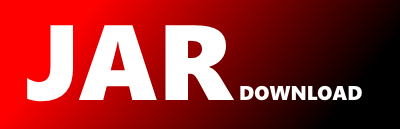
io.micronaut.core.convert.ConversionContext Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of micronaut-core Show documentation
Show all versions of micronaut-core Show documentation
Core components supporting the Micronaut Framework
/*
* Copyright 2017-2020 original authors
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package io.micronaut.core.convert;
import io.micronaut.core.annotation.AnnotationMetadataProvider;
import io.micronaut.core.type.Argument;
import io.micronaut.core.type.TypeVariableResolver;
import io.micronaut.core.util.ArgumentUtils;
import io.micronaut.core.util.ArrayUtils;
import io.micronaut.core.annotation.Nullable;
import java.lang.annotation.Annotation;
import java.nio.charset.Charset;
import java.nio.charset.StandardCharsets;
import java.util.*;
/**
* A conversion context is a context object supplied to a {@link TypeConverter} that allows more accurate conversion.
*
* @author Graeme Rocher
* @since 1.0
*/
public interface ConversionContext extends AnnotationMetadataProvider, TypeVariableResolver, ErrorsContext {
/**
* The default conversion context.
*/
ConversionContext DEFAULT = new ConversionContext() {
};
/**
* Constant for Boolean argument.
*/
ArgumentConversionContext BOOLEAN = ImmutableArgumentConversionContext.of(Argument.BOOLEAN);
/**
* Constant for Integer argument.
*/
ArgumentConversionContext INT = ImmutableArgumentConversionContext.of(Argument.INT);
/**
* Constant for Long argument.
*/
ArgumentConversionContext LONG = ImmutableArgumentConversionContext.of(Argument.LONG);
/**
* Constant for String argument.
*/
ArgumentConversionContext STRING = ImmutableArgumentConversionContext.of(Argument.STRING);
/**
* Constant for {@code List} argument.
*/
ArgumentConversionContext> LIST_OF_STRING = ImmutableArgumentConversionContext.of(Argument.LIST_OF_STRING);
/**
* Constant for {@code List} argument.
*/
ArgumentConversionContext
© 2015 - 2025 Weber Informatics LLC | Privacy Policy