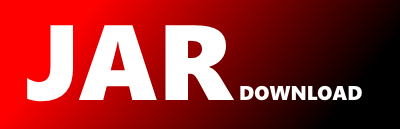
io.micronaut.kubernetes.KubernetesConfiguration Maven / Gradle / Ivy
/*
* Copyright 2017-2020 original authors
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package io.micronaut.kubernetes;
import java.util.Collection;
import java.util.Collections;
import java.util.HashSet;
import java.util.List;
import java.util.Map;
import io.micronaut.context.annotation.BootstrapContextCompatible;
import io.micronaut.context.annotation.ConfigurationProperties;
import io.micronaut.context.annotation.Requires;
import io.micronaut.context.env.Environment;
import io.micronaut.core.annotation.NonNull;
import io.micronaut.discovery.DiscoveryConfiguration;
import io.micronaut.kubernetes.client.NamespaceResolver;
/**
* Encapsulates constants for Kubernetes configuration.
*
* @author Sergio del Amo
* @author Álvaro Sánchez-Mariscal
* @since 1.0.0
*/
@Requires(env = Environment.KUBERNETES)
@ConfigurationProperties(KubernetesConfiguration.PREFIX)
@BootstrapContextCompatible
public class KubernetesConfiguration {
public static final String PREFIX = "kubernetes.client";
private String namespace;
private KubernetesDiscoveryConfiguration discovery = new KubernetesDiscoveryConfiguration();
private KubernetesSecretsConfiguration secrets = new KubernetesSecretsConfiguration();
private KubernetesConfigMapsConfiguration configMaps = new KubernetesConfigMapsConfiguration();
/**
* Default constructor.
*
* @param namespaceResolver namespace resolver
*/
public KubernetesConfiguration(NamespaceResolver namespaceResolver) {
this.namespace = namespaceResolver.resolveNamespace();
}
/**
* @return The {@link DiscoveryConfiguration}.
*/
@NonNull
public KubernetesDiscoveryConfiguration getDiscovery() {
return this.discovery;
}
/**
* @param discoveryConfiguration The discovery configuration
*/
public void setDiscovery(KubernetesDiscoveryConfiguration discoveryConfiguration) {
this.discovery = discoveryConfiguration;
}
/**
* @return the namespace
*/
@NonNull
public String getNamespace() {
return namespace;
}
/**
* @param namespace Sets the namespace.
*/
public void setNamespace(String namespace) {
this.namespace = namespace;
}
/**
* @return the {@link KubernetesSecretsConfiguration}.
*/
@NonNull
public KubernetesSecretsConfiguration getSecrets() {
return secrets;
}
/**
* @param secretsConfiguration the {@link KubernetesSecretsConfiguration}.
*/
public void setSecrets(KubernetesSecretsConfiguration secretsConfiguration) {
this.secrets = secretsConfiguration;
}
/**
* @return The config maps configuration properties
*/
@NonNull
public KubernetesConfigMapsConfiguration getConfigMaps() {
return configMaps;
}
/**
* @param configMapsConfiguration The config maps configuration properties
*/
public void setConfigMaps(KubernetesConfigMapsConfiguration configMapsConfiguration) {
this.configMaps = configMapsConfiguration;
}
@Override
public String toString() {
return "KubernetesConfiguration{" +
"namespace='" + namespace + '\'' +
", discovery=" + discovery +
", secrets=" + secrets +
", configMaps=" + configMaps +
'}';
}
/**
* Configuration class for the discovery client of Kubernetes.
*/
@ConfigurationProperties(DiscoveryConfiguration.PREFIX)
@BootstrapContextCompatible
public static class KubernetesDiscoveryConfiguration extends AbstractKubernetesConfiguration {
public static final String DEFAULT_MODE = "endpoint";
public static final String PREFIX = KubernetesConfiguration.PREFIX + "." + DiscoveryConfiguration.PREFIX;
private String mode = DEFAULT_MODE;
/**
* @return default service discovery mode
*/
public String getMode() {
return mode;
}
/**
* @param mode default service discovery mode
*/
public void setMode(String mode) {
this.mode = mode;
}
}
/**
* Base class for other configuration sub-classes.
