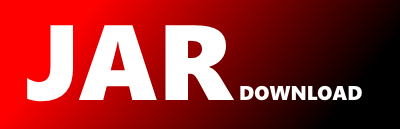
io.milvus.v2.utils.VectorUtils Maven / Gradle / Ivy
/*
* Licensed to the Apache Software Foundation (ASF) under one
* or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. The ASF licenses this file
* to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
*/
package io.milvus.v2.utils;
import com.google.protobuf.ByteString;
import io.milvus.common.utils.GTsDict;
import io.milvus.common.utils.JsonUtils;
import io.milvus.v2.common.ConsistencyLevel;
import io.milvus.exception.ParamException;
import io.milvus.grpc.*;
import io.milvus.param.Constant;
import io.milvus.param.ParamUtils;
import io.milvus.v2.service.vector.request.*;
import io.milvus.v2.service.vector.request.ranker.BaseRanker;
import io.milvus.v2.service.vector.request.data.*;
import lombok.NonNull;
import org.apache.commons.collections4.CollectionUtils;
import org.apache.commons.lang3.StringUtils;
import java.util.*;
public class VectorUtils {
public QueryRequest ConvertToGrpcQueryRequest(QueryReq request){
QueryRequest.Builder builder = QueryRequest.newBuilder()
.setCollectionName(request.getCollectionName())
.addAllPartitionNames(request.getPartitionNames())
.addAllOutputFields(request.getOutputFields())
.setExpr(request.getFilter());
// a new parameter from v2.2.9, if user didn't specify consistency level, set this parameter to true
if (request.getConsistencyLevel() == null) {
builder.setUseDefaultConsistency(true);
} else {
builder.setConsistencyLevelValue(request.getConsistencyLevel().getCode());
}
// set offset and limit value.
// directly pass the two values, the server will verify them.
long offset = request.getOffset();
if (offset > 0) {
builder.addQueryParams(KeyValuePair.newBuilder()
.setKey(Constant.OFFSET)
.setValue(String.valueOf(offset))
.build());
}
long limit = request.getLimit();
if (limit > 0) {
builder.addQueryParams(KeyValuePair.newBuilder()
.setKey(Constant.LIMIT)
.setValue(String.valueOf(limit))
.build());
}
// ignore growing
// builder.addQueryParams(KeyValuePair.newBuilder()
// .setKey(Constant.IGNORE_GROWING)
// .setValue(String.valueOf(request.isIgnoreGrowing()))
// .build());
return builder.build();
}
private static long getGuaranteeTimestamp(ConsistencyLevel consistencyLevel, String collectionName){
if(consistencyLevel == null){
Long ts = GTsDict.getInstance().getCollectionTs(collectionName);
return (ts == null) ? 1L : ts;
}
switch (consistencyLevel){
case STRONG:
return 0L;
case SESSION:
Long ts = GTsDict.getInstance().getCollectionTs(collectionName);
return (ts == null) ? 1L : ts;
case BOUNDED:
return 2L; // let server side to determine the bounded time
default:
return 1L; // EVENTUALLY and others
}
}
public SearchRequest ConvertToGrpcSearchRequest(SearchReq request) {
SearchRequest.Builder builder = SearchRequest.newBuilder()
.setCollectionName(request.getCollectionName());
if (!request.getPartitionNames().isEmpty()) {
request.getPartitionNames().forEach(builder::addPartitionNames);
}
// prepare target vectors
List vectors = request.getData();
if (vectors.isEmpty()) {
throw new ParamException("Target vectors list of search request is empty.");
}
PlaceholderType plType = vectors.get(0).getPlaceholderType();
List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy