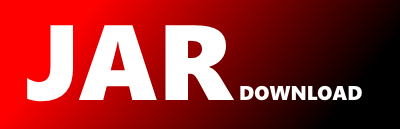
io.milvus.bulkwriter.BulkWriter Maven / Gradle / Ivy
/*
* Licensed to the Apache Software Foundation (ASF) under one
* or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. The ASF licenses this file
* to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
*/
package io.milvus.bulkwriter;
import com.google.gson.*;
import io.milvus.bulkwriter.common.clientenum.BulkFileType;
import io.milvus.bulkwriter.common.clientenum.TypeSize;
import io.milvus.common.utils.ExceptionUtils;
import io.milvus.grpc.*;
import io.milvus.param.ParamUtils;
import io.milvus.param.collection.CollectionSchemaParam;
import io.milvus.param.collection.FieldType;
import org.apache.commons.collections4.CollectionUtils;
import org.apache.commons.lang3.tuple.Pair;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import java.io.IOException;
import java.nio.ByteBuffer;
import java.util.*;
import java.util.concurrent.locks.ReentrantLock;
import static io.milvus.param.Constant.DYNAMIC_FIELD_NAME;
public abstract class BulkWriter {
private static final Logger logger = LoggerFactory.getLogger(BulkWriter.class);
protected CollectionSchemaParam collectionSchema;
protected int chunkSize;
protected BulkFileType fileType;
protected int bufferSize;
protected int bufferRowCount;
protected int totalRowCount;
protected Buffer buffer;
protected ReentrantLock bufferLock;
protected BulkWriter(CollectionSchemaParam collectionSchema, int chunkSize, BulkFileType fileType) {
this.collectionSchema = collectionSchema;
this.chunkSize = chunkSize;
this.fileType = fileType;
if (CollectionUtils.isEmpty(collectionSchema.getFieldTypes())) {
ExceptionUtils.throwUnExpectedException("collection schema fields list is empty");
}
if (!hasPrimaryField(collectionSchema.getFieldTypes())) {
ExceptionUtils.throwUnExpectedException("primary field is null");
}
bufferLock = new ReentrantLock();
buffer = null;
this.newBuffer();
}
protected Integer getBufferSize() {
return bufferSize;
}
public Integer getBufferRowCount() {
return bufferRowCount;
}
public Integer getTotalRowCount() {
return totalRowCount;
}
protected Integer getChunkSize() {
return chunkSize;
}
protected Buffer newBuffer() {
Buffer oldBuffer = buffer;
bufferLock.lock();
this.buffer = new Buffer(collectionSchema, fileType);
bufferLock.unlock();
return oldBuffer;
}
public void appendRow(JsonObject row) throws IOException, InterruptedException {
Map rowValues = verifyRow(row);
bufferLock.lock();
buffer.appendRow(rowValues);
bufferLock.unlock();
}
protected void commit(boolean async) throws InterruptedException {
bufferLock.lock();
bufferSize = 0;
bufferRowCount = 0;
bufferLock.unlock();
}
protected String getDataPath() {
return "";
}
private Map verifyRow(JsonObject row) {
int rowSize = 0;
Map rowValues = new HashMap<>();
for (FieldType fieldType : collectionSchema.getFieldTypes()) {
String fieldName = fieldType.getName();
if (fieldType.isPrimaryKey() && fieldType.isAutoID()) {
if (row.has(fieldName)) {
String msg = String.format("The primary key field '%s' is auto-id, no need to provide", fieldName);
ExceptionUtils.throwUnExpectedException(msg);
} else {
continue;
}
}
if (!row.has(fieldName)) {
String msg = String.format("The field '%s' is missed in the row", fieldName);
ExceptionUtils.throwUnExpectedException(msg);
}
JsonElement obj = row.get(fieldName);
if (obj == null || obj.isJsonNull()) {
String msg = String.format("Illegal value for field '%s', value is null", fieldName);
ExceptionUtils.throwUnExpectedException(msg);
}
DataType dataType = fieldType.getDataType();
switch (dataType) {
case BinaryVector:
case FloatVector:
case Float16Vector:
case BFloat16Vector:
case SparseFloatVector: {
Pair
© 2015 - 2025 Weber Informatics LLC | Privacy Policy