*/
private abstract static class AbstractKubernetesConfiguration extends DiscoveryConfiguration {
private static final Boolean DEFAULT_EXCEPTION_ON_POD_LABELS_MISSING = false;
private Collection includes = new HashSet<>();
private Collection excludes = new HashSet<>();
private Map labels;
private List podLabels;
private boolean exceptionOnPodLabelsMissing = DEFAULT_EXCEPTION_ON_POD_LABELS_MISSING;
/**
* @return the names to include
*/
public Collection getIncludes() {
return includes;
}
/**
* @param includes the names to include
*/
public void setIncludes(Collection includes) {
this.includes = includes;
}
/**
* @return the names to exclude
*/
public Collection getExcludes() {
return excludes;
}
/**
* @param excludes the names to exclude
*/
public void setExcludes(Collection excludes) {
this.excludes = excludes;
}
/**
* @return labels to match
*/
public Map getLabels() {
if (labels == null) {
return Collections.emptyMap();
}
return labels;
}
/**
* @param labels labels to match
*/
public void setLabels(Map labels) {
this.labels = labels;
}
/**
* @return podLabels to match
*/
public List getPodLabels() {
if (podLabels == null) {
return Collections.emptyList();
}
return podLabels;
}
/**
* @param podLabels labels to match
*/
public void setPodLabels(List podLabels) {
this.podLabels = podLabels;
}
/**
* @return Flag to indicate that failure to find configured pod label is fatal (default {@link #DEFAULT_EXCEPTION_ON_POD_LABELS_MISSING}).
*/
public boolean isExceptionOnPodLabelsMissing() {
return exceptionOnPodLabelsMissing;
}
/**
* If set to true an exception will be thrown if at least one of the configured pod labels is not found
* among the application's pod labels.
* Default value ({@link #DEFAULT_EXCEPTION_ON_POD_LABELS_MISSING}).
*
* @param exceptionOnPodLabelsMissing flag to throw exception on pod labels missing
*/
public void setExceptionOnPodLabelsMissing(boolean exceptionOnPodLabelsMissing) {
this.exceptionOnPodLabelsMissing = exceptionOnPodLabelsMissing;
}
}
/**
* Base class for config-maps and secrets.
*/
public abstract static class AbstractConfigConfiguration extends AbstractKubernetesConfiguration {
private Collection paths;
private boolean useApi;
private boolean watch;
AbstractConfigConfiguration(boolean defaultWatch) {
watch = defaultWatch;
}
/**
* @return paths where secrets are mounted
*/
public Collection getPaths() {
if (paths == null) {
return Collections.emptySet();
}
return paths;
}
/**
* @param paths where secrets are mounted
*/
public void setPaths(Collection paths) {
this.paths = paths;
}
/**
* @return whether to use the API to read secrets when {@link #paths} is used.
*/
public boolean isUseApi() {
return useApi;
}
/**
* @param useApi whether to use the API to read secrets when {@link #paths} is used.
*/
public void setUseApi(boolean useApi) {
this.useApi = useApi;
}
/**
* @return whether to enable watching for the ConfigMap changes.
*/
public boolean isWatch() {
return watch;
}
/**
* @param watch flag to watch for the ConfigMap changes.
*/
public void setWatch(boolean watch) {
this.watch = watch;
}
}
/**
* Kubernetes secrets configuration properties.
*/
@ConfigurationProperties(KubernetesSecretsConfiguration.PREFIX)
@BootstrapContextCompatible
public static class KubernetesSecretsConfiguration extends AbstractConfigConfiguration {
static final String PREFIX = "secrets";
static final boolean DEFAULT_ENABLED = false;
static final boolean DEFAULT_WATCH = false;
private boolean enabled = DEFAULT_ENABLED;
public KubernetesSecretsConfiguration() {
super(DEFAULT_WATCH);
}
@Override
public boolean isEnabled() {
return enabled;
}
/**
* @param enabled enabled flag.
*/
public void setEnabled(boolean enabled) {
this.enabled = enabled;
}
}
/**
* Kubernetes config maps configuration properties.
*/
@ConfigurationProperties(KubernetesConfigMapsConfiguration.PREFIX)
@BootstrapContextCompatible
public static class KubernetesConfigMapsConfiguration extends AbstractConfigConfiguration {
public static final String PREFIX = "config-maps";
static final boolean DEFAULT_WATCH = true;
public KubernetesConfigMapsConfiguration() {
super(DEFAULT_WATCH);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy