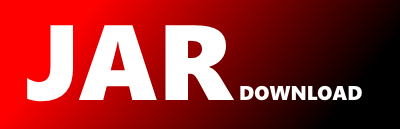
io.micronaut.kotlin.processing.visitor.KotlinMethodElement.kt Maven / Gradle / Ivy
/*
* Copyright 2017-2022 original authors
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package io.micronaut.kotlin.processing.visitor
import com.google.devtools.ksp.*
import com.google.devtools.ksp.symbol.*
import io.micronaut.inject.ast.*
import io.micronaut.inject.ast.annotation.ElementAnnotationMetadataFactory
@OptIn(KspExperimental::class)
internal open class KotlinMethodElement(
owningType: ClassElement,
val declaration: KSFunctionDeclaration,
private val presetParameters: List?,
elementAnnotationMetadataFactory: ElementAnnotationMetadataFactory,
visitorContext: KotlinVisitorContext
) : AbstractKotlinMethodElement(
KotlinMethodNativeElement(declaration),
visitorContext.resolver.getJvmName(declaration)!!,
owningType,
elementAnnotationMetadataFactory,
visitorContext
), MethodElement {
constructor(
owningType: ClassElement,
declaration: KSFunctionDeclaration,
elementAnnotationMetadataFactory: ElementAnnotationMetadataFactory,
visitorContext: KotlinVisitorContext
) : this(
owningType,
declaration,
null,
elementAnnotationMetadataFactory,
visitorContext
)
override val internalDeclaringType: ClassElement by lazy {
resolveDeclaringType(declaration, owningType)
}
override val internalDeclaredTypeArguments: Map by lazy {
resolveTypeArguments(nativeType, declaration, declaringType.typeArguments)
}
override val resolvedParameters: List by lazy {
presetParameters
?: declaration.parameters.map {
KotlinParameterElement(
null,
this,
it,
elementAnnotationMetadataFactory,
visitorContext
)
}
}
override val internalReturnType: ClassElement by lazy {
newClassElement(nativeType, declaration.returnType!!.resolve(), emptyMap())
}
override val internalGenericReturnType: ClassElement by lazy {
newClassElement(nativeType, declaration.returnType!!.resolve(), declaringType.typeArguments)
}
override fun isAbstract(): Boolean = declaration.isAbstract
override fun isPublic(): Boolean = declaration.isPublic()
override fun isProtected(): Boolean = declaration.isProtected()
override fun isPrivate(): Boolean = declaration.isPrivate()
override fun isSynthetic() =
declaration.functionKind != FunctionKind.MEMBER && declaration.functionKind != FunctionKind.STATIC
override fun isSuspend() = declaration.modifiers.contains(Modifier.SUSPEND)
override fun withNewOwningType(owningType: ClassElement): MethodElement {
val newMethod = KotlinMethodElement(
owningType,
declaration,
presetParameters,
elementAnnotationMetadataFactory,
visitorContext,
)
copyValues(newMethod)
return newMethod
}
override fun copyThis(): KotlinMethodElement {
return KotlinMethodElement(
owningType,
declaration,
presetParameters,
elementAnnotationMetadataFactory,
visitorContext,
)
}
override fun withParameters(vararg newParameters: ParameterElement) =
KotlinMethodElement(
owningType,
declaration,
newParameters.toList(),
elementAnnotationMetadataFactory,
visitorContext,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy