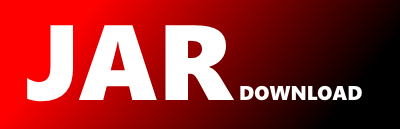
io.micronaut.data.runtime.mapper.DTOMapper Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of micronaut-data-runtime Show documentation
Show all versions of micronaut-data-runtime Show documentation
Data Repository Support for Micronaut
package io.micronaut.data.runtime.mapper;
import edu.umd.cs.findbugs.annotations.NonNull;
import edu.umd.cs.findbugs.annotations.Nullable;
import io.micronaut.core.convert.exceptions.ConversionErrorException;
import io.micronaut.core.util.ArgumentUtils;
import io.micronaut.data.exceptions.DataAccessException;
import io.micronaut.data.model.DataType;
import io.micronaut.data.model.PersistentEntity;
import io.micronaut.data.model.runtime.RuntimePersistentEntity;
import io.micronaut.data.model.runtime.RuntimePersistentProperty;
/**
* A {@link BeanIntrospectionMapper} that reads the result using the specified
* {@link PersistentEntity} and {@link ResultReader} and using the {@link #map(Object, Class)} allows mapping a result to a introspected Data Transfer Object (DTO).
*
* @param The entity type
* @param The source type.
* @param The result type
*/
public class DTOMapper implements BeanIntrospectionMapper {
private final RuntimePersistentEntity persistentEntity;
private final ResultReader resultReader;
/**
* Default constructor.
* @param persistentEntity The entity
* @param resultReader The result reader
*/
public DTOMapper(
RuntimePersistentEntity persistentEntity,
ResultReader resultReader) {
ArgumentUtils.requireNonNull("persistentEntity", persistentEntity);
ArgumentUtils.requireNonNull("resultReader", resultReader);
this.persistentEntity = persistentEntity;
this.resultReader = resultReader;
}
@Nullable
@Override
public Object read(@NonNull S object, @NonNull String name) throws ConversionErrorException {
RuntimePersistentProperty pp = persistentEntity.getPropertyByName(name);
if (pp == null) {
throw new DataAccessException("DTO projection defines a property [" + name + "] that doesn't exist on root entity: " + persistentEntity.getName());
} else {
return read(object, pp);
}
}
/**
* Read the given property.
* @param resultSet The result set
* @param property THe property
* @return The result
*/
public @Nullable Object read(@NonNull S resultSet, @NonNull RuntimePersistentProperty property) {
String propertyName = property.getName();
DataType dataType = property.getDataType();
return read(resultSet, propertyName, dataType);
}
/**
* Read the value from the given result set for the given persisted name and data type.
* @param resultSet The result set
* @param persistedName The persisted name
* @param dataType The data type
* @return The result
*/
public @Nullable Object read(@NonNull S resultSet, @NonNull String persistedName, @NonNull DataType dataType) {
return resultReader.readDynamic(
resultSet,
persistedName,
dataType
);
}
/**
* @return The entity in use
*/
public PersistentEntity getPersistentEntity() {
return persistentEntity;
}
/**
* @return the result reader
*/
public ResultReader getResultReader() {
return resultReader;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy