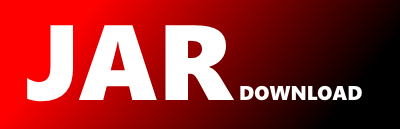
io.micronaut.http.tck.HttpResponseAssertion Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of micronaut-http-tck Show documentation
Show all versions of micronaut-http-tck Show documentation
Core components supporting the Micronaut Framework
/*
* Copyright 2017-2022 original authors
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package io.micronaut.http.tck;
import io.micronaut.core.annotation.Experimental;
import io.micronaut.core.annotation.NonNull;
import io.micronaut.core.annotation.Nullable;
import io.micronaut.http.HttpResponse;
import io.micronaut.http.HttpStatus;
import java.util.HashMap;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.Consumer;
/**
* Utility class to verify assertions given an HTTP Response.
* @author Sergio del Amo
* @since 3.8.0
*/
@Experimental
public final class HttpResponseAssertion {
private final HttpStatus httpStatus;
private final Map headers;
private final BodyAssertion, ?> bodyAssertion;
@Nullable
private final Consumer> responseConsumer;
private HttpResponseAssertion(HttpStatus httpStatus,
Map headers,
BodyAssertion, ?> bodyAssertion,
@Nullable Consumer> responseConsumer) {
this.httpStatus = httpStatus;
this.headers = headers;
this.bodyAssertion = bodyAssertion;
this.responseConsumer = responseConsumer;
}
@NonNull
public Optional>> getResponseConsumer() {
return Optional.ofNullable(responseConsumer);
}
/**
*
* @return Expected HTTP Response Status
*/
public HttpStatus getHttpStatus() {
return httpStatus;
}
/**
*
* @return Expected HTTP Response Headers
*/
public Map getHeaders() {
return headers;
}
/**
*
* @return Expected HTTP Response body
*/
public BodyAssertion, ?> getBody() {
return bodyAssertion;
}
/**
*
* @return Creates an instance of {@link HttpResponseAssertion.Builder}.
*/
public static HttpResponseAssertion.Builder builder() {
return new HttpResponseAssertion.Builder();
}
/**
* HTTP Response Assertion Builder.
*/
public static class Builder {
private HttpStatus httpStatus;
private Map headers;
private BodyAssertion, ?> bodyAssertion;
private Consumer> responseConsumer;
/**
*
* @param responseConsumer HTTP Response Consumer
* @return HTTP Response Assertion Builder
*/
public Builder assertResponse(Consumer> responseConsumer) {
this.responseConsumer = responseConsumer;
return this;
}
/**
*
* @param headers HTTP Headers
* @return HTTP Response Assertion Builder
*/
public Builder headers(Map headers) {
this.headers = headers;
return this;
}
/**
*
* @param headerName Header Name
* @param headerValue Header Value
* @return HTTP Response Assertion Builder
*/
public Builder header(String headerName, String headerValue) {
if (this.headers == null) {
this.headers = new HashMap<>();
}
this.headers.put(headerName, headerValue);
return this;
}
/**
*
* @param containsBody Response Body
* @return HTTP Response Assertion Builder
*/
public Builder body(String containsBody) {
this.bodyAssertion = BodyAssertion.builder().body(containsBody).contains();
return this;
}
/**
*
* @param bodyAssertion Response Body Assertion
* @return HTTP Response Assertion Builder
*/
public Builder body(BodyAssertion, ?> bodyAssertion) {
this.bodyAssertion = bodyAssertion;
return this;
}
/**
*
* @param httpStatus Response's HTTP Status
* @return HTTP Response Assertion Builder
*/
public Builder status(HttpStatus httpStatus) {
this.httpStatus = httpStatus;
return this;
}
/**
*
* @return HTTP Response Assertion
*/
public HttpResponseAssertion build() {
return new HttpResponseAssertion(Objects.requireNonNull(httpStatus), headers, bodyAssertion, responseConsumer);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy