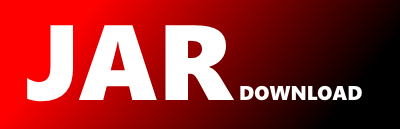
io.micronaut.configuration.kafka.KafkaMessage Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of micronaut-kafka Show documentation
Show all versions of micronaut-kafka Show documentation
Integration between Micronaut and Kafka Messaging
/*
* Copyright 2017-2021 original authors
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package io.micronaut.configuration.kafka;
import io.micronaut.core.annotation.NonNull;
import io.micronaut.core.annotation.Nullable;
import java.util.Map;
/**
* Message payload representation.
*
* @param The key type
* @param The value type
* @author Denis Stepanov
* @since 4.1.0
*/
public final class KafkaMessage {
private final String topic;
private final K key;
private final V body;
private final Integer partition;
private final Long timestamp;
private final Map headers;
/**
* The default constructor.
*
* @param topic The topic
* @param key The key
* @param body The body
* @param partition The partition
* @param timestamp The timestamp
* @param headers The headers
*/
public KafkaMessage(@Nullable String topic, @Nullable K key, @Nullable V body, @Nullable Integer partition,
@Nullable Long timestamp, @Nullable Map headers) {
this.topic = topic;
this.key = key;
this.body = body;
this.partition = partition;
this.timestamp = timestamp;
this.headers = headers;
}
@Nullable
public String getTopic() {
return topic;
}
@Nullable
public K getKey() {
return key;
}
@Nullable
public V getBody() {
return body;
}
@Nullable
public Integer getPartition() {
return partition;
}
@Nullable
public Long getTimestamp() {
return timestamp;
}
@Nullable
public Map getHeaders() {
return headers;
}
/**
* The message builder.
* @param The key type
* @param The value type
*/
public static final class Builder {
private String topic;
private K key;
private V body;
private Integer partition;
private Long timestamp;
private Map headers;
@NonNull
public static Builder withBody(@Nullable F body) {
Builder builder = new Builder<>();
builder.body = body;
return builder;
}
@NonNull
public static Builder withoutBody() {
Builder builder = new Builder<>();
return builder;
}
@NonNull
public Builder topic(@Nullable String topic) {
this.topic = topic;
return this;
}
@NonNull
public Builder key(@Nullable K key) {
this.key = key;
return this;
}
@NonNull
public Builder body(@Nullable V body) {
this.body = body;
return this;
}
@NonNull
public Builder header(@Nullable Map headers) {
this.headers = headers;
return this;
}
@NonNull
public Builder partition(@Nullable Integer partition) {
this.partition = partition;
return this;
}
@NonNull
public Builder timestamp(@Nullable Long timestamp) {
this.timestamp = timestamp;
return this;
}
@NonNull
public KafkaMessage build() {
return new KafkaMessage(topic, key, body, partition, timestamp, headers);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy