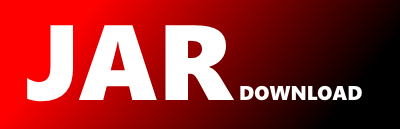
io.micronaut.core.bind.DefaultExecutableBinder Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of micronaut-core Show documentation
Show all versions of micronaut-core Show documentation
Core components supporting the Micronaut Framework
/*
* Copyright 2017-2020 original authors
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package io.micronaut.core.bind;
import io.micronaut.core.bind.exceptions.UnsatisfiedArgumentException;
import io.micronaut.core.convert.ArgumentConversionContext;
import io.micronaut.core.convert.ConversionContext;
import io.micronaut.core.convert.ConversionError;
import io.micronaut.core.convert.exceptions.ConversionErrorException;
import io.micronaut.core.type.Argument;
import io.micronaut.core.type.Executable;
import io.micronaut.core.annotation.Nullable;
import java.util.*;
/**
* Default implementation of the {@link ExecutableBinder} interface.
*
* @param The source type
*/
public class DefaultExecutableBinder implements ExecutableBinder {
private final Map, Object> preBound;
/**
* Default constructor.
*/
public DefaultExecutableBinder() {
this.preBound = Collections.emptyMap();
}
/**
* A map of pre-bound values for any arguments that don't need to be dynamically bound.
*
* @param preBound The pre bound values
*/
public DefaultExecutableBinder(@Nullable Map, Object> preBound) {
this.preBound = preBound == null ? Collections.emptyMap() : preBound;
}
@Override
public BoundExecutable bind(
Executable target,
ArgumentBinderRegistry registry,
S source) throws UnsatisfiedArgumentException {
Argument[] arguments = target.getArguments();
Object[] boundArguments = new Object[arguments.length];
for (int i = 0; i < arguments.length; i++) {
Argument> argument = arguments[i];
if (preBound.containsKey(argument)) {
boundArguments[i] = preBound.get(argument);
} else {
Optional extends ArgumentBinder, S>> argumentBinder = registry.findArgumentBinder(argument);
if (argumentBinder.isPresent()) {
ArgumentBinder, S> binder = argumentBinder.get();
ArgumentConversionContext conversionContext = ConversionContext.of(argument);
ArgumentBinder.BindingResult> bindingResult = binder.bind(
conversionContext,
source
);
if (!bindingResult.isPresentAndSatisfied()) {
if (argument.isNullable()) {
boundArguments[i] = null;
} else {
final Optional lastError = conversionContext.getLastError();
if (lastError.isPresent()) {
throw new ConversionErrorException(argument, lastError.get());
} else {
throw new UnsatisfiedArgumentException(argument);
}
}
} else {
boundArguments[i] = bindingResult.get();
}
} else {
throw new UnsatisfiedArgumentException(argument);
}
}
}
return new BoundExecutable<>() {
@Override
public Executable getTarget() {
return target;
}
@Override
public R invoke(T instance) {
return target.invoke(instance, getBoundArguments());
}
@Override
public Object[] getBoundArguments() {
return boundArguments;
}
};
}
@Override
public BoundExecutable tryBind(Executable target, ArgumentBinderRegistry registry, S source) {
Argument[] arguments = target.getArguments();
Object[] boundArguments = new Object[arguments.length];
List> unbound = new ArrayList<>(arguments.length);
for (int i = 0; i < arguments.length; i++) {
Argument> argument = arguments[i];
if (preBound.containsKey(argument)) {
boundArguments[i] = preBound.get(argument);
} else {
Optional extends ArgumentBinder, S>> argumentBinder = registry.findArgumentBinder(argument);
if (argumentBinder.isPresent()) {
ArgumentBinder, S> binder = argumentBinder.get();
ArgumentConversionContext conversionContext = ConversionContext.of(argument);
ArgumentBinder.BindingResult> bindingResult = binder.bind(
conversionContext,
source
);
if (!bindingResult.isPresentAndSatisfied()) {
if (argument.isNullable()) {
boundArguments[i] = null;
} else {
boundArguments[i] = null;
unbound.add(argument);
}
} else {
boundArguments[i] = bindingResult.get();
}
} else {
boundArguments[i] = null;
unbound.add(argument);
}
}
}
return new BoundExecutable<>() {
@Override
public List> getUnboundArguments() {
return Collections.unmodifiableList(unbound);
}
@Override
public Executable getTarget() {
return target;
}
@Override
public R invoke(T instance) {
return target.invoke(instance, getBoundArguments());
}
@Override
public Object[] getBoundArguments() {
return boundArguments;
}
};
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy