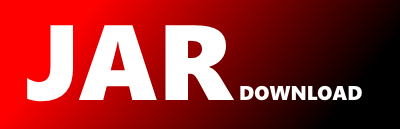
io.micronaut.discovery.consul.client.v1.ConsulOperations Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of micronaut-discovery-client Show documentation
Show all versions of micronaut-discovery-client Show documentation
Adds Service Discovery Features for Eureka and Consul
/*
* Copyright 2017-2020 original authors
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package io.micronaut.discovery.consul.client.v1;
import io.micronaut.core.annotation.Nullable;
import io.micronaut.http.HttpStatus;
import io.micronaut.http.MediaType;
import io.micronaut.http.annotation.Body;
import io.micronaut.http.annotation.Get;
import io.micronaut.http.annotation.Put;
import io.micronaut.http.annotation.QueryValue;
import io.micronaut.retry.annotation.Retryable;
import jakarta.validation.constraints.NotNull;
import org.reactivestreams.Publisher;
import java.util.List;
import java.util.Map;
/**
* API operations for Consul.
*
* @author graemerocher
* @since 1.0
*/
public interface ConsulOperations {
/**
* Writes a value for the given key to Consul.
*
* @param key The key
* @param value The value as a String
* @return A {@link Publisher} that emits a boolean if the operation succeeded
*/
@Put(value = "/kv/{+key}", processes = MediaType.TEXT_PLAIN, single = true)
Publisher putValue(String key, @Body String value);
/**
* Reads a Key from Consul. See https://www.consul.io/api/kv.html.
*
* @param key The key to read
* @return A {@link Publisher} that emits a list of {@link KeyValue}
*/
@Get(uri = "/kv/{+key}?recurse", single = true)
Publisher> readValues(String key);
/**
* Reads a Key from Consul. See https://www.consul.io/api/kv.html.
*
* @param key The key
* @param datacenter The data center
* @param raw Whether the value should be raw without encoding or metadata
* @param seperator The separator to use
* @return A {@link Publisher} that emits a list of {@link KeyValue}
*/
@Get(uri = "/kv/{+key}?recurse=true{&dc}{&raw}{&seperator}", single = true)
@Retryable(
attempts = AbstractConsulClient.EXPR_CONSUL_CONFIG_RETRY_COUNT,
delay = AbstractConsulClient.EXPR_CONSUL_CONFIG_RETRY_DELAY
)
Publisher> readValues(
String key,
@Nullable @QueryValue("dc") String datacenter,
@Nullable Boolean raw,
@Nullable String seperator);
/**
* Pass the TTL check. See https://www.consul.io/api/agent/check.html.
*
* @param checkId The check ID
* @param note An optional note
* @return An {@link HttpStatus} of {@link HttpStatus#OK} if all is well
*/
@Put("/agent/check/pass/{checkId}{?note}")
@Retryable(
attempts = AbstractConsulClient.CONSUL_REGISTRATION_RETRY_COUNT,
delay = AbstractConsulClient.CONSUL_REGISTRATION_RETRY_DELAY
)
Publisher pass(String checkId, @Nullable String note);
/**
* Warn the TTL check. See https://www.consul.io/api/agent/check.html.
*
* @param checkId The check ID
* @param note An optional note
* @return An {@link HttpStatus} of {@link HttpStatus#OK} if all is well
*/
@Put("/agent/check/warn/{checkId}{?note}")
Publisher warn(String checkId, @Nullable String note);
/**
* Fail the TTL check. See https://www.consul.io/api/agent/check.html.
*
* @param checkId The check ID
* @param note An optional note
* @return An {@link HttpStatus} of {@link HttpStatus#OK} if all is well
*/
@Put("/agent/check/fail/{checkId}{?note}")
@Retryable(
attempts = AbstractConsulClient.CONSUL_REGISTRATION_RETRY_COUNT,
delay = AbstractConsulClient.CONSUL_REGISTRATION_RETRY_DELAY
)
Publisher fail(String checkId, @Nullable String note);
/**
* @return The current leader address
*/
@Get(uri = "/status/leader", single = true)
@Retryable
Publisher status();
/**
* Register a new {@link CatalogEntry}. See https://www.consul.io/api/catalog.html.
*
* @param entry The entry to register
* @return A {@link Publisher} that emits a boolean true if the operation was successful
* @deprecated Use {@link ConsulOperations#register(ConsulCatalogEntry)} instead.
*
*/
@Put(uri = "/catalog/register", single = true)
@Deprecated(since = "4.1.0", forRemoval = true)
Publisher register(@NotNull @Body CatalogEntry entry);
/**
* Register a new {@link CatalogEntry}. See https://www.consul.io/api/catalog.html.
*
* @param entry The entry to register
* @return A {@link Publisher} that emits a boolean true if the operation was successful
* @deprecated Use {@link ConsulOperations#deregister(ConsulCatalogEntry)} instead.
*/
@Deprecated(since = "4.1.0", forRemoval = true)
Publisher deregister(@NotNull @Body CatalogEntry entry);
/**
* Register a new {@link CatalogEntry}. See https://www.consul.io/api/catalog.html.
*
* @param entry The entry to register
* @return A {@link Publisher} that emits a boolean true if the operation was successful
*/
@Put(uri = "/catalog/register", single = true)
Publisher register(@NotNull @Body ConsulCatalogEntry entry);
/**
* Register a new {@link ConsulCatalogEntry}. See https://www.consul.io/api/catalog.html.
*
* @param entry The entry to register
* @return A {@link Publisher} that emits a boolean true if the operation was successful
*/
@Put(uri = "/catalog/deregister", single = true)
Publisher deregister(@NotNull @Body ConsulCatalogEntry entry);
/**
* Register a new {@link CatalogEntry}. See https://www.consul.io/api/catalog.html.
*
* @param entry The entry to register
* @return A {@link Publisher} that emits a boolean true if the operation was successful
*/
@Put("/agent/service/register")
@Retryable(
attempts = AbstractConsulClient.CONSUL_REGISTRATION_RETRY_COUNT,
delay = AbstractConsulClient.CONSUL_REGISTRATION_RETRY_DELAY
)
Publisher register(@NotNull @Body ConsulNewServiceEntry entry);
/**
* Register a new {@link NewServiceEntry}. See https://www.consul.io/api/catalog.html.
*
* @param entry The entry to register
* @return A {@link Publisher} that emits a boolean true if the operation was successful
* @deprecated Use {@link ConsulOperations#register(ConsulNewServiceEntry)} instead.
*/
@Retryable(
attempts = AbstractConsulClient.CONSUL_REGISTRATION_RETRY_COUNT,
delay = AbstractConsulClient.CONSUL_REGISTRATION_RETRY_DELAY
)
@Deprecated(forRemoval = true, since = "4.1.0")
Publisher register(@NotNull @Body NewServiceEntry entry);
/**
* Deregister a service.
*
* @param service The service to register
* @return A {@link Publisher} that emits a boolean true if the operation was successful
*/
@Put("/agent/service/deregister/{service}")
@Retryable(
attempts = AbstractConsulClient.CONSUL_REGISTRATION_RETRY_COUNT,
delay = AbstractConsulClient.CONSUL_REGISTRATION_RETRY_DELAY
)
Publisher deregister(@NotNull String service);
/**
* Gets all of the registered services.
*
* @return The {@link ServiceEntry} instances
* @deprecated Use {@link ConsulOperations#findServices()} instead.
*/
@Deprecated(forRemoval = true, since = "4.1.0")
Publisher
© 2015 - 2025 Weber Informatics LLC | Privacy Policy