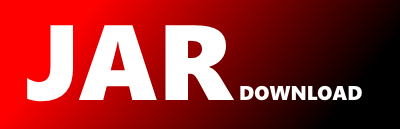
io.micronaut.data.runtime.query.internal.QueryResultStoredQuery Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of micronaut-data-runtime Show documentation
Show all versions of micronaut-data-runtime Show documentation
Data Repository Support for Micronaut
/*
* Copyright 2017-2022 original authors
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package io.micronaut.data.runtime.query.internal;
import io.micronaut.core.annotation.AnnotationMetadata;
import io.micronaut.core.annotation.Internal;
import io.micronaut.core.reflect.ClassUtils;
import io.micronaut.data.model.DataType;
import io.micronaut.data.model.JsonDataType;
import io.micronaut.data.model.query.JoinPath;
import io.micronaut.data.model.query.builder.QueryResult;
import io.micronaut.data.model.runtime.QueryParameterBinding;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Set;
/**
* The basic {@link io.micronaut.data.model.runtime.StoredQuery} created from {@link QueryResult}.
*
* @param The entity type
* @param The result type
* @author Denis Stepanov
* @since 3.5.0
*/
@Internal
public final class QueryResultStoredQuery extends BasicStoredQuery {
private final QueryResult queryResult;
private final Set joinPaths;
public QueryResultStoredQuery(String name,
AnnotationMetadata annotationMetadata,
QueryResult queryResult,
Class rootEntity,
Class resultType,
boolean pageable,
boolean isSingleResult,
boolean isCount,
OperationType operationType,
Collection joinPaths) {
super(name,
annotationMetadata,
queryResult.getQuery(),
queryResult.getParameterBindings().stream()
.anyMatch(io.micronaut.data.model.query.builder.QueryParameterBinding::isExpandable) ? queryResult.getQueryParts().toArray(new String[0]) : null,
map(queryResult.getParameterBindings()),
rootEntity,
resultType,
pageable,
isSingleResult,
isCount,
operationType);
this.queryResult = queryResult;
this.joinPaths = joinPaths == null ? Collections.emptySet() : Set.copyOf(joinPaths);
}
public static QueryResultStoredQuery single(OperationType operationType,
String name,
AnnotationMetadata annotationMetadata,
QueryResult queryResult,
Class rootEntity) {
return new QueryResultStoredQuery<>(name, annotationMetadata, queryResult, rootEntity, rootEntity, false, true, false, operationType, Collections.emptySet());
}
public static QueryResultStoredQuery single(OperationType operationType,
String name,
AnnotationMetadata annotationMetadata,
QueryResult queryResult,
Class rootEntity,
Class resultType,
Collection joinPaths) {
return new QueryResultStoredQuery<>(name, annotationMetadata, queryResult, rootEntity, resultType == Object.class ? (Class) rootEntity : resultType, false, true, false, operationType, joinPaths);
}
public static QueryResultStoredQuery many(String name,
AnnotationMetadata annotationMetadata,
QueryResult queryResult,
Class rootEntity,
boolean pageable) {
return new QueryResultStoredQuery<>(name, annotationMetadata, queryResult, rootEntity, rootEntity, pageable, false, false, OperationType.QUERY, Collections.emptySet());
}
public static QueryResultStoredQuery many(String name,
AnnotationMetadata annotationMetadata,
QueryResult queryResult,
Class rootEntity,
Class resultType,
boolean pageable,
Collection joinPaths) {
return new QueryResultStoredQuery<>(name, annotationMetadata, queryResult, rootEntity, resultType == Object.class ? (Class) rootEntity : resultType, pageable, false, false, OperationType.QUERY, joinPaths);
}
public static QueryResultStoredQuery count(String name,
AnnotationMetadata annotationMetadata,
QueryResult queryResult,
Class rootEntity) {
return new QueryResultStoredQuery<>(name, annotationMetadata, queryResult, rootEntity, Long.class, false, true, true, OperationType.COUNT, Collections.emptySet());
}
private static List map(List parameterBindings) {
List queryParameters = new ArrayList<>(parameterBindings.size());
for (io.micronaut.data.model.query.builder.QueryParameterBinding p : parameterBindings) {
queryParameters.add(
new QueryResultParameterBinding(p, queryParameters)
);
}
return queryParameters;
}
public QueryResult getQueryResult() {
return queryResult;
}
@Override
public Set getJoinFetchPaths() {
return joinPaths;
}
@Override
public Set getJoinPaths() {
return joinPaths;
}
private static class QueryResultParameterBinding implements QueryParameterBinding {
private final io.micronaut.data.model.query.builder.QueryParameterBinding p;
private final List all;
private boolean previousInitialized;
private QueryParameterBinding previousPopulatedValueParameter;
public QueryResultParameterBinding(io.micronaut.data.model.query.builder.QueryParameterBinding p, List all) {
this.p = p;
this.all = all;
}
@Override
public String getName() {
return p.getKey();
}
@Override
public DataType getDataType() {
return p.getDataType();
}
@Override
public JsonDataType getJsonDataType() {
return p.getJsonDataType();
}
@Override
public Class> getParameterConverterClass() {
if (p.getConverterClassName() == null) {
return null;
}
return ClassUtils.forName(p.getConverterClassName(), null).orElseThrow(IllegalStateException::new);
}
@Override
public int getParameterIndex() {
return p.getParameterIndex();
}
@Override
public String[] getParameterBindingPath() {
return p.getParameterBindingPath();
}
@Override
public String[] getPropertyPath() {
return p.getPropertyPath();
}
@Override
public boolean isAutoPopulated() {
return p.isAutoPopulated();
}
@Override
public boolean isRequiresPreviousPopulatedValue() {
return p.isRequiresPreviousPopulatedValue();
}
@Override
public QueryParameterBinding getPreviousPopulatedValueParameter() {
if (!previousInitialized) {
for (QueryParameterBinding it : all) {
if (it != this && it.getParameterIndex() != -1 && Arrays.equals(getPropertyPath(), it.getPropertyPath())) {
previousPopulatedValueParameter = it;
break;
}
}
previousInitialized = true;
}
return previousPopulatedValueParameter;
}
@Override
public boolean isExpandable() {
return p.isExpandable();
}
@Override
public Object getValue() {
return p.getValue();
}
@Override
public boolean isExpression() {
return p.isExpression();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy