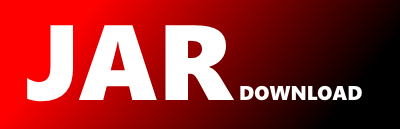
io.mosip.authentication.common.service.impl.match.AgeMatchingStrategy Maven / Gradle / Ivy
package io.mosip.authentication.common.service.impl.match;
import java.util.Map;
import io.mosip.authentication.core.constant.IdAuthCommonConstants;
import io.mosip.authentication.core.constant.IdAuthenticationErrorConstants;
import io.mosip.authentication.core.exception.IdAuthenticationBusinessException;
import io.mosip.authentication.core.logger.IdaLogger;
import io.mosip.authentication.core.spi.indauth.match.MatchFunction;
import io.mosip.authentication.core.spi.indauth.match.MatchingStrategyType;
import io.mosip.authentication.core.spi.indauth.match.TextMatchingStrategy;
import io.mosip.authentication.core.util.DemoMatcherUtil;
import io.mosip.kernel.core.exception.ExceptionUtils;
import io.mosip.kernel.core.logger.spi.Logger;
/**
* The Enum AgeMatchingStrategy - used to compare and
* evaluate the AGE value received from the request and entity
*
* @author Sanjay Murali
* @author Nagarjuna
*/
public enum AgeMatchingStrategy implements TextMatchingStrategy {
/** The exact. */
EXACT(MatchingStrategyType.EXACT, (Object reqInfo, Object entityInfo, Map props) -> {
try {
int reqAge = Integer.parseInt(String.valueOf(reqInfo));
int entityAge = Integer.parseInt(String.valueOf(entityInfo));
return getDemoMatcherUtilObject(props).doLessThanEqualToMatch(reqAge, entityAge);
} catch (NumberFormatException e) {
logError(e);
throw new IdAuthenticationBusinessException(IdAuthenticationErrorConstants.DATA_VALIDATION_FAILED, e);
}
});
/** The match function. */
private final MatchFunction matchFunction;
/** The match strategy type. */
private final MatchingStrategyType matchStrategyType;
/** The mosipLogger. */
private static Logger mosipLogger = IdaLogger.getLogger(AgeMatchingStrategy.class);
/** The Constant AGE Matching strategy. */
private static final String TYPE = "AgeMatchingStrategy";
/**
* Instantiates a new age matching strategy.
*
* @param matchStrategyType the match strategy type
* @param matchFunction the match function
*/
AgeMatchingStrategy(MatchingStrategyType matchStrategyType, MatchFunction matchFunction) {
this.matchFunction = matchFunction;
this.matchStrategyType = matchStrategyType;
}
/**
* Log error.
*
* @param e the e
*/
private static void logError(NumberFormatException e) {
mosipLogger.error(IdAuthCommonConstants.SESSION_ID, TYPE, "Inside AgeMathing Strategy", ExceptionUtils.getStackTrace(e));
}
/*
* (non-Javadoc)
*
* @see
* io.mosip.authentication.service.impl.indauth.service.demo.MatchingStrategy#
* getType()
*/
@Override
public MatchingStrategyType getType() {
return matchStrategyType;
}
/*
* (non-Javadoc)
*
* @see
* io.mosip.authentication.service.impl.indauth.service.demo.MatchingStrategy#
* getMatchFunction()
*/
@Override
public MatchFunction getMatchFunction() {
return matchFunction;
}
/**
* Gets the demoMatcherUtil object
* @param props
* @return
*/
public static DemoMatcherUtil getDemoMatcherUtilObject(Map props) {
return (DemoMatcherUtil)props.get("demoMatcherUtil");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy