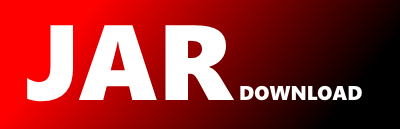
io.mosip.authentication.common.service.impl.DemoAuthServiceImpl Maven / Gradle / Ivy
package io.mosip.authentication.common.service.impl;
import java.util.List;
import java.util.Map;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import io.mosip.authentication.common.service.builder.AuthStatusInfoBuilder;
import io.mosip.authentication.common.service.builder.MatchInputBuilder;
import io.mosip.authentication.common.service.config.IDAMappingConfig;
import io.mosip.authentication.common.service.helper.IdInfoHelper;
import io.mosip.authentication.common.service.impl.match.DemoAuthType;
import io.mosip.authentication.common.service.impl.match.DemoMatchType;
import io.mosip.authentication.core.constant.IdAuthenticationErrorConstants;
import io.mosip.authentication.core.exception.IdAuthenticationBusinessException;
import io.mosip.authentication.core.indauth.dto.AuthRequestDTO;
import io.mosip.authentication.core.indauth.dto.AuthStatusInfo;
import io.mosip.authentication.core.indauth.dto.IdentityInfoDTO;
import io.mosip.authentication.core.spi.indauth.match.MatchInput;
import io.mosip.authentication.core.spi.indauth.match.MatchOutput;
import io.mosip.authentication.core.spi.indauth.service.DemoAuthService;
/**
* The implementation of Demographic Authentication service.
*
* @author Arun Bose
*/
@Service
public class DemoAuthServiceImpl implements DemoAuthService {
/** The id info helper. */
@Autowired
public IdInfoHelper idInfoHelper;
/** The id info helper. */
@Autowired
public MatchInputBuilder matchInputBuilder;
/** The ida mapping config. */
@Autowired
IDAMappingConfig idaMappingConfig;
/**
* Gets the match output.
*
* @param listMatchInputs the list match inputs
* @param authRequestDTO the demo DTO
* @param demoEntity the demo entity
* @param partnerId the partner id
* @return the match output
* @throws IdAuthenticationBusinessException the id authentication business
* exception
*/
public List getMatchOutput(List listMatchInputs, AuthRequestDTO authRequestDTO,
Map> demoEntity, String partnerId) throws IdAuthenticationBusinessException {
return idInfoHelper.matchIdentityData(authRequestDTO, demoEntity, listMatchInputs, partnerId);
}
/*
* (non-Javadoc)
*
* @see io.mosip.authentication.core.spi.indauth.service.DemoAuthService#
* getDemoStatus(io.mosip.authentication.core.dto.indauth.AuthRequestDTO)
*/
public AuthStatusInfo authenticate(AuthRequestDTO authRequestDTO, String token,
Map> demoEntity, String partnerId) throws IdAuthenticationBusinessException {
if (demoEntity == null || demoEntity.isEmpty()) {
throw new IdAuthenticationBusinessException(IdAuthenticationErrorConstants.SERVER_ERROR);
}
List listMatchInputs = constructMatchInput(authRequestDTO, demoEntity);
List listMatchOutputs = getMatchOutput(listMatchInputs, authRequestDTO, demoEntity, partnerId);
// Using AND condition on the match output for Bio auth.
boolean demoMatched = !listMatchOutputs.isEmpty() && listMatchOutputs.stream().allMatch(MatchOutput::isMatched);
return AuthStatusInfoBuilder.buildStatusInfo(demoMatched, listMatchInputs, listMatchOutputs,
DemoAuthType.values(), idaMappingConfig);
}
/**
* Construct match input.
*
* @param authRequestDTO the auth request DTO
* @return the list
*/
public List constructMatchInput(AuthRequestDTO authRequestDTO,
Map> idInfo) {
return matchInputBuilder.buildMatchInput(authRequestDTO, DemoAuthType.values(), DemoMatchType.values(),
idInfo);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy