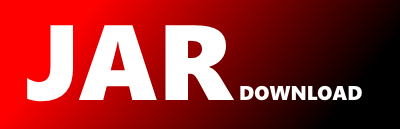
io.micronaut.inject.ast.ElementQuery Maven / Gradle / Ivy
/*
* Copyright 2017-2020 original authors
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package io.micronaut.inject.ast;
import io.micronaut.core.annotation.NonNull;
import io.micronaut.core.annotation.AnnotationMetadata;
import java.util.List;
import java.util.Objects;
import java.util.Set;
import java.util.function.Predicate;
/**
* An interface for querying the AST for elements.
*
* @param The element kind
* @since 2.3.0
* @author graemerocher
*/
public interface ElementQuery {
/**
* Constant to retrieve all fields.
*/
ElementQuery ALL_FIELDS = ElementQuery.of(FieldElement.class);
/**
* Constant to retrieve all methods.
*/
ElementQuery ALL_METHODS = ElementQuery.of(MethodElement.class);
/**
* Indicates that only declared members should be returned and not members from parent classes.
*
* @return This query
*/
@NonNull ElementQuery onlyDeclared();
/**
* Indicates that only concrete members should be returned.
*
* @return This query
*/
@NonNull ElementQuery onlyConcrete();
/**
* Indicates that only abstract members should be returned.
*
* @return This query
*/
@NonNull ElementQuery onlyAbstract();
/**
* Indicates that only accessible members should be returned. Inaccessible members include:
*
*
* - package/private members that are in a different package
* - private members
* - synthetic members or those whose names start with the dollar symbol
*
*
* @return This query
*/
@NonNull ElementQuery onlyAccessible();
/**
* Indicates to return only instance (non-static methods).
* @return The query
*/
ElementQuery onlyInstance();
/**
* Allows filtering elements by name.
* @param predicate The predicate to use. Should return true to include the element.
* @return This query
*/
@NonNull ElementQuery named(@NonNull Predicate predicate);
/**
* Allows filtering elements by annotation.
* @param predicate The predicate to use. Should return true to include the element.
* @return This query
*/
@NonNull ElementQuery annotated(@NonNull Predicate predicate);
/**
* Allows filtering by modifiers.
* @param predicate The predicate to use. Should return true to include the element.
* @return This query
*/
@NonNull ElementQuery modifiers(@NonNull Predicate> predicate);
/**
* A final filter that allows access to the materialized Element. This method should be used
* as a last resort as it is less efficient than the other filtration methods.
* @param predicate The predicate to use. Should return true to include the element.
* @return This query
*/
@NonNull ElementQuery filter(@NonNull Predicate predicate);
/**
* Build the query result.
*
* @return The query result.
*/
@NonNull Result result();
/**
* Create a new {@link ElementQuery} for the given element type.
* @param elementType The element type
* @param The element generic type
* @return The query
*/
static @NonNull ElementQuery of(@NonNull Class elementType) {
return new DefaultElementQuery<>(
Objects.requireNonNull(elementType, "Element type cannot be null")
);
}
/**
* Result interface when building a query.
* @param The element type.
*/
interface Result {
/**
* @return Whether to return only abstract methods
*/
boolean isOnlyAbstract();
/**
* @return Whether to return only concrete methods
*/
boolean isOnlyConcrete();
/**
* @return The element type
*/
@NonNull Class getElementType();
/**
* @return Whether to return only accessible members
*/
boolean isOnlyAccessible();
/**
* @return Whether to declare only declared members
*/
boolean isOnlyDeclared();
/**
* @return Whether to return only instance methods
*/
boolean isOnlyInstance();
/**
* @return The name predicates
*/
@NonNull List> getNamePredicates();
/**
* @return The annotation predicates
*/
@NonNull List> getAnnotationPredicates();
/**
* @return The modifier predicate
*/
@NonNull List>> getModifierPredicates();
/**
* @return The element predicates
*/
@NonNull List> getElementPredicates();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy