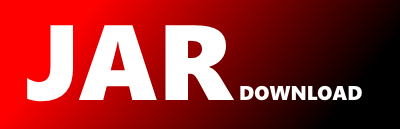
io.milton.http.http11.SimpleContentGenerator Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of milton-server-ce Show documentation
Show all versions of milton-server-ce Show documentation
Milton Community Edition: Supports DAV level 1 and is available on Apache2 license
The newest version!
/*
* Licensed to the Apache Software Foundation (ASF) under one
* or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. The ASF licenses this file
* to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
*/
package io.milton.http.http11;
import io.milton.http.Request;
import io.milton.http.Response;
import io.milton.http.Response.Status;
import io.milton.resource.Resource;
import java.io.OutputStream;
import org.apache.commons.lang.StringEscapeUtils;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
/**
* Just uses simple property values to generate error content
*
* @author brad
*/
public class SimpleContentGenerator implements ContentGenerator {
private static final Logger log = LoggerFactory.getLogger(SimpleContentGenerator.class);
private String methodNotAllowed = "Method Not Allowed
";
private String notFound = "${url} Not Found (404)
";
private String methodNotImplemented = "Method Not Implemented
";
private String conflict = "Conflict
";
private String serverError = "Server Error
";
private String unauthorised = "Not authorised
";
private String loginExternal = "Not authorised
Please login with: ${externalProviders}
";
private String unknown = "Unknown error
";
public SimpleContentGenerator() {
}
@Override
public void generate(Resource resource, Request request, Response response, Status status) {
String template;
switch (status) {
case SC_METHOD_NOT_ALLOWED:
template = getMethodNotAllowed();
break;
case SC_NOT_FOUND:
template = getNotFound();
break;
case SC_NOT_IMPLEMENTED:
template = getMethodNotImplemented();
break;
case SC_CONFLICT:
template = getConflict();
break;
case SC_INTERNAL_SERVER_ERROR:
template = getServerError();
break;
case SC_UNAUTHORIZED:
template = getUnauthorised();
break;
default:
template = getUnknown();
}
final String finalTemplate = applyTemplates(template, request);
response.setEntity((response1, outputStream) -> {
outputStream.write(finalTemplate.getBytes("UTF-8"));
outputStream.flush();
});
}
private String applyTemplates(String template, Request request) {
template = template.replace("${url}", StringEscapeUtils.escapeHtml(request.getAbsolutePath()));
return template;
}
/**
* @return the methodNotAllowed
*/
public String getMethodNotAllowed() {
return methodNotAllowed;
}
/**
* @param methodNotAllowed the methodNotAllowed to set
*/
public void setMethodNotAllowed(String methodNotAllowed) {
this.methodNotAllowed = methodNotAllowed;
}
/**
* @return the notFound
*/
public String getNotFound() {
return notFound;
}
/**
* @param notFound the notFound to set
*/
public void setNotFound(String notFound) {
this.notFound = notFound;
}
/**
* @return the methodNotImplemented
*/
public String getMethodNotImplemented() {
return methodNotImplemented;
}
/**
* @param methodNotImplemented the methodNotImplemented to set
*/
public void setMethodNotImplemented(String methodNotImplemented) {
this.methodNotImplemented = methodNotImplemented;
}
/**
* @return the conflict
*/
public String getConflict() {
return conflict;
}
/**
* @param conflict the conflict to set
*/
public void setConflict(String conflict) {
this.conflict = conflict;
}
/**
* @return the serverError
*/
public String getServerError() {
return serverError;
}
/**
* @param serverError the serverError to set
*/
public void setServerError(String serverError) {
this.serverError = serverError;
}
/**
* @return the unauthorised
*/
public String getUnauthorised() {
return unauthorised;
}
/**
* @param unauthorised the unauthorised to set
*/
public void setUnauthorised(String unauthorised) {
this.unauthorised = unauthorised;
}
/**
* @return the unknown
*/
public String getUnknown() {
return unknown;
}
/**
* @param unknown the unknown to set
*/
public void setUnknown(String unknown) {
this.unknown = unknown;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy