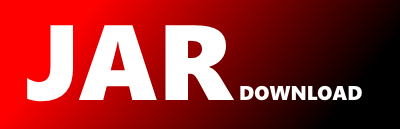
io.mindmaps.graph.internal.ElementFactory Maven / Gradle / Ivy
/*
* MindmapsDB - A Distributed Semantic Database
* Copyright (C) 2016 Mindmaps Research Ltd
*
* MindmapsDB is free software: you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* MindmapsDB is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with MindmapsDB. If not, see .
*/
package io.mindmaps.graph.internal;
import io.mindmaps.concept.EntityType;
import io.mindmaps.concept.RelationType;
import io.mindmaps.concept.ResourceType;
import io.mindmaps.concept.RoleType;
import io.mindmaps.concept.RuleType;
import io.mindmaps.concept.Type;
import io.mindmaps.util.Schema;
import org.apache.tinkerpop.gremlin.structure.Vertex;
/**
* Internal factory to produce different types of concepts
*/
final class ElementFactory {
private final AbstractMindmapsGraph mindmapsGraph;
public ElementFactory(AbstractMindmapsGraph mindmapsGraph){
this.mindmapsGraph = mindmapsGraph;
}
public RelationImpl buildRelation(Vertex v, RelationType type){
return new RelationImpl(v, type, mindmapsGraph);
}
public CastingImpl buildCasting(Vertex v, RoleType type){
return new CastingImpl(v, type, mindmapsGraph);
}
public TypeImpl buildConceptType(Vertex v, Type type){
return new TypeImpl(v, type, mindmapsGraph);
}
public RuleTypeImpl buildRuleType(Vertex v, Type type){
return new RuleTypeImpl(v, type, mindmapsGraph);
}
public RoleTypeImpl buildRoleType(Vertex v, Type type){
return new RoleTypeImpl(v, type, mindmapsGraph);
}
public ResourceTypeImpl buildResourceType(Vertex v, Type type){
return new ResourceTypeImpl<>(v, type, mindmapsGraph);
}
public ResourceTypeImpl buildResourceType(Vertex v, Type type, ResourceType.DataType dataType){
return new ResourceTypeImpl<>(v, type, mindmapsGraph, dataType);
}
public RelationTypeImpl buildRelationType(Vertex v, Type type){
return new RelationTypeImpl(v, type, mindmapsGraph);
}
public EntityTypeImpl buildEntityType(Vertex v, Type type){
return new EntityTypeImpl(v, type, mindmapsGraph);
}
public EntityImpl buildEntity(Vertex v, EntityType type){
return new EntityImpl(v, type, mindmapsGraph);
}
public ResourceImpl buildResource(Vertex v, ResourceType type){
return new ResourceImpl<>(v, type, mindmapsGraph);
}
public ResourceImpl buildResource(Vertex v, ResourceType type, V value){
return new ResourceImpl<>(v, type, mindmapsGraph, value);
}
public RuleImpl buildRule(Vertex v, RuleType type){
return buildRule(v, type, v.value(Schema.ConceptProperty.RULE_LHS.name()), v.value(Schema.ConceptProperty.RULE_RHS.name()));
}
public RuleImpl buildRule(Vertex v, RuleType type, String lhs, String rhs){
return new RuleImpl(v, type, mindmapsGraph, lhs, rhs);
}
/**
*
* @param v A vertex of an unknown type
* @return A concept built to the correct type
*/
public ConceptImpl buildUnknownConcept(Vertex v){
if(!v.property(Schema.ConceptProperty.BASE_TYPE.name()).isPresent()){
return null;
}
Schema.BaseType type = Schema.BaseType.valueOf(v.value(Schema.ConceptProperty.BASE_TYPE.name()));
ConceptImpl concept = null;
//All these types are null because at this stage the concept has been defined so we don't need to know the type.
switch (type){
case RELATION:
concept = buildRelation(v, null);
break;
case CASTING:
concept = buildCasting(v, null);
break;
case TYPE:
concept = buildConceptType(v, null);
break;
case ROLE_TYPE:
concept = buildRoleType(v, null);
break;
case RELATION_TYPE:
concept = buildRelationType(v, null);
break;
case ENTITY:
concept = buildEntity(v, null);
break;
case ENTITY_TYPE:
concept = buildEntityType(v, null);
break;
case RESOURCE_TYPE:
concept = buildResourceType(v, null);
break;
case RESOURCE:
concept = buildResource(v, null);
break;
case RULE:
concept = buildRule(v, null);
break;
case RULE_TYPE:
concept = buildRuleType(v, null);
break;
}
return concept;
}
public TypeImpl buildSpecificConceptType(Vertex vertex, Type type){
Schema.BaseType baseType = Schema.BaseType.valueOf(vertex.value(Schema.ConceptProperty.BASE_TYPE.name()));
TypeImpl conceptType;
switch (baseType){
case ROLE_TYPE:
conceptType = buildRoleType(vertex, type);
break;
case RELATION_TYPE:
conceptType = buildRelationType(vertex, type);
break;
case RESOURCE_TYPE:
conceptType = buildResourceType(vertex, type);
break;
case RULE_TYPE:
conceptType = buildRuleType(vertex, type);
break;
case ENTITY_TYPE:
conceptType = buildEntityType(vertex, type);
break;
default:
conceptType = buildConceptType(vertex, type);
}
return conceptType;
}
public EdgeImpl buildEdge(org.apache.tinkerpop.gremlin.structure.Edge edge, AbstractMindmapsGraph mindmapsGraph){
return new EdgeImpl(edge, mindmapsGraph);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy