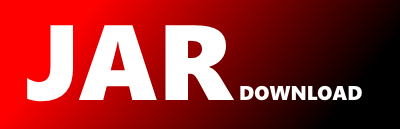
io.micronaut.jaxrs.runtime.ext.bind.UriInfoImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of micronaut-jaxrs-server Show documentation
Show all versions of micronaut-jaxrs-server Show documentation
JAX-RS Support for Micronaut
/*
* Copyright 2017-2022 original authors
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package io.micronaut.jaxrs.runtime.ext.bind;
import io.micronaut.core.annotation.Internal;
import io.micronaut.core.util.StringUtils;
import io.micronaut.http.HttpRequest;
import jakarta.ws.rs.core.MultivaluedHashMap;
import jakarta.ws.rs.core.MultivaluedMap;
import jakarta.ws.rs.core.PathSegment;
import jakarta.ws.rs.core.UriBuilder;
import jakarta.ws.rs.core.UriInfo;
import java.net.URI;
import java.net.URISyntaxException;
import java.net.URLDecoder;
import java.nio.charset.StandardCharsets;
import java.util.List;
import java.util.stream.Collectors;
import java.util.stream.Stream;
/**
* The JAX-RS {@link UriInfo} injected through {@link jakarta.ws.rs.core.Context} annotation.
*
* @author Dan Hollingsworth
* @since 3.3.0
*/
@Internal
public final class UriInfoImpl implements UriInfo {
private final HttpRequest> request;
/**
* Construct from an HTTP request.
*
* @param request The HTTP request to this URI
*/
public UriInfoImpl(HttpRequest> request) {
this.request = request;
}
private String string(String str, boolean decode) {
return decode ? URLDecoder.decode(str, StandardCharsets.UTF_8) : str;
}
@Override
public String getPath() {
return getPath(true);
}
@Override
public String getPath(boolean decode) {
return string(request.getPath(), decode);
}
@Override
public List getPathSegments() {
return getPathSegments(true);
}
@Override
public List getPathSegments(boolean decode) {
return Stream.of(request.getPath().split("/"))
.filter(StringUtils::isNotEmpty)
.map(token -> {
String[] segmentTokens = token.split(";");
MultivaluedMap params = new MultiMapNullPermitted<>();
for (int i = 1; i < segmentTokens.length; ++i) {
String[] keyVal = segmentTokens[i].split("=", 2);
String key = keyVal[0];
String val = keyVal.length > 1 ? keyVal[1] : null;
params.add(string(key, decode), string(val, decode));
}
return new UriPathSegment(string(segmentTokens[0], decode), params);
})
.collect(Collectors.toList());
}
@Override
public URI getRequestUri() {
return request.getUri();
}
/**
* This operation is not supported currently,
* so {@link UnsupportedOperationException} is thrown for all invocations.
*
* @throws UnsupportedOperationException this operation is not supported currently.
*/
@Override
public UriBuilder getRequestUriBuilder() {
throw new UnsupportedOperationException();
}
@Override
public URI getAbsolutePath() {
return getBaseUri().resolve(getPath(false));
}
/**
* This operation is not supported currently,
* so {@link UnsupportedOperationException} is thrown for all invocations.
*
* @throws UnsupportedOperationException this operation is not supported currently.
*/
@Override
public UriBuilder getAbsolutePathBuilder() {
throw new UnsupportedOperationException();
}
@Override
public URI getBaseUri() {
URI uri = request.getUri();
try {
return new URI(uri.getScheme(), uri.getUserInfo(), uri.getHost(), uri.getPort(), "", uri.getQuery(), uri.getFragment());
} catch (URISyntaxException e) {
throw new IllegalArgumentException("Unexpected URI format: " + uri.toASCIIString(), e);
}
}
/**
* This operation is not supported currently,
* so {@link UnsupportedOperationException} is thrown for all invocations.
*
* @throws UnsupportedOperationException this operation is not supported currently.
*/
@Override
public UriBuilder getBaseUriBuilder() {
throw new UnsupportedOperationException();
}
/**
* This operation is not supported currently,
* so {@link UnsupportedOperationException} is thrown for all invocations.
*
* @throws UnsupportedOperationException this operation is not supported currently.
*/
@Override
public MultivaluedMap getPathParameters() {
throw new UnsupportedOperationException();
}
/**
* This operation is not supported currently,
* so {@link UnsupportedOperationException} is thrown for all invocations.
*
* @throws UnsupportedOperationException this operation is not supported currently.
*/
@Override
public MultivaluedMap getPathParameters(boolean decode) {
throw new UnsupportedOperationException();
}
@Override
public MultivaluedMap getQueryParameters() {
return getQueryParameters(true);
}
@Override
public MultivaluedMap getQueryParameters(boolean decode) {
MultivaluedMap map = new MultivaluedHashMap<>();
request.getParameters().forEach(
(str, vals) -> vals.forEach(
val -> map.add(string(str, decode), string(val, decode))));
return map;
}
/**
* This operation is not supported currently,
* so {@link UnsupportedOperationException} is thrown for all invocations.
*
* @throws UnsupportedOperationException this operation is not supported currently.
*/
@Override
public List getMatchedURIs() {
throw new UnsupportedOperationException();
}
/**
* This operation is not supported currently,
* so {@link UnsupportedOperationException} is thrown for all invocations.
*
* @throws UnsupportedOperationException this operation is not supported currently.
*/
@Override
public List getMatchedURIs(boolean decode) {
throw new UnsupportedOperationException();
}
/**
* This operation is not supported currently,
* so {@link UnsupportedOperationException} is thrown for all invocations.
*
* @throws UnsupportedOperationException this operation is not supported currently.
*/
@Override
public List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy