original authors
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package io.micronaut.data.mongodb.serde;
import io.micronaut.core.annotation.Internal;
import io.micronaut.core.beans.BeanIntrospection;
import io.micronaut.core.convert.ConversionContext;
import io.micronaut.core.type.Argument;
import io.micronaut.data.annotation.GeneratedValue;
import io.micronaut.data.annotation.MappedProperty;
import io.micronaut.data.document.serde.CustomConverterDeserializer;
import io.micronaut.data.document.serde.IdDeserializer;
import io.micronaut.data.document.serde.IdPropertyNamingStrategy;
import io.micronaut.data.document.serde.OneRelationDeserializer;
import io.micronaut.data.model.runtime.AttributeConverterRegistry;
import io.micronaut.data.model.runtime.convert.AttributeConverter;
import io.micronaut.data.mongodb.conf.MongoDataConfiguration;
import io.micronaut.serde.Decoder;
import io.micronaut.serde.Deserializer;
import io.micronaut.serde.LimitingStream;
import io.micronaut.serde.bson.BsonReaderDecoder;
import io.micronaut.serde.bson.custom.CodecBsonDecoder;
import io.micronaut.serde.config.naming.PropertyNamingStrategy;
import io.micronaut.serde.exceptions.SerdeException;
import io.micronaut.serde.reference.PropertyReference;
import org.bson.BsonDocument;
import org.bson.codecs.BsonDocumentCodec;
import org.bson.codecs.Codec;
import org.bson.codecs.configuration.CodecRegistry;
import org.bson.types.ObjectId;
import java.io.IOException;
import java.util.Collection;
/**
* The Micronaut Data's Serde's {@link Deserializer.DecoderContext}.
*
* @author Denis Stepanov
* @since 3.3
*/
@Internal
final class DataDecoderContext implements Deserializer.DecoderContext {
private final Argument OBJECT_ID = Argument.of(ObjectId.class);
private final MongoDataConfiguration mongoDataConfiguration;
private final AttributeConverterRegistry attributeConverterRegistry;
private final Deserializer.DecoderContext parent;
private final CodecRegistry codecRegistry;
/**
* Default constructor.
*
* @param mongoDataConfiguration The Mongo data configuration
* @param attributeConverterRegistry The attributeConverterRegistry
* @param parent The parent context
* @param codecRegistry The codec registry
*/
DataDecoderContext(MongoDataConfiguration mongoDataConfiguration,
AttributeConverterRegistry attributeConverterRegistry,
Deserializer.DecoderContext parent,
CodecRegistry codecRegistry) {
this.mongoDataConfiguration = mongoDataConfiguration;
this.attributeConverterRegistry = attributeConverterRegistry;
this.parent = parent;
this.codecRegistry = codecRegistry;
}
@Override
public PropertyReference resolveReference(PropertyReference reference) {
return parent.resolveReference(reference);
}
@Override
public > D findCustomDeserializer(Class extends D> deserializerClass) throws SerdeException {
if (deserializerClass == OneRelationDeserializer.class) {
OneRelationDeserializer oneRelationDeserializer = new OneRelationDeserializer() {
@Override
public Deserializer
© 2015 - 2025 Weber Informatics LLC | Privacy Policy