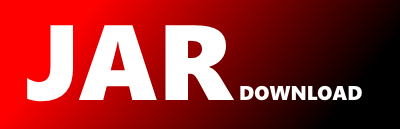
io.mosip.authentication.common.service.impl.match.FingerPrintMatchingStrategy Maven / Gradle / Ivy
package io.mosip.authentication.common.service.impl.match;
import java.util.Map;
import io.mosip.authentication.core.constant.IdAuthCommonConstants;
import io.mosip.authentication.core.constant.IdAuthenticationErrorConstants;
import io.mosip.authentication.core.exception.IdAuthenticationBusinessException;
import io.mosip.authentication.core.logger.IdaLogger;
import io.mosip.authentication.core.spi.indauth.match.TriFunctionWithBusinessException;
import io.mosip.authentication.core.spi.indauth.match.MatchFunction;
import io.mosip.authentication.core.spi.indauth.match.MatchingStrategy;
import io.mosip.authentication.core.spi.indauth.match.MatchingStrategyType;
import io.mosip.kernel.core.logger.spi.Logger;
/**
*
* Matching Strategy for Fingerprint
*
* @author Dinesh Karuppiah.T
*/
public enum FingerPrintMatchingStrategy implements MatchingStrategy {
@SuppressWarnings("unchecked")
PARTIAL(MatchingStrategyType.PARTIAL, (Object reqInfo, Object entityInfo, Map props) -> {
if (reqInfo instanceof Map && entityInfo instanceof Map) {
Object object = props.get(IdaIdMapping.FINGERPRINT.getIdname());
if (object instanceof TriFunctionWithBusinessException) {
TriFunctionWithBusinessException
© 2015 - 2025 Weber Informatics LLC | Privacy Policy