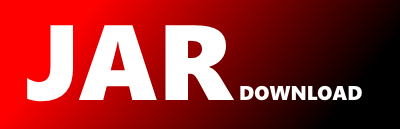
commonMain.io.mockk.GatewayAPI.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of mockk-dsl-jvm Show documentation
Show all versions of mockk-dsl-jvm Show documentation
Java MockK DSL providing API for MockK implementation
The newest version!
package io.mockk
import kotlin.reflect.KClass
/**
* Mediates mocking implementation
*/
interface MockKGateway {
val mockFactory: MockFactory
val staticMockFactory: StaticMockFactory
val objectMockFactory: ObjectMockFactory
val constructorMockFactory: ConstructorMockFactory
val stubber: Stubber
val verifier: Verifier
val excluder: Excluder
val callRecorder: CallRecorder
val instanceFactoryRegistry: InstanceFactoryRegistry
val clearer: Clearer
val mockInitializer: MockInitializer
val verificationAcknowledger: VerificationAcknowledger
val mockTypeChecker: MockTypeChecker
fun verifier(params: VerificationParameters): CallVerifier
data class ClearOptions(
val answers: Boolean,
val recordedCalls: Boolean,
val childMocks: Boolean,
val verificationMarks: Boolean,
val exclusionRules: Boolean
)
companion object {
lateinit var implementation: () -> MockKGateway
}
/**
* Create new mocks or spies
*/
interface MockFactory {
fun mockk(
mockType: KClass,
name: String?,
relaxed: Boolean,
moreInterfaces: Array>,
relaxUnitFun: Boolean
): T
fun spyk(
mockType: KClass?,
objToCopy: T?,
name: String?,
moreInterfaces: Array>,
recordPrivateCalls: Boolean
): T
fun temporaryMock(mockType: KClass<*>): Any
fun isMock(value: Any): Boolean
}
/**
* Binds static mocks
*/
interface StaticMockFactory {
fun staticMockk(cls: KClass<*>): () -> Unit
fun clear(type: KClass<*>, options: ClearOptions)
fun clearAll(options: ClearOptions, currentThreadOnly: Boolean)
}
/**
* Binds object mocks
*/
interface ObjectMockFactory {
fun objectMockk(obj: Any, recordPrivateCalls: Boolean): () -> Unit
fun clear(obj: Any, options: ClearOptions)
fun clearAll(options: ClearOptions, currentThreadOnly: Boolean)
}
/**
* Controls constructor mocking
*/
interface ConstructorMockFactory {
fun constructorMockk(
cls: KClass<*>,
recordPrivateCalls: Boolean,
localToThread: Boolean
): () -> Unit
fun mockPlaceholder(cls: KClass, args: Array>? = null): T
fun clear(type: KClass<*>, options: ClearOptions)
fun clearAll(options: ClearOptions, currentThreadOnly: Boolean)
}
/**
* Clears mocks
*/
interface Clearer {
fun clear(
mocks: Array,
options: ClearOptions
)
fun clearAll(
options: ClearOptions,
currentThreadOnly: Boolean
)
}
/**
* Stub calls
*/
interface Stubber {
fun every(
mockBlock: (MockKMatcherScope.() -> T)?,
coMockBlock: (suspend MockKMatcherScope.() -> T)?
): MockKStubScope
}
/**
* Verify calls
*/
interface Verifier {
fun verify(
params: VerificationParameters,
mockBlock: (MockKVerificationScope.() -> Unit)?,
coMockBlock: (suspend MockKVerificationScope.() -> Unit)?
)
}
/**
* Verify calls
*/
interface Excluder {
fun exclude(
params: ExclusionParameters,
mockBlock: (MockKMatcherScope.() -> Unit)?,
coMockBlock: (suspend MockKMatcherScope.() -> Unit)?
)
}
/**
* Parameters of verification
*/
data class VerificationParameters(
val ordering: Ordering,
val min: Int,
val max: Int,
val inverse: Boolean,
val timeout: Long
)
/**
* Parameters of exclusion
*/
data class ExclusionParameters(
val current: Boolean
)
interface AnswerOpportunity {
fun provideAnswer(answer: Answer)
}
/**
* Builds a list of calls
*/
interface CallRecorder {
val calls: List
fun startStubbing()
fun startVerification(params: VerificationParameters)
fun startExclusion(params: ExclusionParameters)
fun round(n: Int, total: Int = 64)
fun matcher(matcher: Matcher<*>, cls: KClass): T
fun call(invocation: Invocation): Any?
fun answerOpportunity(): AnswerOpportunity<*>
fun done()
fun hintNextReturnType(cls: KClass<*>, n: Int)
fun reset()
fun estimateCallRounds(): Int
fun nCalls(): Int
fun wasNotCalled(list: List)
fun discardLastCallRound()
fun isLastCallReturnsNothing(): Boolean
}
/**
* Verifier takes the list of calls and checks what invocations happened to the mocks
*/
interface CallVerifier {
fun verify(verificationSequence: List, params: VerificationParameters): VerificationResult
fun captureArguments()
}
/**
* Result of verification
*/
sealed class VerificationResult {
data class OK(val verifiedCalls: List) : VerificationResult()
data class Failure(val message: String) : VerificationResult()
val matches: Boolean
get() = this is OK
}
interface InstanceFactoryRegistry {
fun registerFactory(factory: InstanceFactory)
fun deregisterFactory(factory: InstanceFactory)
}
/**
* Factory of dummy objects
*/
interface InstanceFactory {
fun instantiate(cls: KClass<*>): Any?
}
interface MockInitializer {
fun initAnnotatedMocks(
targets: List,
overrideRecordPrivateCalls: Boolean,
relaxUnitFun: Boolean,
relaxed: Boolean
)
}
interface VerificationAcknowledger {
fun markCallVerified(invocation: Invocation)
fun acknowledgeVerified(mock: Any)
fun acknowledgeVerified()
fun checkUnnecessaryStub(mock: Any)
fun checkUnnecessaryStub()
}
interface MockTypeChecker {
fun isRegularMock(mock: Any): Boolean
fun isSpy(mock: Any): Boolean
fun isObjectMock(mock: Any): Boolean
fun isStaticMock(mock: Any): Boolean
fun isConstructorMock(mock: Any): Boolean
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy