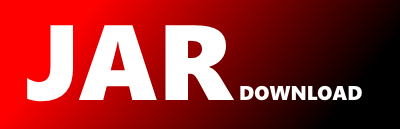
io.mosip.testrig.apirig.testscripts.DeleteWithParam Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of apitest-masterdata Show documentation
Show all versions of apitest-masterdata Show documentation
Parent project of MOSIP Admin Services apitests
The newest version!
package io.mosip.testrig.apirig.testscripts;
import java.lang.reflect.Field;
import java.util.ArrayList;
import java.util.List;
import java.util.Map;
import org.apache.log4j.Level;
import org.apache.log4j.Logger;
import org.json.JSONObject;
import org.testng.ITest;
import org.testng.ITestContext;
import org.testng.ITestResult;
import org.testng.Reporter;
import org.testng.SkipException;
import org.testng.annotations.AfterMethod;
import org.testng.annotations.BeforeClass;
import org.testng.annotations.DataProvider;
import org.testng.annotations.Test;
import org.testng.internal.BaseTestMethod;
import org.testng.internal.TestResult;
import io.mosip.testrig.apirig.dto.OutputValidationDto;
import io.mosip.testrig.apirig.dto.TestCaseDTO;
import io.mosip.testrig.apirig.testrunner.HealthChecker;
import io.mosip.testrig.apirig.utils.AdminTestException;
import io.mosip.testrig.apirig.utils.AdminTestUtil;
import io.mosip.testrig.apirig.utils.AuthenticationTestException;
import io.mosip.testrig.apirig.utils.GlobalConstants;
import io.mosip.testrig.apirig.utils.MasterDataConfigManager;
import io.mosip.testrig.apirig.utils.MasterDataUtil;
import io.mosip.testrig.apirig.utils.OutputValidationUtil;
import io.mosip.testrig.apirig.utils.ReportUtil;
import io.restassured.response.Response;
public class DeleteWithParam extends AdminTestUtil implements ITest {
private static final Logger logger = Logger.getLogger(DeleteWithParam.class);
protected String testCaseName = "";
public Response response = null;
@BeforeClass
public static void setLogLevel() {
if (MasterDataConfigManager.IsDebugEnabled())
logger.setLevel(Level.ALL);
else
logger.setLevel(Level.ERROR);
}
/**
* get current testcaseName
*/
@Override
public String getTestName() {
return testCaseName;
}
/**
* Data provider class provides test case list
*
* @return object of data provider
*/
@DataProvider(name = "testcaselist")
public Object[] getTestCaseList(ITestContext context) {
String ymlFile = context.getCurrentXmlTest().getLocalParameters().get("ymlFile");
logger.info("Started executing yml: " + ymlFile);
return getYmlTestData(ymlFile);
}
/**
* Test method for OTP Generation execution
*
* @param objTestParameters
* @param testScenario
* @param testcaseName
* @throws AuthenticationTestException
* @throws AdminTestException
*/
@Test(dataProvider = "testcaselist")
public void test(TestCaseDTO testCaseDTO) throws AuthenticationTestException, AdminTestException {
testCaseName = testCaseDTO.getTestCaseName();
testCaseName = MasterDataUtil.isTestCaseValidForExecution(testCaseDTO);
testCaseName = isTestCaseValidForExecution(testCaseDTO);
if (HealthChecker.signalTerminateExecution) {
throw new SkipException(
GlobalConstants.TARGET_ENV_HEALTH_CHECK_FAILED + HealthChecker.healthCheckFailureMapS);
}
String[] templateFields = testCaseDTO.getTemplateFields();
if (testCaseDTO.getTemplateFields() != null && templateFields.length > 0) {
ArrayList inputtestCases = AdminTestUtil.getInputTestCase(testCaseDTO);
ArrayList outputtestcase = AdminTestUtil.getOutputTestCase(testCaseDTO);
for (int i = 0; i < languageList.size(); i++) {
response = deleteWithPathParamAndCookie(ApplnURI + testCaseDTO.getEndPoint(),
getJsonFromTemplate(inputtestCases.get(i).toString(), testCaseDTO.getInputTemplate()),
COOKIENAME, testCaseDTO.getRole(), testCaseDTO.getTestCaseName());
Map> ouputValid = OutputValidationUtil.doJsonOutputValidation(
response.asString(),
getJsonFromTemplate(outputtestcase.get(i).toString(), testCaseDTO.getOutputTemplate()),
testCaseDTO, response.getStatusCode());
Reporter.log(ReportUtil.getOutputValidationReport(ouputValid));
if (!OutputValidationUtil.publishOutputResult(ouputValid))
throw new AdminTestException("Failed at output validation");
}
}
else {
response = deleteWithPathParamAndCookie(ApplnURI + testCaseDTO.getEndPoint(),
getJsonFromTemplate(testCaseDTO.getInput(), testCaseDTO.getInputTemplate()), COOKIENAME,
testCaseDTO.getRole(), testCaseDTO.getTestCaseName());
Map> ouputValid = OutputValidationUtil.doJsonOutputValidation(
response.asString(), getJsonFromTemplate(testCaseDTO.getOutput(), testCaseDTO.getOutputTemplate()),
testCaseDTO, response.getStatusCode());
Reporter.log(ReportUtil.getOutputValidationReport(ouputValid));
if (!OutputValidationUtil.publishOutputResult(ouputValid))
throw new AdminTestException("Failed at output validation");
}
}
/**
* The method ser current test name to result
*
* @param result
*/
@AfterMethod(alwaysRun = true)
public void setResultTestName(ITestResult result) {
try {
Field method = TestResult.class.getDeclaredField("m_method");
method.setAccessible(true);
method.set(result, result.getMethod().clone());
BaseTestMethod baseTestMethod = (BaseTestMethod) result.getMethod();
Field f = baseTestMethod.getClass().getSuperclass().getDeclaredField("m_methodName");
f.setAccessible(true);
f.set(baseTestMethod, testCaseName);
} catch (Exception e) {
Reporter.log("Exception : " + e.getMessage());
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy