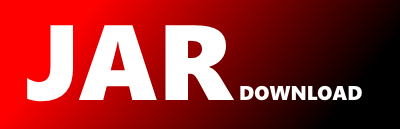
io.milvus.v2.service.collection.request.CreateCollectionReq Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of milvus-sdk-java Show documentation
Show all versions of milvus-sdk-java Show documentation
Java SDK for Milvus, a distributed high-performance vector database.
/*
* Licensed to the Apache Software Foundation (ASF) under one
* or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. The ASF licenses this file
* to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
*/
package io.milvus.v2.service.collection.request;
import io.milvus.v2.common.ConsistencyLevel;
import io.milvus.v2.common.DataType;
import io.milvus.v2.common.IndexParam;
import io.milvus.v2.exception.ErrorCode;
import io.milvus.v2.exception.MilvusClientException;
import lombok.Builder;
import lombok.Data;
import lombok.NonNull;
import lombok.experimental.SuperBuilder;
import java.util.ArrayList;
import java.util.List;
@Data
@SuperBuilder
public class CreateCollectionReq {
@NonNull
private String collectionName;
@Builder.Default
private String description = "";
private Integer dimension;
@Builder.Default
private String primaryFieldName = "id";
@Builder.Default
private DataType idType = DataType.Int64;
@Builder.Default
private Integer maxLength = 65535;
@Builder.Default
private String vectorFieldName = "vector";
@Builder.Default
private String metricType = IndexParam.MetricType.COSINE.name();
@Builder.Default
private Boolean autoID = Boolean.FALSE;
// used by quickly create collections and create collections with schema
// Note: This property is only for fast creating collection. If user use CollectionSchema to create a collection,
// the CollectionSchema.enableDynamicField must equal to CreateCollectionReq.enableDynamicField.
@Builder.Default
private Boolean enableDynamicField = Boolean.TRUE;
@Builder.Default
private Integer numShards = 1;
// create collections with schema
private CollectionSchema collectionSchema;
@Builder.Default
private List indexParams = new ArrayList<>();
//private String partitionKeyField;
private Integer numPartitions;
@Builder.Default
private ConsistencyLevel consistencyLevel = ConsistencyLevel.BOUNDED;
public static abstract class CreateCollectionReqBuilder> {
public B indexParam(IndexParam indexParam) {
try {
this.indexParams$value.add(indexParam);
}catch (UnsupportedOperationException _e){
this.indexParams$value = new ArrayList<>(this.indexParams$value);
this.indexParams$value.add(indexParam);
}
this.indexParams$set = true;
return self();
}
public B enableDynamicField(Boolean enableDynamicField) {
if (this.collectionSchema != null && (this.collectionSchema.isEnableDynamicField() != enableDynamicField)) {
throw new MilvusClientException(ErrorCode.INVALID_PARAMS,
"The enableDynamicField flag has been set by CollectionSchema, not allow to set different value by enableDynamicField().");
}
this.enableDynamicField$value = enableDynamicField;
this.enableDynamicField$set = true;
return self();
}
public B collectionSchema(CollectionSchema collectionSchema) {
if (this.enableDynamicField$set && (collectionSchema.isEnableDynamicField() != this.enableDynamicField$value)) {
throw new MilvusClientException(ErrorCode.INVALID_PARAMS,
"The enableDynamicField flag has been set by enableDynamicField(), not allow to set different value by collectionSchema.");
}
this.collectionSchema = collectionSchema;
this.enableDynamicField$value = collectionSchema.isEnableDynamicField();
this.enableDynamicField$set = true;
return self();
}
}
@Data
@SuperBuilder
public static class CollectionSchema {
@Builder.Default
private List fieldSchemaList = new ArrayList<>();
@Builder.Default
private boolean enableDynamicField = false;
public CollectionSchema addField(AddFieldReq addFieldReq) {
CreateCollectionReq.FieldSchema fieldSchema = FieldSchema.builder()
.name(addFieldReq.getFieldName())
.dataType(addFieldReq.getDataType())
.description(addFieldReq.getDescription())
.isPrimaryKey(addFieldReq.getIsPrimaryKey())
.isPartitionKey(addFieldReq.getIsPartitionKey())
.isClusteringKey(addFieldReq.getIsClusteringKey())
.autoID(addFieldReq.getAutoID())
.build();
if (addFieldReq.getDataType().equals(DataType.Array)) {
if (addFieldReq.getElementType() == null) {
throw new MilvusClientException(ErrorCode.INVALID_PARAMS, "Element type, maxCapacity are required for array field");
}
fieldSchema.setElementType(addFieldReq.getElementType());
fieldSchema.setMaxCapacity(addFieldReq.getMaxCapacity());
} else if (addFieldReq.getDataType().equals(DataType.VarChar)) {
fieldSchema.setMaxLength(addFieldReq.getMaxLength());
} else if (addFieldReq.getDataType().equals(DataType.FloatVector) || addFieldReq.getDataType().equals(DataType.BinaryVector) ||
addFieldReq.getDataType().equals(DataType.Float16Vector) || addFieldReq.getDataType().equals(DataType.BFloat16Vector)) {
if (addFieldReq.getDimension() == null) {
throw new MilvusClientException(ErrorCode.INVALID_PARAMS, "Dimension is required for vector field");
}
fieldSchema.setDimension(addFieldReq.getDimension());
}
fieldSchemaList.add(fieldSchema);
return this;
}
public CreateCollectionReq.FieldSchema getField(String fieldName) {
for (CreateCollectionReq.FieldSchema field : fieldSchemaList) {
if (field.getName().equals(fieldName)) {
return field;
}
}
return null;
}
}
@Data
@SuperBuilder
public static class FieldSchema {
private String name;
@Builder.Default
private String description = "";
private DataType dataType;
@Builder.Default
private Integer maxLength = 65535;
private Integer dimension;
@Builder.Default
private Boolean isPrimaryKey = Boolean.FALSE;
@Builder.Default
private Boolean isPartitionKey = Boolean.FALSE;
@Builder.Default
private Boolean isClusteringKey = Boolean.FALSE;
@Builder.Default
private Boolean autoID = Boolean.FALSE;
private DataType elementType;
private Integer maxCapacity;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy