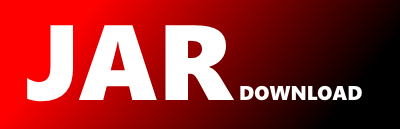
io.milton.common.StringSplitUtils Maven / Gradle / Ivy
The newest version!
/*
* Licensed to the Apache Software Foundation (ASF) under one
* or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. The ASF licenses this file
* to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
*/
package io.milton.common;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
/**
* Utility helper for splitting the strings.
*
* @author brad
*/
public class StringSplitUtils {
//~ Static fields/initializers =====================================================================================
private static final String[] EMPTY_STRING_ARRAY = new String[0];
//~ Constructors ===================================================================================================
private StringSplitUtils() {
}
//~ Methods ========================================================================================================
/**
* Splits a String
at the first instance of the delimiter.Does not include the delimiter in
* the response.
*
* @param toSplit the string to split
* @param delimiter to split the string up with
* @return a two element array with index 0 being before the delimiter, and index 1 being after the delimiter
* (neither element includes the delimiter)
* @throws IllegalArgumentException if an argument was invalid
*/
public static String[] split(String toSplit, String delimiter) {
if (delimiter.length() != 1) {
throw new IllegalArgumentException(
"Delimiter can only be one character in length");
}
int offset = toSplit.indexOf(delimiter);
if (offset < 0) {
return null;
}
String beforeDelimiter = toSplit.substring(0, offset);
String afterDelimiter = toSplit.substring(offset + 1);
return new String[]{beforeDelimiter, afterDelimiter};
}
/**
* Takes an array of String
s, and for each element removes any instances of
* removeCharacter
, and splits the element based on the delimiter
. A Map
is
* then generated, with the left of the delimiter providing the key, and the right of the delimiter providing the
* value.Will trim both the key and value before adding to the Map
.
*
* @param array the array to process
* @param delimiter to split each element using (typically the equals symbol)
* @param removeCharacters one or more characters to remove from each element prior to attempting the split
* operation (typically the quotation mark symbol) or null
if no removal should occur
* @return a Map
representing the array contents, or null
if the array to process was
* null or empty
*/
public static Map splitEachArrayElementAndCreateMap(String[] array,
String delimiter, String removeCharacters) {
if ((array == null) || (array.length == 0)) {
return null;
}
Map map = new HashMap<>();
for (String element : array) {
String postRemove;
if (removeCharacters == null) {
postRemove = element;
} else {
postRemove = replace(element, removeCharacters, "");
}
String[] splitThisArrayElement = split(postRemove, delimiter);
if (splitThisArrayElement == null) {
continue;
}
map.put(splitThisArrayElement[0].trim(),
splitThisArrayElement[1].trim());
}
return map;
}
/**
* Replace all occurrences of a substring within a string with
* another string.
*
* @param inString String to examine
* @param oldPattern String to replace
* @param newPattern String to insert
* @return a String with the replacements
*/
public static String replace(String inString, String oldPattern, String newPattern) {
if (inString == null) {
return null;
}
if (oldPattern == null || newPattern == null) {
return inString;
}
StringBuilder sbuf = new StringBuilder();
int pos = 0;//ourpositionintheoldstring
int index = inString.indexOf(oldPattern);
int patLen = oldPattern.length();
while (index >= 0) {
sbuf.append(inString, pos, index);
sbuf.append(newPattern);
pos = index + patLen;
index = inString.indexOf(oldPattern, pos);
}
sbuf.append(inString.substring(pos));
return sbuf.toString();
}
public static String substringBeforeLast(String str,
String separator) {
if (str == null || separator == null || str.isEmpty()
|| separator.isEmpty()) {
return str;
}
int pos = str.lastIndexOf(separator);
if (pos == -1) {
return str;
}
return str.substring(0, pos);
}
public static String substringAfterLast(String str, String separator) {
if (str == null || str.isEmpty()) {
return str;
}
if (separator == null || separator.isEmpty()) {
return "";
}
int pos = str.lastIndexOf(separator);
if (pos == -1 || pos == (str.length() - separator.length())) {
return "";
}
return str.substring(pos + separator.length());
}
/**
* Splits a given string on the given separator character, skips the contents of quoted substrings
* when looking for separators.
* Introduced for use in DigestProcessingFilter (see SEC-506).
*
* This was copied and modified from commons-lang StringUtils
*/
public static String[] splitIgnoringQuotes(String str,
char separatorChar) {
if (str == null) {
return null;
}
int len = str.length();
if (len == 0) {
return EMPTY_STRING_ARRAY;
}
List list = new ArrayList<>();
int i = 0;
int start = 0;
boolean match = false;
while (i < len) {
if (str.charAt(i) == '"') {
i++;
while (i < len) {
if (str.charAt(i) == '"') {
i++;
break;
}
i++;
}
match = true;
continue;
}
if (str.charAt(i) == separatorChar) {
if (match) {
list.add(str.substring(start, i));
match = false;
}
start = ++i;
continue;
}
match = true;
i++;
}
if (match) {
list.add(str.substring(start, i));
}
return list.toArray(new String[0]);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy