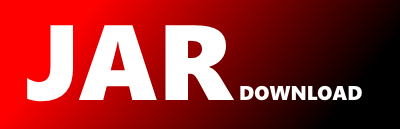
io.mosip.authentication.common.service.impl.AuthAnonymousProfileServiceImpl Maven / Gradle / Ivy
package io.mosip.authentication.common.service.impl;
import static io.mosip.authentication.core.constant.IdAuthCommonConstants.BIOMETRICS;
import static io.mosip.authentication.core.constant.IdAuthCommonConstants.BIO_SUB_TYPE;
import static io.mosip.authentication.core.constant.IdAuthCommonConstants.BIO_TYPE;
import static io.mosip.authentication.core.constant.IdAuthCommonConstants.DEFAULT_DOB_PATTERN;
import static io.mosip.authentication.core.constant.IdAuthCommonConstants.DIGITAL_ID;
import static io.mosip.authentication.core.constant.IdAuthCommonConstants.FAILURE;
import static io.mosip.authentication.core.constant.IdAuthCommonConstants.IDA;
import static io.mosip.authentication.core.constant.IdAuthCommonConstants.QUALITY_SCORE;
import static io.mosip.authentication.core.constant.IdAuthCommonConstants.REQUEST;
import static io.mosip.authentication.core.constant.IdAuthCommonConstants.SUCCESS;
import static io.mosip.authentication.core.constant.IdAuthConfigKeyConstants.MOSIP_DATE_OF_BIRTH_PATTERN;
import java.time.LocalDateTime;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Date;
import java.util.List;
import java.util.Map;
import java.util.Map.Entry;
import java.util.Optional;
import java.util.UUID;
import java.util.stream.Collectors;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.beans.factory.annotation.Value;
import org.springframework.context.annotation.Lazy;
import org.springframework.stereotype.Service;
import com.fasterxml.jackson.core.JsonProcessingException;
import com.fasterxml.jackson.databind.ObjectMapper;
import io.mosip.authentication.common.service.entity.AnonymousProfileEntity;
import io.mosip.authentication.common.service.entity.AutnTxn;
import io.mosip.authentication.common.service.helper.IdInfoHelper;
import io.mosip.authentication.common.service.impl.idevent.AnonymousAuthenticationProfile;
import io.mosip.authentication.common.service.impl.idevent.BiometricProfileInfo;
import io.mosip.authentication.common.service.impl.match.DemoMatchType;
import io.mosip.authentication.common.service.repository.AuthAnonymousProfileRepository;
import io.mosip.authentication.common.service.util.EnvUtil;
import io.mosip.authentication.common.service.websub.impl.AuthAnonymousEventPublisher;
import io.mosip.authentication.core.constant.IdAuthCommonConstants;
import io.mosip.authentication.core.constant.IdAuthConfigKeyConstants;
import io.mosip.authentication.core.exception.IdAuthenticationBusinessException;
import io.mosip.authentication.core.indauth.dto.AuthError;
import io.mosip.authentication.core.indauth.dto.IdentityInfoDTO;
import io.mosip.authentication.core.logger.IdaLogger;
import io.mosip.authentication.core.partner.dto.PartnerDTO;
import io.mosip.authentication.core.spi.indauth.match.MatchType;
import io.mosip.authentication.core.spi.profile.AuthAnonymousProfileService;
import io.mosip.kernel.core.exception.ExceptionUtils;
import io.mosip.kernel.core.logger.spi.Logger;
import io.mosip.kernel.core.util.DateUtils;
/**
*
* @author Loganathan Sekar
*
*/
@Service
@Lazy
public class AuthAnonymousProfileServiceImpl implements AuthAnonymousProfileService {
private Logger logger = IdaLogger.getLogger(AuthAnonymousProfileServiceImpl.class);
@Autowired
private IdInfoHelper idInfoHelper;
@Autowired
private AuthAnonymousEventPublisher authAnonymousEventPublisher;
@Autowired
private AuthAnonymousProfileRepository authAnonymousProfileRepository;
@Value("${" + IdAuthConfigKeyConstants.DATE_TIME_PATTERN + "}")
private String dateTimePattern;
@Value("${" + IdAuthConfigKeyConstants.PREFERRED_LANG_ATTRIB_NAME + "}")
private String preferredLangAttribName;
@Value("${" + IdAuthConfigKeyConstants.LOCATION_PROFILE_ATTRIB_NAME + "}")
private String locationProfileAttribName;
@Value("${" + MOSIP_DATE_OF_BIRTH_PATTERN + ":" + DEFAULT_DOB_PATTERN + "}")
private String dateOfBirthPattern;
@Autowired
private ObjectMapper mapper;
@Override
public void storeAnonymousProfile(Map requestBody, Map requestMetadata,
Map responseMetadata, boolean status, List errors) {
AnonymousAuthenticationProfile ananymousProfile = createAnonymousProfile(requestBody, requestMetadata, responseMetadata, status, errors);
storeAnonymousProfile(ananymousProfile);
authAnonymousEventPublisher.publishEvent(ananymousProfile);
}
private void storeAnonymousProfile(AnonymousAuthenticationProfile ananymousProfile) {
AnonymousProfileEntity authAnonymousProfileEntity = new AnonymousProfileEntity();
String id = UUID.randomUUID().toString();
authAnonymousProfileEntity.setId(id);
authAnonymousProfileEntity.setCrBy(IDA);
LocalDateTime crDTimes = DateUtils.getUTCCurrentDateTime();
authAnonymousProfileEntity.setCrDTimes(crDTimes);
try {
authAnonymousProfileEntity.setProfile(mapper.writeValueAsString(ananymousProfile));
} catch (JsonProcessingException e) {
logger.error("Error saving anonymous profile. %s", ExceptionUtils.getStackTrace(e));
}
authAnonymousProfileRepository.save(authAnonymousProfileEntity);
authAnonymousProfileRepository.flush();
}
private AnonymousAuthenticationProfile createAnonymousProfile(Map requestBody,
Map requestMetadata, Map responseMetadata, boolean status,
List errorCodes) {
AnonymousAuthenticationProfile ananymousProfile = new AnonymousAuthenticationProfile();
Map> idInfo = getMapOfIdentityInfoDTOList(responseMetadata);
if(idInfo != null && !idInfo.isEmpty()) {
setYearOfBirth(ananymousProfile, idInfo);
String preferredLang = idInfoHelper.getDynamicEntityInfoAsString(idInfo, null, preferredLangAttribName);
if(preferredLang != null) {
ananymousProfile.setPreferredLanguages(List.of(preferredLang));
}
String langCode = getProfileDataLangCode(idInfo, preferredLang);
if(langCode != null) {
setGender(ananymousProfile, idInfo, langCode);
try {
Map locationInfo = idInfoHelper.getIdEntityInfoMap(DemoMatchType.DYNAMIC, idInfo, langCode, locationProfileAttribName);
ananymousProfile.setLocation(new ArrayList<>(locationInfo.values()));
} catch (IdAuthenticationBusinessException e) {
logger.error("Error fetching %s for anonymous profile: %s", locationProfileAttribName, ExceptionUtils.getStackTrace(e));
}
}
List biometricInfos = getBiometricInfos(requestBody);
ananymousProfile.setBiometricInfo(biometricInfos);
}
setAuthFactors(responseMetadata, ananymousProfile);
setDate(ananymousProfile);
setErrorCodes(errorCodes, ananymousProfile);
setPartnerName(requestMetadata, ananymousProfile);
setStatus(status, ananymousProfile);
return ananymousProfile;
}
@SuppressWarnings("unchecked")
private List getBiometricInfos(Map requestBody) {
Object requestObj = requestBody.get(REQUEST);
if(requestObj instanceof Map) {
Object biometricsObj = ((Map) requestObj).get(BIOMETRICS);
if(biometricsObj instanceof List) {
return ((List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy